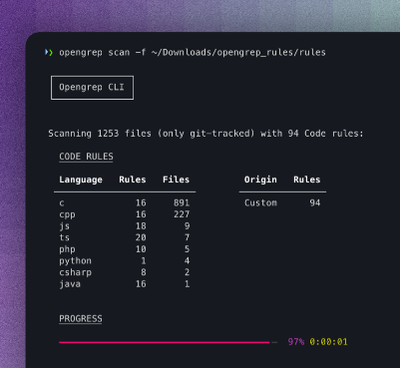
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
@placekit/client-js
Advanced tools
Location data, search and autocomplete for your apps
Features • Quick start • Reference • Examples • License
PlaceKit JavaScript Client abstracts interactions with our API, making your life easier. We highly recommend to use it instead of accessing our API directly.
👉 If you're looking for a full Autocomplete experience, have a look at our standalone PlaceKit Autocomplete JS library, or check out our examples to learn how to integrate with an existing components library.
First, install PlaceKit JavaScript Client using npm package manager:
npm install --save @placekit/client-js
Then import the package and perform your first address search:
// CommonJS syntax:
const placekit = require('@placekit/client-js');
// ES6 Modules syntax:
import placekit from '@placekit/client-js';
const pk = placekit('<your-api-key>', {
countries: ['fr'],
//...
});
pk.search('Paris').then((res) => {
console.log(res.results);
});
👉 For advanced usages, check out our examples.
First, add this line before the closing </body>
tag in your HTML to import PlaceKit JavaScript Client:
<script src="https://cdn.jsdelivr.net/npm/@placekit/client-js@1.1.0/dist/placekit.umd.js"></script>
Then it works the same as the node example above.
After importing the library, placekit
becomes available as a global:
<script>
const pk = placekit('<your-api-key>', {
countries: ['fr'],
//...
});
pk.search('Paris').then((res) => {
console.log(res.results);
});
</script>
Or if you are using native ES Modules:
<script type="module">
import placekit from 'https://cdn.jsdelivr.net/npm/@placekit/client-js@1.1.0/dist/placekit.esm.mjs';
const pk = placekit(/* ... */);
// ...
</script>
👉 For advanced usages, check out our examples.
placekit()
pk.search()
pk.reverse()
pk.options
pk.configure()
pk.requestGeolocation()
pk.clearGeolocation()
pk.hasGeolocation
placekit()
PlaceKit initialization function returns a PlaceKit client, named pk
in all examples.
const pk = placekit('<your-api-key>', {
countries: ['fr'],
language: 'en',
maxResults: 10,
});
Parameter | Type | Description |
---|---|---|
apiKey | string | API key |
options | key-value mapping (optional) | Global parameters (see options) |
pk.search()
Performs a search and returns a Promise, which response is a list of results alongside some request metadata. The options passed as second parameter override the global parameters only for the current query.
pk.search('Paris', {
countries: ['fr'],
maxResults: 5,
}).then((res) => {
console.log(res.results);
});
Parameter | Type | Description |
---|---|---|
query | string | Search terms |
opts | key-value mapping (optional) | Search-specific parameters (see options) |
pk.reverse()
Performs a reverse geocoding search and returns a Promise, which response is a list of results alongside some request metadata.
The options passed as first parameter override the global parameters only for the current query.
Any coordinates
previously set as option would be overriden by the coordinates passed as first argument.
pk.reverse({
coordinates: '48.871086,2.3036339',
countries: ['fr'],
maxResults: 5,
}).then((res) => {
console.log(res.results);
});
Parameter | Type | Description |
---|---|---|
opts | key-value mapping (optional) | Search-specific parameters (see options) |
Notes:
options.coordinates
, it'll use coordinates
from global parameters set when instanciating with placekit()
or with pk.configure()
.pk.reverse()
, then it'll use the user's IP approximate coordinates but relevance will be less accurate.pk.reverse()
, the API automatically sets countryByIP
to true
. Explicitely set it to false
to turn it off.pk.reverse()
is the same as calling pk.search
with an empty query and countryByIP: true
:pk.reverse({
countries: ['fr'],
});
// is the same as:
pk.search('', {
countryByIP: true,
countries: ['fr'],
});
pk.options
Read-only to access global options persistent across all API calls that are set at initialization and with pk.configure()
.
Options passed at query time in pk.search()
override global parameters only for that specific query.
console.log(pk.options); // { "language": "en", "maxResults": 10, ... }
Option | Type | Default | Description |
---|---|---|---|
maxResults | integer? | 5 | Number of results per page. |
language | string? | undefined | Preferred language for the results(1), two-letter ISO language code. Supported languages are en and fr . By default the results are displayed in their country's language. |
types | string[]? | undefined | Type of results to show. Array of accepted values: street , city , country , airport , bus , train , townhall , tourism . Prepend - to omit a type like ['-bus'] . Unset to return all. |
countries | string[]? | undefined | Countries to search in, or fallback to if countryByIP is true . Array of two-letter ISO country codes(1). |
countryByIP | boolean? | undefined | Use IP to find user's country (turned off). |
forwardIP | string? | undefined | Set x-forwarded-for header to forward the provided IP for back-end usages (otherwise it'll use the server IP). |
coordinates | string? | undefined | Coordinates to search around. Automatically set when calling pk.requestGeolocation() . |
[1]: See Scope and Limitations for more details.
countries
option is requiredThe countries
option is required at search time, but we like to keep it optional across all methods so developers remain free on when and how to define it:
placekit()
,pk.configure()
,pk.search()
.If countries
is missing or invalid, you'll get a 422
error, excepted whentypes
option is set to ['country']
only.
countryByIP
optionSet countryByIP
to true
when you don't know which country users will search addresses in. In that case, the option countries
will be used as a fallback if the user's country is not supported:
pk.search('123 ave', {
countryByIP: true, // use user's country, based on their IP
countries: ['fr', 'be'], // returning results from France and Belgium if user's country is not supported
});
pk.configure()
Updates global parameters. Returns void
.
pk.configure({
language: 'fr',
maxResults: 5,
countries: ['fr'],
});
Parameter | Type | Description |
---|---|---|
opts | key-value mapping (optional) | Global parameters (see options) |
pk.requestGeolocation()
Requests device's geolocation (browser-only). Returns a Promise with a GeolocationPosition
object.
pk.requestGeolocation({ timeout: 10000 }).then((pos) => console.log(pos.coords));
Parameter | Type | Description |
---|---|---|
opts | key-value mapping (optional) | navigator.geolocation.getCurrentPosition options |
The location will be store in the coordinates
global options, you can still manually override it.
pk.clearGeolocation()
Clear device's geolocation stored with pk.requestGeolocation
.
pk.clearGeolocation();
The global option coordinates
will be deleted and pk.hasGeolocation
will be set to false
.
pk.hasGeolocation
Reads if device geolocation is activated or not (read-only).
console.log(pk.hasGeolocation); // true or false
PlaceKit JavaScript Client is an open-sourced software licensed under the MIT license.
FAQs
PlaceKit JavaScript client
The npm package @placekit/client-js receives a total of 636 weekly downloads. As such, @placekit/client-js popularity was classified as not popular.
We found that @placekit/client-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.