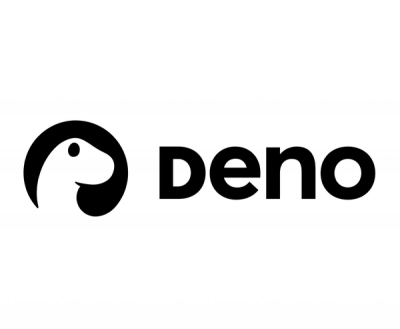
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@outlinerisk/auth0-tools
Advanced tools
Handles everything Auth0.
npm i -g @outlinerisk/auth0-tools
yarn global add @outlinerisk/auth0-tools
You'll need to set a few values to secure a connection to Auth0's Management API:
Domain // The domain of your Auth0 tenant.
Client ID // The client ID of the Management API M2M app.
Client Secret // The client secret of the Management API M2M app.
Name Prefix // An optional prefix for the names of all your Auth0 resources.
You can pass these values in as optional arguments...
auth0-tools api deploy api_name api_audience --domain=domain --client-id=client_id --client-secret=client_secret
...but this can be tedious. Without these optional arguments, the CLI will default to the following environment variables:
process.env.AUTH0_DOMAIN
process.env.AUTH0_MANAGEMENT_CLIENT_ID
process.env.AUTH0_MANAGEMENT_CLIENT_SECRET
process.env.AUTH0_NAME_PREFIX
To deploy actions, you'll need to provide JSON files that describes each action's properties.
# sample `action.json`
{
"code": "\nexports.onExecuteCredentialsExchange = async (event, api) => {\n const greeting = 'Hello World!'\n}",
"name": "My Action",
"runtime": "node16",
"supported_triggers": [
{
"id": "post-login",
"version": "v2"
}
]
}
To do this, create a .auth0-toolrc
configuration file. You must keep this file in your project root directory.
In the config file, create a actionsDir
variable and set it to the path of your actions directory, relative to the project's root directory.
# project
# ├---actions
# | | action1.json
# | | action2.json
# | ├---action3
# | | action3.json
# | └---action4
# | action4.json
# ├---folder1
# ...
# sample `.auth0-toolsrc`
actionsDir='./actions'
The CLI will recursively look within the actionsDir
for all JSON files. Note that if you have any non-action JSON files in the actionsDir
, the CLI will break.
It can be messy keeping code as a string. Instead of manually creating action.json
files, we recommend creating Action
objects, parsing them using a tool such as JSON.stringify()
, then writing the JSON string to a JSON file.
// create the action as an Action object
const action: Action = {
code: `
exports.onExecuteCredentialsExchange = async (event, api) => {
const greeting = 'Hello World!'
}`,
name: 'My Action',
runtime: 'node16',
supported_triggers: [
{
id: 'credentials-exchange',
version: 'v2',
},
],
}
// parse the action as a JSON string
const actionJSONString = JSON.stringify(action)
// write the JSON string to a JSON file
import fs from 'fs'
fs.writeFile('./action/action1/action1.json', actionJSONString, (err) => {
if (err) throw err
})
import { APIClient } from '@outlinerisk/auth0-tools'
const domain = process.env.AUTH0_DOMAIN
const clientId = process.env.AUTH0_MANAGEMENT_CLIENT_ID
const clientSecret = process.env.AUTH0_MANAGEMENT_CLIENT_SECRET
const apiClient = new APIClient(domain, clientId, clientSecret)
const apiName = 'My API'
const apiAudience = 'https://my-website.com/my/api'
await apiClient.deployAPI(apiName, apiAudience)
auth0-tools help
Usage: main [options] [command]
Pathpoint's CLI tool for managing Auth0 resources.
Options:
-V, --version output the version number
-h, --help display help for command
Commands:
action Run action commands.
api Run API commands.
app Run app commands.
help [command] display help for command
FAQs
Pathpoint's internal Auth0 tooling.
The npm package @outlinerisk/auth0-tools receives a total of 257 weekly downloads. As such, @outlinerisk/auth0-tools popularity was classified as not popular.
We found that @outlinerisk/auth0-tools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.