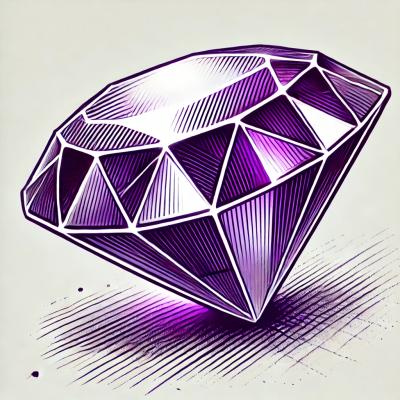
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@poppinss/utils
Advanced tools
@poppinss/utils is a utility library that provides a collection of helper functions for various common tasks in JavaScript and TypeScript. It includes utilities for data manipulation, type checking, and more.
String Manipulation
The string manipulation utilities include functions like camelCase, which converts a string to camel case.
const { string } = require('@poppinss/utils');
const camelCaseString = string.camelCase('hello world');
console.log(camelCaseString); // 'helloWorld'
Object Utilities
Object utilities include functions like clone, which creates a deep copy of an object.
const { object } = require('@poppinss/utils');
const obj = { a: 1, b: 2 };
const clonedObj = object.clone(obj);
console.log(clonedObj); // { a: 1, b: 2 }
Type Checking
Type checking utilities include functions like is.number and is.string, which check the type of a given value.
const { is } = require('@poppinss/utils');
console.log(is.number(123)); // true
console.log(is.string(123)); // false
Lodash is a popular utility library that provides a wide range of functions for data manipulation, type checking, and more. It is more comprehensive and widely used compared to @poppinss/utils.
Underscore is another utility library similar to Lodash, offering a variety of functions for common programming tasks. It is older and less feature-rich than Lodash but still widely used.
Ramda is a functional programming library for JavaScript that provides utility functions with a focus on immutability and pure functions. It offers a different approach compared to @poppinss/utils, emphasizing functional programming paradigms.
Collection of reusable scripts used by AdonisJS core team
This module exports a collection of re-usable utilties to avoid re-writing the same code in every other package. We also include a handful of Lodash utilities, which are used across the AdonisJS packages eco-system.
The version 3.0 re-format the exports to expose an "helpers" subpath to be used within the AdonisJS apps as well.
The idea is to separate helpers that we need to share with AdonisJS core inside its own module, accessible as @poppinss/utils/build/helpers
.
Following modules are now moved to a subpath.
MessageBuilder
base64
compose
fsReadAll
interpolate
requireAll
resolveDir
resolveFrom
// Earlier
import {
MessageBuilder,
base64,
compose,
fsReadAll,
interpolate,
requireAll,
resolveDir,
resolveFrom,
safeEqual
} from '@poppinss/utils'
// After version 3.0
import {
MessageBuilder,
base64,
compose,
fsReadAll,
interpolate,
requireAll,
resolveDir,
resolveFrom,
safeEqual
} from '@poppinss/utils/build/helpers'
The randomString
is now part of the string
helpers.
// Earlier
import { randomString } from '@poppinss/utils'
randomString(32)
// After version 3.0
import { string } from '@poppinss/utils/build/helpers'
string.generateRandom(32)
The following lodash functions have been removed with new alternatives.
snakeCase
camelCase
startCase
// Earlier
import { lodash } from '@poppinss/utils'
lodash.snakeCase()
lodash.camelCase()
lodash.startCase()
// After version 3.0
import { string } from '@poppinss/utils/build/helpers'
string.snakeCase()
string.camelCase()
string.titleCase()
Install the package from npm registry as follows:
npm i @poppinss/utils
# yarn
yarn add @poppinss/utils
and then use it as follows:
import { requireAll } from '@poppinss/utils'
requireAll(__dirname)
A custom exception class that extends the Error
class to add support for defining status
and error codes
.
import { Exception } from '@poppinss/utils'
throw new Exception('Something went wrong', 500, 'E_RUNTIME_EXCEPTION')
throw new Exception('Route not found', 404, 'E_ROUTE_NOT_FOUND')
Utility to require script files wihtout worrying about CommonJs
and ESM
exports. This is how it works.
