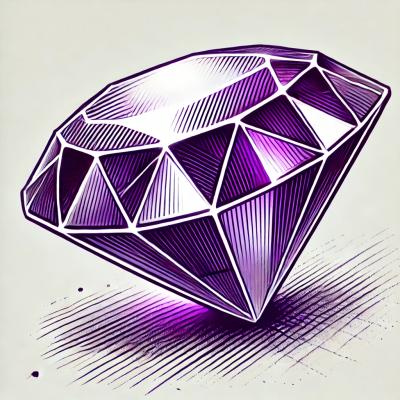
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@poppinss/utils
Advanced tools
@poppinss/utils is a utility library that provides a collection of helper functions for various common tasks in JavaScript and TypeScript. It includes utilities for data manipulation, type checking, and more.
String Manipulation
The string manipulation utilities include functions like camelCase, which converts a string to camel case.
const { string } = require('@poppinss/utils');
const camelCaseString = string.camelCase('hello world');
console.log(camelCaseString); // 'helloWorld'
Object Utilities
Object utilities include functions like clone, which creates a deep copy of an object.
const { object } = require('@poppinss/utils');
const obj = { a: 1, b: 2 };
const clonedObj = object.clone(obj);
console.log(clonedObj); // { a: 1, b: 2 }
Type Checking
Type checking utilities include functions like is.number and is.string, which check the type of a given value.
const { is } = require('@poppinss/utils');
console.log(is.number(123)); // true
console.log(is.string(123)); // false
Lodash is a popular utility library that provides a wide range of functions for data manipulation, type checking, and more. It is more comprehensive and widely used compared to @poppinss/utils.
Underscore is another utility library similar to Lodash, offering a variety of functions for common programming tasks. It is older and less feature-rich than Lodash but still widely used.
Ramda is a functional programming library for JavaScript that provides utility functions with a focus on immutability and pure functions. It offers a different approach compared to @poppinss/utils, emphasizing functional programming paradigms.
Collection of reusable scripts used by AdonisJS core team
This module exports a collection of re-usable utilties to avoid re-writing the same code in every other package. We also include a handful of Lodash utilities, which are used across the AdonisJS packages eco-system.
Install the package from npm registry as follows:
npm i @poppinss/utils
# yarn
yarn add @poppinss/utils
and then use it as follows:
import { requireAll } from '@poppinss/utils'
requireAll(__dirname)
A custom exception class that extends the Error
class to add support for defining status
and error codes
.
import { Exception } from '@poppinss/utils'
throw new Error('Something went wrong', 500, 'E_RUNTIME_EXCEPTION')
throw new Error('Route not found', 404, 'E_ROUTE_NOT_FOUND')
A utility to recursively read all script files for a given directory. This method is equivalent to
readdir + recursive + filter (.js, .json, .ts)
.
import { fsReadAll } from '@poppinss/utils'
const files = fsReadAll(__dirname) // array of strings
You can also define your custom filter function. The filter function must return true
for files to be included.
const files = fsReadAll(__dirname, (file) => {
return file.endsWith('.foo.js')
})
Same as fsReadAll
, but instead require the files. Helpful when you want to load all the config files inside a directory on app boot.
import { requireAll } from '@poppinss/utils'
const config = requireAll(join(__dirname, 'config'))
{
file1: {}, // exported object
file2: {} // exported object
}
Utility to require script files wihtout worrying about CommonJs
and ESM
exports. This is how it works.
module.exports
.export default
.export default
.foo.js
module.exports = {
greeting: 'Hello world'
}
foo.default.js
export default {
greeting: 'Hello world'
}
foo.esm.js
export const greeting = {
greeting: 'hello world'
}
import { esmRequire } from '@poppinss/utils'
esmRequire('./foo.js') // { greeting: 'hello world' }
esmRequire('./foo.default.js') // { greeting: 'hello world' }
esmRequire('./foo.esm.js') // { greeting: { greeting: 'hello world' } }
The esmResolver
method works similar to esmRequire
. However, instead of requiring the file, it accepts the object and returns the exported as per the same logic defined above.
