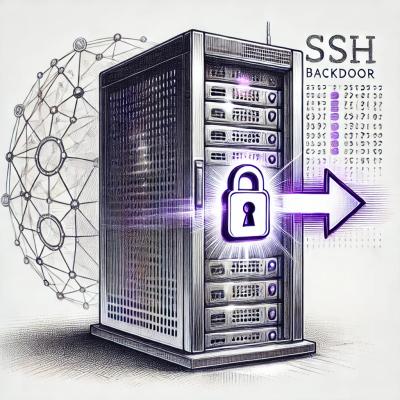
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@or-sdk/qna
Advanced tools
The `@or-sdk/qna` package provides a client for working with the QnA (Question and Answer) system in OneReach.ai. With this client, you can perform operations such as loading documents, creating collections, searching in collections, and asking questions
The @or-sdk/qna
package provides a client for working with the QnA (Question and Answer) system in OneReach.ai. With this client, you can perform operations such as loading documents, creating collections, searching in collections, and asking questions to generate answers based on the loaded documents.
A collection is a group of searchable items, also known as passages. It allows you to organize and manage related documents and their passages together under a single entity. When you perform a search or ask a question, the QnA system will search through the passages within the specified collection.
Documents are used to load a bulk of data into a collection. They can be any type of text-based file such as PDFs, text files, or web pages. When you provide a URL for a document, the QnA system downloads the content, splits it into smaller chunks (passages), and adds them to the collection.
A passage is an atomic piece of data that represents a portion of a document. It is the smallest searchable unit within a collection. When you search or ask a question, the QnA system uses vector similarity to find the most relevant passages from all the documents in the collection.
The search process involves comparing the vector representation of the input query (search term or question) with the vector representations of the passages in the collection. The results are ranked based on the similarity between the query and passages, with the most similar passages being returned as the search results.
To install the package, run the following command:
$ npm install @or-sdk/qna
To use the QnA
client, you need to create an instance of the class with the appropriate configuration options. You can either provide the direct API URL or the service discovery URL. Here is an example:
import { QnA } from '@or-sdk/qna';
// with direct api url
const qna = new QnA({
token: 'my-account-token-string',
serviceUrl: 'http://example.qna/endpoint'
});
// with service discovery(slower)
const qna = new QnA({
token: 'my-account-token-string',
discoveryUrl: 'http://example.qna/endpoint'
});
Once you have an instance of the QnA
client, you can use its methods to interact with the OneReach.ai QnA system. The available methods are:
loadDocument
Load a document into a collection.
const collectionId = 'a1b2c3d4-uuid';
const document = await qna.loadDocument(collectionId, {
description: 'A sample document',
url: 'http://example.com/sample-document.pdf',
name: 'Sample Document',
});
// Example response
{
id: 'e5f6g7h8-uuid',
accountId: 'i9j0k1l2-uuid',
collection: 'a1b2c3d4-uuid',
name: 'Sample Document',
description: 'A sample document'
}
createCollection
Create a new collection.
const collection = await qna.createCollection({
description: 'A sample collection',
name: 'Sample Collection',
});
// Example response
{
id: 'a1b2c3d4-uuid',
accountId: 'i9j0k1l2-uuid',
name: 'Sample Collection',
description: 'A sample collection'
}
deleteCollection
Delete a collection by its ID.
const collectionId = 'a1b2c3d4-uuid';
await qna.deleteCollection(collectionId);
search
Search for documents in a collection.
const collectionId = 'a1b2c3d4-uuid';
const searchResults = await qna.search(collectionId, {
term: 'search term',
limit: 10,
});
// Example response
[
{
id: 'e5f6g7h8-uuid',
distance: '0.1234',
content: 'Found passage content',
metadata: {}
}
]
ask
Ask a question and generate an answer based on the documents in a collection.
const collectionId = 'a1b2c3d4-uuid';
const askResults = await qna.ask(collectionId, {
question: 'What is the meaning of life?',
messages: [
{ role: 'user', content: 'Hello' },
{ role: 'assistant', content: 'Hi there!' },
],
limit: 10,
});
// Example response
{
result: { role: 'assistant', content: 'The meaning of life is 42.' },
searchResult: {
id: 'e5f6g7h8-uuid',
distance: '0.1234',
content: 'Found passage content',
metadata: {}
}
}
updateDocument
Update a document's description.
const collectionId = 'a1b2c3d4-uuid';
const documentId = 'e5f6g7h8-uuid';
const updatedDocument = await qna.updateDocument(collectionId, documentId, {
description: 'Updated document description',
});
// Example response
{
id: 'e5f6g7h8-uuid',
accountId: 'i9j0k1l2-uuid',
collection: 'a1b2c3d4-uuid',
name: 'Sample Document',
description: 'Updated document description'
}
updateCollection
Update a collection's description.
const collectionId = 'a1b2c3d4-uuid';
const updatedCollection = await qna.updateCollection(collectionId, {
description: 'Updated collection description',
});
// Example response
{
id: 'a1b2c3d4-uuid',
accountId: 'i9j0k1l2-uuid',
name: 'Sample Collection',
description: 'Updated collection description'
}
getDocument
Get a document by its ID.
const collectionId = 'a1b2c3d4-uuid';
const documentId = 'e5f6g7h8-uuid';
const document = await qna.getDocument(collectionId, documentId);
// Example response
{
id: 'e5f6g7h8-uuid',
accountId: 'i9j0k1l2-uuid',
collection: 'a1b2c3d4-uuid',
name: 'Sample Document',
description: 'A sample document'
}
getCollection
Get a collection by its ID.
const collectionId = 'a1b2c3d4-uuid';
const collection = await qna.getCollection(collectionId);
// Example response
{
id: 'a1b2c3d4-uuid',
accountId: 'i9j0k1l2-uuid',
name: 'Sample Collection',
description: 'A sample collection'
}
listDocuments
List documents in a collection with optional pagination and query.
const collectionId = 'a1b2c3d4-uuid';
const documents = await qna.listDocuments(collectionId, {
query: 'search query',
size: 10,
skip: 0,
});
// Example response
```json
{
"items": [
{
"id": "e5f6g7h8-uuid",
"accountId": "i9j0k1l2-uuid",
"collection": "a1b2c3d4-uuid",
"name": "Sample Document",
"description": "A sample document"
}
],
"total": 1
}
listCollections
List collections with optional pagination and query.
const collections = await qna.listCollections({
query: 'search query',
size: 10,
skip: 0,
});
// Example response
{
"items": [
{
"id": "a1b2c3d4-uuid",
"accountId": "i9j0k1l2-uuid",
"name": "Sample Collection",
"description": "A sample collection"
}
],
"total": 1
}
deleteDocument
Delete a document from a collection.
const collectionId = 'a1b2c3d4-uuid';
const documentId = 'e5f6g7h8-uuid';
await qna.deleteDocument(collectionId, documentId);
FAQs
The `@or-sdk/qna` package provides a client for working with the QnA (Question and Answer) system in OneReach.ai a.k.a Lookup. With this client, you can perform operations such as loading documents, creating collections, searching in collections, asking q
The npm package @or-sdk/qna receives a total of 379 weekly downloads. As such, @or-sdk/qna popularity was classified as not popular.
We found that @or-sdk/qna demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.