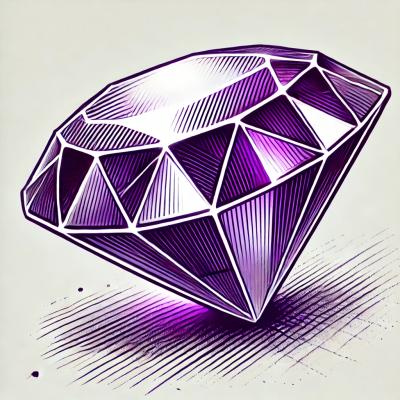
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@poppinss/manager
Advanced tools
This module exposes a class making it easier to implement builder pattern to your classes.
Install the package from npm as follows:
npm i @poppinss/manager
# yarn
yarn add @poppinss/manager
and then import it as follows. Check How it works section for complete docs
import { Manager, ManagerContract } from '@poppinss/manager'
Builder pattern is a way to compose objects by hiding most of their complexities. Similarly, the Manager pattern also manages the lifecycle of those objects, after composing them.
AdonisJs heavily makes use of this pattern in modules like Mail
, Auth
, Session
and so on, where you can switch between multiple drivers, without realizing the amount of work done behind the scenes.
Let's see how the driver's based approach works without the Manager pattern.
class SessionRedisDriver {
public write () {}
public read () {}
}
class SessionFileDriver {
public write () {}
public read () {}
}
class Session {
constructor (private _driver) {
}
put () {
this._driver.put()
}
}
Now, you can use it as follows.
const file = new SessionFileDriver()
const session = new Session(file)
session.put('')
The above example is very simple and may feel fine at first glance. However, you may face following challenges.
Continuing to the same example, let's create a Manager class that abstracts away all of this manual boilerplate.
import { Manager } from '@poppinss/manager'
class SessionManager extends Manager<Session> {
protected getDefaultDriverName () {
return 'file'
}
// Create singleton instances and re-use them
protected $cacheDrivers = true
protected createFile () {
return new Session(new SessionFileDriver())
}
protected createRedis () {
return new Session(new SessionRedisDriver())
}
}
and now, you can use it as follows.
const session = new SessionManager()
session.driver('file') // redis instance
session.driver('redis') // file instance
Also, the session manager can be extended to add more dynamic drivers. Think of accepting plugins to add drivers.
const session = new SessionManager()
session.extend('mongo', () => {
return new SessionMongoDriver()
})
session.driver('mongo') // MongoDriver
Also, you can pass a union to the Manager
constructor and it will typehint the list of drivers for you.
class SessionManager extends Manager<
Session,
{ file: SessionFileDriver, redis: SessionRedisDriver },
> {
}
new SessionManager
.driver('') // typehints 'file' and 'redis'
Along with the runtime code to extend and fetch drivers, we also ship the types to deal with static side of things as well.
Since the process of having extensible drivers with type information requires some complex types structure, we recommend you to look at example/index.ts file for a complete example.
Following are the autogenerated files via Typedoc
`
FAQs
The builder (Manager) pattern implementation
The npm package @poppinss/manager receives a total of 3,199 weekly downloads. As such, @poppinss/manager popularity was classified as popular.
We found that @poppinss/manager demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.