import { esmRequire } from '@poppinss/utils'
esmResolver({ greeting: 'hello world' }) // { greeting: 'hello world' }
esmResolver({
default: { greeting: 'hello world' },
__esModule: true,
}) // { greeting: 'hello world' }
esmResolver({
greeting: { greeting: 'hello world' },
__esModule: true,
}) // { greeting: { greeting: 'hello world' } }
Works similar to require.resolve
, however it handles the absolute paths properly.
import { resolveFrom } from '@poppinss/utils'
resolveFrom(__dirname, 'npm-package') // returns path to package "main" file
resolveFrom(__dirname, './foo.js') // returns path to `foo.js` (if exists)
resolveFrom(__dirname, join(__dirname, './foo.js')) // returns path to `foo.js` (if exists)
Lodash itself is a bulky library and most of the times, we don't need all the functions from it. For this purpose, the lodash team decided to publish individual methods to npm as packages. However, most of those individual packages are outdated.
At this point, whether we should use the complete lodash build or use outdated individual packages. Both are not acceptable.
Instead, we make use of lodash-cli
to create a custom build of all the utilities we ever need inside the AdonisJS eco-system and export it as part of this package. Why part of this package?
Well, creating custom builds in multiple packages will cause friction, so it's better to keep it at a single place.
Do note: There are no Typescript types for the lodash methods, since their CLI doesn't generate one and the one published on
@types/lodash
package are again maintained by community and not the lodash core team, so at times, they can also be outdated.
import { lodash } from '@poppinss/utils'
lodash.snakeCase('HelloWorld') // hello_world
Following is the list of exported helpers.
Following helpers for base64 encoding/decoding also exists.
import { base64 } from '@poppinss/utils'
base64.encode('hello world')
base64.encode(Buffer.from('hello world', 'binary'))
import { base64 } from '@poppinss/utils'
base64.decode(base64.encode('hello world'))
base64.decode(base64.encode(Buffer.from('hello world', 'binary')), 'binary')
Same as encode
, but safe for URLS and Filenames
Same as decode
, but decodes the urlEncode
output values
A helper to generate random strings of a given length. Uses crypto
under the hood.
import { randomString } from '@poppinss/utils'
randomString(32)
randomString(128)
Compares two values by avoid timing attack. Accepts any input that can be passed to Buffer.from
import { safeValue } from '@poppinss/utils'
if (safeValue('foo', 'foo')) {
}
Similar to JSON.stringify
, but also handles Circular references by removing them.
import { safeStringify } from '@poppinss/utils'
const o = { b: 1, a: 0 }
o.o = o
console.log(safeStringify(o))
// { "b":1,"a":0 }
console.log(JSON.stringify(o))
// TypeError: Converting circular structure to JSON
Similar to JSON.parse
, but protects against Prototype Poisoning
import { safeParse } from '@poppinss/utils'
const input = '{ "user": { "__proto__": { "isAdmin": true } } }'
JSON.parse(input)
// { user: { __proto__: { isAdmin: true } } }
safeParse(input)
// { user: {} }
Message builder provides a sane API for stringifying objects similar to JSON.stringify
but has a few advantages.
verify
method will respect these values.The message builder alone may seem useless, since anyone can decode the object and change its expiry or purpose. However, you can generate an hash of the stringified object and verify for tampering by validating the hash. This is what AdonisJS does for cookies.
import { MessageBuilder } from '@poppinss/utils'
const builder = new MessageBuilder()
const encoded = builder.build(
{ username: 'virk' },
'1 hour',
'login',
)
Now verify it
builder.verify(encoded) // returns null, no purpose defined
builder.verify(encoded, 'register') // returns null, purpose mismatch.
builder.verify(encoded, 'login') // return { username: 'virk' }
FAQs
Handy utilities for repetitive work
The npm package @poppinss/utils receives a total of 91,364 weekly downloads. As such, @poppinss/utils popularity was classified as popular.
We found that @poppinss/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.