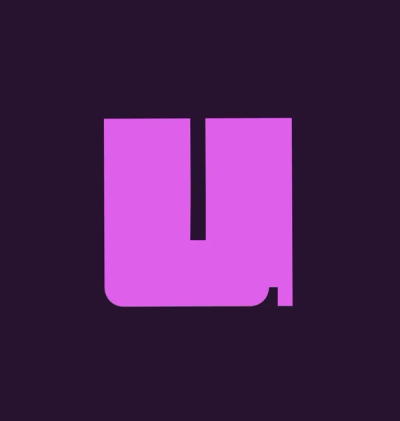
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@polkadot/types
Advanced tools
@polkadot/types is a library for handling types and encoding/decoding of data for the Polkadot and Substrate ecosystem. It provides a comprehensive set of tools for working with the various types used in the Polkadot network, including creating, parsing, and validating data structures.
Creating and Using Custom Types
This feature allows you to create and use custom types. In this example, a custom type 'u32' is created and initialized with the value 12345.
const { TypeRegistry } = require('@polkadot/types');
const registry = new TypeRegistry();
const MyCustomType = registry.createType('u32', 12345);
console.log(MyCustomType.toString()); // '12345'
Encoding and Decoding Data
This feature allows you to encode and decode data. In this example, a 'u32' type is encoded to a Uint8Array and then decoded back to its original value.
const { TypeRegistry } = require('@polkadot/types');
const registry = new TypeRegistry();
const encoded = registry.createType('u32', 12345).toU8a();
const decoded = registry.createType('u32', encoded);
console.log(decoded.toString()); // '12345'
Working with Complex Types
This feature allows you to work with complex types such as vectors and tuples. In this example, a vector of tuples containing 'u32' and 'bool' types is created and logged.
const { TypeRegistry } = require('@polkadot/types');
const registry = new TypeRegistry();
const ComplexType = registry.createType('Vec<(u32, bool)>', [[1, true], [2, false]]);
console.log(ComplexType.toString()); // '[[1,true],[2,false]]'
The ethers.js library is a complete and compact library for interacting with the Ethereum blockchain and its ecosystem. It provides similar functionalities for encoding/decoding data and working with custom types, but it is tailored for the Ethereum network rather than Polkadot.
web3.js is a collection of libraries that allow you to interact with a local or remote Ethereum node using HTTP, IPC, or WebSocket. It provides functionalities for encoding/decoding data and working with custom types, similar to @polkadot/types, but it is specific to the Ethereum blockchain.
bignumber.js is a library for arbitrary-precision decimal and non-decimal arithmetic. While it does not provide the same breadth of functionality as @polkadot/types, it is often used in conjunction with blockchain libraries to handle large numbers and custom types.
Implementation of the types and their (de-)serialisation via SCALE codec. On the Rust side, the codec types and primitive types are implemented via the parity-codec.
FAQs
Implementation of the Parity codec
We found that @polkadot/types demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.