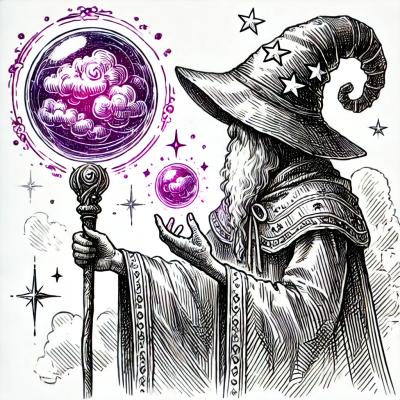
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@pixi/math
Advanced tools
@pixi/math is a utility library for mathematical operations and geometric transformations, primarily used in conjunction with the PixiJS rendering library. It provides a set of classes and functions to handle common mathematical tasks such as vector operations, matrix transformations, and geometric calculations.
Vector Operations
The @pixi/math package provides a Point class for 2D vector operations. You can create points, add them, and perform other vector operations.
const { Point } = require('@pixi/math');
const point1 = new Point(10, 20);
const point2 = new Point(30, 40);
const result = point1.add(point2);
console.log(result); // Point { x: 40, y: 60 }
Matrix Transformations
The Matrix class allows for complex 2D transformations including translation, scaling, and rotation. This is useful for manipulating objects in a 2D space.
const { Matrix } = require('@pixi/math');
const matrix = new Matrix();
matrix.translate(10, 20);
matrix.scale(2, 2);
console.log(matrix); // Matrix { a: 2, b: 0, c: 0, d: 2, tx: 10, ty: 20 }
Rectangle and Circle Geometry
The package includes classes for geometric shapes like Rectangle and Circle, which provide methods to check for containment and intersection.
const { Rectangle, Circle } = require('@pixi/math');
const rect = new Rectangle(0, 0, 100, 100);
const circle = new Circle(50, 50, 25);
console.log(rect.contains(50, 50)); // true
console.log(circle.contains(50, 50)); // true
gl-matrix is a high-performance matrix and vector library for WebGL. It provides similar functionalities for vector and matrix operations but is more focused on 3D transformations and is widely used in WebGL applications.
mathjs is a comprehensive math library for JavaScript and Node.js. It offers a wide range of mathematical functions and supports complex numbers, matrices, and units. While it is more general-purpose, it can be used for similar vector and matrix operations.
Three.js is a popular 3D library that includes its own set of mathematical utilities for 3D transformations, including vectors, matrices, and quaternions. It is more focused on 3D rendering but provides similar mathematical functionalities.
npm install @pixi/math
import * as math from '@pixi/math';
FAQs
Collection of math utilities
The npm package @pixi/math receives a total of 113,885 weekly downloads. As such, @pixi/math popularity was classified as popular.
We found that @pixi/math demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.