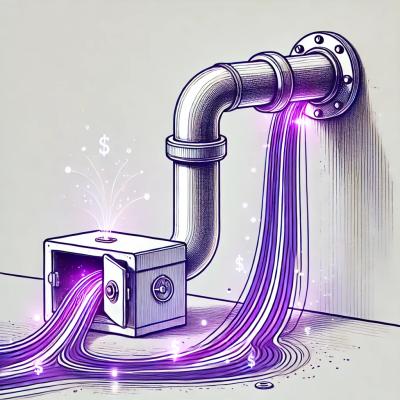
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@orbis-systems/omni-sdk-ts
Advanced tools
Omni SDK is designed to help clients consume Orbis APIs with ease. Omni SDK takes care of all the heavy lifting and provides out of the box ready to use objects.
Omni SDK is designed to help clients consume Orbis APIs with ease. Omni SDK takes care of all the heavy lifting and provides out of the box ready to use objects.
npm install @orbis-systems/omni-sdk-ts
import { Orbis, Accounts, Avatar, Passport, Logos } from '@orbis-systems/omni-sdk-ts';
const orbis = Orbis.connect({ api_url: '', token_id: 'orbis_token' });
const accounts = Accounts.connect( { api_url: '', token_id: 'accounts_id' });
const passport = Passport.connect( { api_key: 'key', api_secret: 'secret'});
const logos = Logos.connect({ api_key: 'key', api_secret: 'secret' });
const avatar = Avatar.connect({ api_url: '' });
const sdk = { orbis, passport, accounts, logos };
const login = await sdk.orbis.client.login('username', 'password');
const logout = await sdk.orbis.client.logout();
Note
api_url
contact orbis to for correct environment configuration.
interface IOrbisConnect {
api_url: string
token_id: string
auto_renew_token?: boolean
error_handling?: boolean
onUnauthorized?: () => void
request_timeout?: number
}
interface IAccountsConnect {
api_url: string
token_id: string
auto_renew_token?: boolean
error_handling?: boolean
onUnauthorized?: () => void
request_timeout?: number
}
interface IPassportConnect {
api_key: string
api_secret: string
error_handling?: boolean
onUnauthorized?: () => void
request_timeout?: number
}
interface ILogosConnect {
api_key: string
api_secret: string
error_handling?: boolean
onUnauthorized?: () => void
request_timeout?: number
}
interface IAvatarConnect {
api_url: string
error_handling?: boolean
onUnauthorized?: () => void
request_timeout?: number
}
interface ICustomStorage {
setItem: (key: string, value: string) => Promise<void> | void
getItem: (key: string) => Promise<string | undefined> | string | undefined
removeItem: (key: string) => Promise<void> | void
}
config
api_url: string
required
token_id: string
required
auto_renew_token: boolean
optional
Default: true
error_handling: 'boolean
optional
Default: true
false
Accounts will NOT automatically catch and handle errors but instead throw the error which needs to be caught by the client, see error handling section.onUnauthorized: Function
optional
request_timeout: number
optional
storage
storage: 'session' | 'local' | 'node' | ICustomStorage
required
Default: session
for web clients and node
for server clients.
Describes the type of storage that will be used to store authorization token. Custom controller can be provided.
ICustomStorage: Object
Provides storage functions for authentication that Accounts needs to use to get, set or delete an API token.
setItem: Function
required
getItem: Function
required
removeItem: Function
required
config
api_url: string
required
token_id: string
required
auto_renew_token: boolean
optional
Default: true
error_handling: 'boolean
optional
Default: true
false
Orbis will NOT automatically catch and handle errors but instead throw the error which needs to be caught by the client, see error handling section.onUnauthorized: Function
optional
request_timeout: number
optional
storage
storage: 'session' | 'local' | 'node' | ICustomStorage
required
Default: session
for web clients and node
for server clients.
Describes the type of storage that will be used to store authorization token. Custom controller can be provided.
