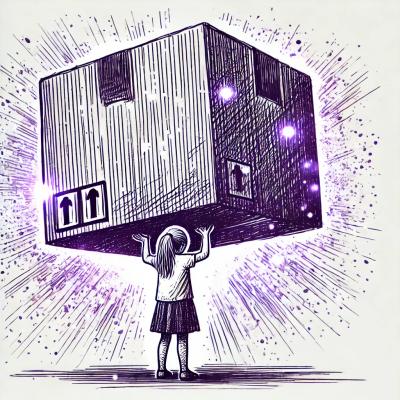
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@petamoriken/float16
Advanced tools
@petamoriken/float16 is an npm package that provides utilities for handling 16-bit floating-point numbers (half-precision). It allows for conversion between 16-bit floats and other numeric types, as well as arithmetic operations on 16-bit floats.
Conversion from 32-bit float to 16-bit float
This feature allows you to convert a 32-bit floating-point number to a 16-bit floating-point number. The code sample demonstrates converting the 32-bit float value 1.5 to its 16-bit float representation.
const { float32ToFloat16 } = require('@petamoriken/float16');
const float32 = 1.5;
const float16 = float32ToFloat16(float32);
console.log(float16); // Output: 15360
Conversion from 16-bit float to 32-bit float
This feature allows you to convert a 16-bit floating-point number back to a 32-bit floating-point number. The code sample demonstrates converting the 16-bit float value 15360 back to its 32-bit float representation.
const { float16ToFloat32 } = require('@petamoriken/float16');
const float16 = 15360;
const float32 = float16ToFloat32(float16);
console.log(float32); // Output: 1.5
Arithmetic operations on 16-bit floats
This feature provides basic arithmetic operations (addition, subtraction, multiplication, and division) on 16-bit floating-point numbers. The code sample demonstrates these operations using two 16-bit float values representing 1.5.
const { addFloat16, subFloat16, mulFloat16, divFloat16 } = require('@petamoriken/float16');
const a = 15360; // 1.5 in 16-bit float
const b = 15360; // 1.5 in 16-bit float
console.log(addFloat16(a, b)); // Output: 16384 (3.0 in 16-bit float)
console.log(subFloat16(a, b)); // Output: 0 (0.0 in 16-bit float)
console.log(mulFloat16(a, b)); // Output: 15872 (2.25 in 16-bit float)
console.log(divFloat16(a, b)); // Output: 15360 (1.0 in 16-bit float)
The 'float16' package provides similar functionality for handling 16-bit floating-point numbers. It includes methods for converting between 16-bit and 32-bit floats, as well as arithmetic operations. However, it may have a different API and performance characteristics compared to @petamoriken/float16.
half precision floating point for JavaScript
see ES Discuss Float16Array topic
This library's Float16Array
uses Proxy
object, so IE11 is never supported.
lib/
and browser/
directories in the npm package have JavaScript files already built, whose target are
When you build by yourself using webpack or rollup.js for old browsers support, please transpile JavaScript files in src/
directory.
yarn add @petamoriken/float16
or
npm install @petamoriken/float16 --save
// ES Modules
import { Float16Array, getFloat16, setFloat16, hfround } from "@petamoriken/float16";
or
// CommonJS
const { Float16Array, getFloat16, setFloat16, hfround } = require("@petamoriken/float16");
Copy browser/float16.js
file to your project directory.
<script src="DEST/TO/float16.js"></script>
<script>
const { Float16Array, getFloat16, setFloat16, hfround } = float16;
</script>
Float16Array
This API is similar to TypedArray
such as Float32Array
.
const float16 = new Float16Array([1.0, 1.1, 1.2]);
for(const val of float16) {
console.log(val); // => 1, 1.099609375, 1.19921875
}
float16.reduce((prev, current) => prev + current); // 3.298828125
getFloat16(view: DataView, byteOffset: number, littleEndian?: boolean): number
setFloat16(view: DataView, byteOffset: number, value: number, littleEndian?: boolean): void
These APIs are similar to DataView
methods such as DataView#getFloat32
and DataView#setFloat32
.
const buffer = new ArrayBuffer(10);
const view = new DataView(buffer);
view.setUint16(0, 0x1234);
getFloat16(view, 0); // 0.0007572174072265625
// You can append methods to DataView instance
view.getFloat16 = (...args) => getFloat16(view, ...args);
view.setFloat16 = (...args) => setFloat16(view, ...args);
view.getFloat16(0); // 0.0007572174072265625
view.setFloat16(0, Math.PI, true);
view.getFloat16(0, true); // 3.140625
hfround(x: number): number
This API is similar to Math.fround
(MDN).
This function returns nearest half precision float representation of a number.
Math.fround(1.337); // 1.3370000123977661
hfround(1.337); // 1.3369140625
First, download devDependencies.
yarn
Build lib/
, browser/
files.
yarn run build
Build docs/
files (for browser test).
yarn run docs
First, download devDependencies.
yarn
yarn test
Access test page (power-assert version).
FAQs
IEEE 754 half-precision floating-point for JavaScript
The npm package @petamoriken/float16 receives a total of 224,070 weekly downloads. As such, @petamoriken/float16 popularity was classified as popular.
We found that @petamoriken/float16 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.