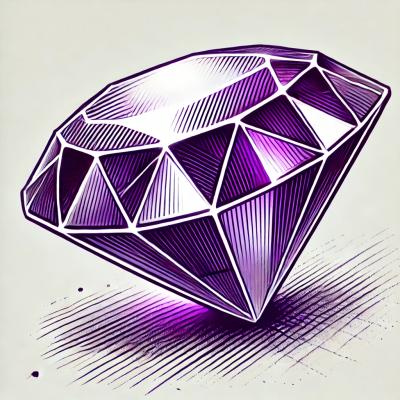
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@poppinss/utils
Advanced tools
@poppinss/utils is a utility library that provides a collection of helper functions for various common tasks in JavaScript and TypeScript. It includes utilities for data manipulation, type checking, and more.
String Manipulation
The string manipulation utilities include functions like camelCase, which converts a string to camel case.
const { string } = require('@poppinss/utils');
const camelCaseString = string.camelCase('hello world');
console.log(camelCaseString); // 'helloWorld'
Object Utilities
Object utilities include functions like clone, which creates a deep copy of an object.
const { object } = require('@poppinss/utils');
const obj = { a: 1, b: 2 };
const clonedObj = object.clone(obj);
console.log(clonedObj); // { a: 1, b: 2 }
Type Checking
Type checking utilities include functions like is.number and is.string, which check the type of a given value.
const { is } = require('@poppinss/utils');
console.log(is.number(123)); // true
console.log(is.string(123)); // false
Lodash is a popular utility library that provides a wide range of functions for data manipulation, type checking, and more. It is more comprehensive and widely used compared to @poppinss/utils.
Underscore is another utility library similar to Lodash, offering a variety of functions for common programming tasks. It is older and less feature-rich than Lodash but still widely used.
Ramda is a functional programming library for JavaScript that provides utility functions with a focus on immutability and pure functions. It offers a different approach compared to @poppinss/utils, emphasizing functional programming paradigms.
Collection of reusable scripts used by AdonisJS core team
This module exports a collection of re-usable utilties to avoid re-writing the same code in every other package.
Install the package from npm registry as follows:
npm i @poppinss/utils
# yarn
yarn add @poppinss/utils
and then use it as follows:
import { requireAll } from '@poppinss/utils'
requireAll(__dirname)
A custom exception class that extends the Error
class to add support for defining status
and error codes
.
import { Exception } from '@poppinss/utils'
throw new Error('Something went wrong', 500, 'E_RUNTIME_EXCEPTION')
throw new Error('Route not found', 404, 'E_ROUTE_NOT_FOUND')
A utility to recursively read all script files for a given directory. This method is equivalent to
readdir + recursive + filter (.js, .json, .ts)
.
import { fsReadAll } from '@poppinss/utils'
const files = fsReadAll(__dirname) // array of strings
Same as fsReadAll
, but instead require the files. Helpful when you want to load all the config files inside a directory on app boot.
import { requireAll } from '@poppinss/utils'
const config = requireAll(join(__dirname, 'config'))
{
file1: {}, // exported object
file2: {} // exported object
}
Utility to require script files wihtout worrying about CommonJs
and ESM
exports. This is how it works.
module.exports
.export default
.export default
.foo.js
module.exports = {
greeting: 'Hello world'
}
foo.default.js
export default {
greeting: 'Hello world'
}
foo.esm.js
export const greeting = {
greeting: 'hello world'
}
import { esmRequire } from '@poppinss/utils'
esmRequire('./foo.js') // { greeting: 'hello world' }
esmRequire('./foo.default.js') // { greeting: 'hello world' }
esmRequire('./foo.esm.js') // { greeting: { greeting: 'hello world' } }
The esmResolver
method works similar to esmRequire
. However, instead of requiring the file, it accepts the object and returns the exported as per the same logic defined above.
import { esmRequire } from '@poppinss/utils'
esmResolver({ greeting: 'hello world' }) // { greeting: 'hello world' }
esmResolver({
default: { greeting: 'hello world' },
__esModule: true,
}) // { greeting: 'hello world' }
esmResolver({
greeting: { greeting: 'hello world' },
__esModule: true,
}) // { greeting: { greeting: 'hello world' } }
Works similar to require.resolve
, however it handles the absolute paths properly.
import { resolveFrom } from '@poppinss/utils'
resolveFrom(__dirname, 'npm-package') // returns path to package "main" file
resolveFrom(__dirname, './foo.js') // returns path to `foo.js` (if exists)
resolveFrom(__dirname, join(__dirname, './foo.js')) // returns path to `foo.js` (if exists)
FAQs
Handy utilities for repetitive work
The npm package @poppinss/utils receives a total of 91,364 weekly downloads. As such, @poppinss/utils popularity was classified as popular.
We found that @poppinss/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.