ICustomStorage: Object
Provides storage functions for authentication that Orbis needs to use to get, set or delete an API token.
setItem: Function
required
getItem: Function
required
removeItem: Function
required
config
api_key: string
required
api_secret: string
required
error_handling: 'boolean
optional
Default: true
false
Passport will NOT automatically catch and handle errors but instead throw the error which needs to be caught by the client, see error handling section.onUnauthorized: Function
optional
request_timeout: number
optional
config
api_key: string
required
api_secret: string
required
error_handling: 'boolean
optional
Default: true
false
Logos will NOT automatically catch and handle errors but instead throw the error which needs to be caught by the client, see error handling section.onUnauthorized: Function
optional
request_timeout: number
optional
config
api_url: string
required
error_handling: 'boolean
optional
Default: true
false
Avatar will NOT automatically catch and handle errors but instead throw the error which needs to be caught by the client, see error handling section.onUnauthorized: Function
optional
request_timeout: number
optional
Orbis
Accounts
Logos
Passport
Avatar
Omni SDK provides a client
object that is used to authenticate with each API. On a successful authentication Omni SDK calls setItem(token_id, token)
function provided in storage
or the default controller. All endpoints that are protected must be used after the client has authenticated, otherwise the onUnauthorized()
function with be called. client
object has the following methods:
login(username: string, password: string)
const login = await sdk.orbis.client.login('username', 'password');
logout()
const logout = await sdk.orbis.client.logout();
updatePassword(currentPassword: string, newPassword: string)
const update = await sdk.orbis.client.updatePassword('password', 'newPassword');
login(email: string, password: string, includes?: Array<string>)
const login = await sdk.accounts.client.login('email', 'password', ['user.role']);
updateToken(token: string, auto_new: boolean)
sdk.accounts.client.updateToken('token', true);
logout()
const logout = await sdk.accounts.client.logout();
api_key
and api_secret
to each configuration object for logos
and passport
Orbis API
and Accounts API
token for authenticationOmni SDK allows four different storage types local
, session
, node
and custom
. When custom is used the ICustomStorage
object is required which supports synchronous and asynchronous methods. The following is an asynchronous:
import { Orbis } from '@orbis-systems/omni-sdk-ts';
//Default storage: `session` for web and `node` for server
Orbis.storage.use({
//With a callback
setItem: async (key, value): Promise<void> => {
return new Promise((resolve, reject) => {
db.set(key, value, (err) => {
if(err){
reject('set_item'); //Will be caught and passed through to `on_controller_error`
}else{
resolve();
}
});
});
},
//With async/await
getItem: async (key): Promise<string | undefined> => {
return await db.get(key); //If an error is thrown, it will be caught and passed through to `on_controller_error`
}
});
orbis.dev
for the corresponding API documentation.Besides authentication all endpoints have the same structure.
To call an endpoint for example; use the sdk.orbis
object, access the method object and then add the endpoint name.
//POST {{domain}}/api/user/info
sdk.orbis.post('user/info');
//GET {{domain}}/api/quotes/equity
sdk.orbis.get('quotes/equity');
endpoint(url: Url, data?: any, config?: IEndpointRequest) => Promise<IClientResponse>
data
optional
is anything that is required by the corresponding API.sdk.accounts.post('users/get', {
user_id: 1 //Or anything else required for given endpoint
});
config
optional
can be used to override defaults{
urlQuery?: string | number | Array<string | number> //Default: ''
params?: any //Default {}
headers?: { key: string]: string } //Default { 'Authorization': `Bearer ${token}`, 'Content-Type': 'application/json' }
responseType?: 'arraybuffer' | 'document' | 'json' | 'text' | 'stream' | 'blob' //Default json
timeout?: number //Specifies after hold long should the request timeout
}
Note
config
parameter is used ONLY for overwriting the default values if needed.
Omni SDK automatically catches and handles errors unless the error_handling
is set to false. The following is an example of custom error handling:
import { Orbis } from '@orbis-systems/omni-sdk-ts';
const orbis = Orbis.connect({ api_url: '', token_id: 'orbis_token', error_handling: false });
try{
const login = await sdk.orbis.client.login('username', 'password');
//Proceed with login.data
}catch(err){
//Handle error
}
interface IClientResponse {
data: any,
status: number | null,
success: boolean,
error: AxiosError | null
}
FAQs
Omni SDK is designed to help clients consume Orbis APIs with ease. Omni SDK takes care of all the heavy lifting and provides out of the box ready to use objects.
We found that @orbis-systems/omni-sdk-ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.