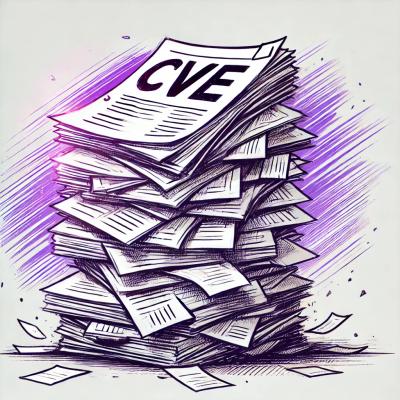
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@pawsteam/ts-jsonrpc-server
Advanced tools
A framework for easily building JSONRPC simple servers in TS
For MacOS Catalina, please follow this link
All the rest, npm install
.
The framework is aimed at making life easier when writing JSON-RPC servers. It is Promise-based, so everything will return a promise, except for validators. It is revolving around stages/hooks in the life of a request:
Input validators
They check the request for required data and types. It's a place to define what parameters are needed for a method to be called.
Any number of Input Validators can be assigned for one method. Generic ones as well;
@AppConfigDecorator({
port: 2000,
services: [SomeService],
genericValidators: [
new HeaderExistsValidator('content-type'),
new HeaderValueContainsValidator('user-agent', 'Mozilla/5.0')
]
})
export class AppMain {
...
}
export class HeaderExistsValidator implements ValidatorInterface {
headerName: string;
constructor(headerName: string) {
this.headerName = headerName;
}
validate(params: any, request): boolean {
...
}
}
The input validators implement ValidatorInterface and return a boolean value.
Input transformers - they transform the request data into objects that are passed to the methods Any number of input transformer can exist per method.
It must return a promise of one parameter.
The order of validators will give the order of parameters in the method call.
The transformers implement the TransformerInterface and return a Promise to the type of object they are for.
export class BucketTransformer implements TransformerInterface {
/**
* The ParsedRpcObjectType is an object created from the body of the request, parsed,
* and put into the form of a JSONRPC object:
* {
* id: <id>,
* method: <methodname>,
* jsonrpc: '2.0',
* params: {...}
* }
*/
transform(request: IncomingMessage, jsonrpcObj: ParsedRpcObjectType): Promise<BucketType> {
const newBucket = new BucketType(jsonrpcObj.params.path);
return Promise.resolve(newBucket);
}
}
The reason this method needs to return a promise is because we might need to fetch additional data in order to create the object. For instance, we might need to query a DB.
Validation can also be done in the transformer. If the transformer rejects the promise or throws, the transformation is interpreted as failed, so validation failed.
To use a transformer, you simply instantiate a new instance of your transformer:
@TransformerDecorator(new CarTransformer())
@TransformerDecorator(new EngineTransformer())
method3(car: CarType, engine: EngineType, request: IncomingMessage, response: ServerResponse) {
return Promise.resolve(car.wheels * engine.power);
}
The method itself - this is where the app logic lives
It must also return a promise of a HttpResponse type of object, this object will be returned to the user.
@ValidatorDecorator(new BucketValidator())
@TransformerDecorator(new BucketTransformer())
createBucket(bucket: BucketType, context: RequestContextType): Promise<any> {
return Promise.resolve({created: bucket.path});
}
TODO: Response serializer - this is where you can decide what exactly ends up in the response.
In the near future, a functionality to make the server emit events will be implemented.
FAQs
A framework for easily building JSONRPC simple servers in TS
The npm package @pawsteam/ts-jsonrpc-server receives a total of 14 weekly downloads. As such, @pawsteam/ts-jsonrpc-server popularity was classified as not popular.
We found that @pawsteam/ts-jsonrpc-server demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.