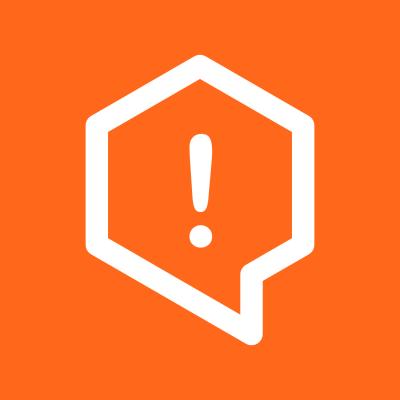
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@paciolan/remote-module-loader
Advanced tools
Loads a CommonJS module from a remote url.
Lazy Load Modules to keep initial load times down and load modules just in time, similar to Webpack's code splitting.
Update Remote Modules independent of the web application. Update a module without redeploying the web application.
npm install @paciolan/remote-module-loader
The createLoadRemoteModule
function is used to inject dependencies into a loadRemoteModule
function.
It is recommended to create a separate file, in this example it is called src/lib/loadRemoteModule.js
.
If your module has no external dependencies, this is the easiest method to fetch the remote module.
/**
* src/lib/loadRemoteModule.js
*/
import { createLoadRemoteModule } from "@paciolan/remote-module-loader";
export default createLoadRemoteModule();
You can pass dependencies to the module. All modules loaded with this version of loadRemoteModule
, will have the dependencies available to require
.
/**
* src/lib/loadRemoteModule.js
*/
import {
createLoadRemoteModule,
createRequires
} from "@paciolan/remote-module-loader";
const dependencies = {
react: require("react")
};
const requires = createRequires(dependencies);
export default createLoadRemoteModule({ requires });
By default loadRemoteModule
will use the XMLHttpRequest
object avaiable in the browser. This can be overridden if you want to use an alternate method.
/**
* src/lib/loadRemoteModule.js
*/
import { createLoadRemoteModule } from "@paciolan/remote-module-loader";
import axios from "axios";
const fetcher = url => axios.get(url).then(request => request.data);
export default createLoadRemoteModule({ fetcher });
Modules are loaded asynchronously, so use similar techniques to any other async function.
/**
* src/index.js
*/
import loadRemoteModule from "./lib/loadRemoteModule";
const myModule = loadRemoteModule("http://fake.url/modules/my-module.js");
myModule.then(m => {
const value = m.default();
console.log({ value });
});
/**
* src/index.js
*/
import loadRemoteModule from "./lib/loadRemoteModule";
const main = async () => {
const myModule = await loadRemoteModule(
"http://fake.url/modules/my-module.js"
);
const value = myModule.default();
console.log({ value });
};
main();
Remote Modules must be in the CommonJS format, using exports
to export functionality.
This is an example of a simple CommonJS module:
var name = "myModule";
function helloWorld() {
console.log("Hello World!");
}
exports.name = name;
exports.default = helloWorld;
note: overwriting exports
will cause failures.
// ❌ NO!
exports = {
default: "FAIL!"
};
// ✅ YES!
exports.default = "SUCCESS!";
Setting up Webpack to export a CommonJS is pretty easy.
Inside webpack.config.js
, set the libraryTarget
to "commonjs"
.
module.exports = {
output: {
libraryTarget: "commonjs"
}
};
Dependencies that will be provided by the Web Application that uses your Remote Module can be added to webpack's externals
section.
This will prevent webpack from bundling unwanted 3rd party libraries, decreasing the bundle size.
module.exports = {
output: {
libraryTarget: "commonjs"
},
externals: {
react: "react",
"prop-types": "prop-types"
}
};
Joel Thoms (https://twitter.com/joelnet)
Icon made by Freepik from www.flaticon.com
FAQs
Loads a CommonJS module from a remote URL for the Browser or Node.js.
The npm package @paciolan/remote-module-loader receives a total of 5,845 weekly downloads. As such, @paciolan/remote-module-loader popularity was classified as popular.
We found that @paciolan/remote-module-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.