module.exports
.export default
.export default
.foo.js
module.exports = {
greeting: 'Hello world',
}
foo.default.js
export default {
greeting: 'Hello world',
}
foo.esm.js
export const greeting = {
greeting: 'hello world',
}
import { esmRequire } from '@poppinss/utils'
esmRequire('./foo.js') // { greeting: 'hello world' }
esmRequire('./foo.default.js') // { greeting: 'hello world' }
esmRequire('./foo.esm.js') // { greeting: { greeting: 'hello world' } }
The esmResolver
method works similar to esmRequire
. However, instead of requiring the file, it accepts the object and returns the exported as per the same logic defined above.
import { esmRequire } from '@poppinss/utils'
esmResolver({ greeting: 'hello world' }) // { greeting: 'hello world' }
esmResolver({
default: { greeting: 'hello world' },
__esModule: true,
}) // { greeting: 'hello world' }
esmResolver({
greeting: { greeting: 'hello world' },
__esModule: true,
}) // { greeting: { greeting: 'hello world' } }
Lodash itself is a bulky library and most of the times, we don't need all the functions from it.
Also, all of the lodash functions are published as individual modules on npm. However, most of those individual packages are outdated and using them is not an option.
Instead, we decided to use the lodash-cli
to create a custom build for all the utilities we need inside AdonisJS ecosystem and export it as part of this package.
import { lodash } from '@poppinss/utils'
lodash.get({ name: 'virk' }, 'name') // virk
Following is the list of exported helpers.
Similar to JSON.stringify
, but also handles Circular references by removing them.
import { safeStringify } from '@poppinss/utils'
const o = { b: 1, a: 0 }
o.o = o
console.log(safeStringify(o))
// { "b":1,"a":0 }
console.log(JSON.stringify(o))
// TypeError: Converting circular structure to JSON
Similar to JSON.parse
, but protects against Prototype Poisoning
import { safeParse } from '@poppinss/utils'
const input = '{ "user": { "__proto__": { "isAdmin": true } } }'
JSON.parse(input)
// { user: { __proto__: { isAdmin: true } } }
safeParse(input)
// { user: {} }
Explicitly define static properties on a class by checking for hasOwnProperty
. In case of inheritance, the properties from the parent class are cloned vs following the prototypal inheritance.
We use/need this copy from parent class behavior a lot in AdonisJS. Here's an example of Lucid models
You create an application wide base model
class AppModel extends BaseModel {
@column.datetime()
public createdAt: DateTime
}
AdonisJS will create the $columnDefinitions
property on the AppModel
class, that holds all the columns
AppModel.$columnDefinitions // { createdAt: { columName: created_at } }
Now, lets create another model inheriting the AppModel
class User extends AppModel {
@column()
public id: number
}
As per the Javascript prototypal inheritance. The User
model will not contain the columns from the AppModel
, because we just re-defined the $columnDefinitions
property. However, we don't want this behavior and instead want to copy the columns from the AppModel
and then add new columns to it.
Voila! Use the defineStaticProperty
helper from this class.
class LucidBaseModel {
static boot() {
defineStaticProperty(this, LucidBaseModel, {
propertyName: '$columnDefinitions',
defaultValue: {},
strategy: 'inherit',
})
}
}
The defineStaticProperty
takes a total of three arguments.
this
.propertyName
, defaultValue (in case, there is nothing to copy)
, and the strategy
.inherit
strategy will copy the properties from the base class.define
strategy will always use the defaultValue
to define the property on the class. In other words, there is no copy behavior, but prototypal inheritance chain is also breaked by explicitly re-defining the property.The helpers module is also available in AdonisJS applications as follows:
import { fsReadAll, string, types } from '@ioc:Adonis/Core/Helpers'
The @poppinss/utils
exposes this module as follows
import { fsReadAll, string, types } from '@poppinss/utils/build/helpers'
A utility to recursively read all script files for a given directory. This method is equivalent to
readdir + recursive + filter (.js, .json, .ts)
.
import { fsReadAll } from '@poppinss/utils/build/helpers'
const files = fsReadAll(__dirname) // array of strings
You can also define your custom filter function. The filter function must return true
for files to be included.
const files = fsReadAll(__dirname, (file) => {
return file.endsWith('.foo.js')
})
Same as fsReadAll
, but instead require the files. Helpful when you want to load all the config files inside a directory on app boot.
import { requireAll } from '@poppinss/utils/build/helpers'
const config = requireAll(join(__dirname, 'config'))
{
file1: {}, // exported object
file2: {} // exported object
}
Works similar to require.resolve
, however it handles the absolute paths properly.
import { resolveFrom } from '@poppinss/utils/build/helpers'
resolveFrom(__dirname, 'npm-package') // returns path to package "main" file
resolveFrom(__dirname, './foo.js') // returns path to `foo.js` (if exists)
resolveFrom(__dirname, join(__dirname, './foo.js')) // returns path to `foo.js` (if exists)
The require.resolve
or resolveFrom
method can only resolve paths to a given file and not the directory. For example: If you pass path to a directory, then it will search for index.js
inside it and in case of a package, it will be search for main
entry point.
On the other hand, the resolveDir
method can also resolve path to directories using following resolution.
./
or .\
are resolved using path.join
.node_modules
are resolved as follows: - Uses require.resolve
to resolve the package.json
file. - Then replace the package-name
with the absolute resolved package path.import { resolveDir } from '@poppinss/utils/build/helpers'
resolveDir(__dirname, './database/migrations')
// __dirname + /database/migrations
resolveDir(__dirname, 'some-package/database/migrations')
// {path-to-package}/database/migrations
resolveDir(__dirname, '@some/package/database/migrations')
// {path-to-package}/database/migrations
A small utility function to interpolate values inside a string.
import { interpolate } from '@poppinss/utils/build/helpers'
interpolate('hello {{ username }}', {
username: 'virk'
})
interpolate('hello {{ users.0.username }}', {
users: [{ username: 'virk' }]
})
If value is missing, it will be replaced with an 'undefined'
string.
Use the \
to escape a mustache block from getting evaluated.
import { interpolate } from '@poppinss/utils/build/helpers'
interpolate('\\{{ username }} expression evaluates to {{ username }}', {
username: 'virk'
})
// Output: {{ username }} expression evaluates to virk
Following helpers for base64 encoding/decoding also exists.
import { base64 } from '@poppinss/utils/build/helpers'
base64.encode('hello world')
base64.encode(Buffer.from('hello world', 'binary'))
import { base64 } from '@poppinss/utils/build/helpers'
base64.decode(base64.encode('hello world'))
base64.decode(base64.encode(Buffer.from('hello world', 'binary')), 'binary')
Same as encode
, but safe for URLS and Filenames
Same as decode
, but decodes the urlEncode
output values
Compares two values by avoid timing attack. Accepts any input that can be passed to Buffer.from
import { safeValue } from '@poppinss/utils/build/helpers'
if (safeValue('foo', 'foo')) {
}
Message builder provides a sane API for stringifying objects similar to JSON.stringify
but has a few advantages.
verify
method will respect these values.The message builder alone may seem useless, since anyone can decode the object and change its expiry or purpose. However, you can generate an hash of the stringified object and verify the tampering by validating the hash. This is what AdonisJS does for cookies.
import { MessageBuilder } from '@poppinss/utils/build/helpers'
const builder = new MessageBuilder()
const encoded = builder.build({ username: 'virk' }, '1 hour', 'login')
Now verify it
builder.verify(encoded) // returns null, no purpose defined
builder.verify(encoded, 'register') // returns null, purpose mismatch.
builder.verify(encoded, 'login') // return { username: 'virk' }
Javascript doesn't have a concept of inherting multiple classes together and neither does Typescript. However, the official documentation of Typescript does talks about the concept of mixins.
As per the Typescript docs, you can create and apply mixins as follows.
type Constructor = new (...args: any[]) => any
const UserWithEmail = <T extends Constructor>(superclass: T) => {
return class extends superclass {
public email: string
}
}
const UserWithPassword = <T extends Constructor>(superclass: T) => {
return class extends superclass {
public password: string
}
}
class BaseModel {}
class User extends UserWithPassword(UserWithEmail(BaseModel)) {}
Mixins are close to a perfect way of inherting multiple classes. I recommend reading this article for same.
However, the syntax of applying multiple mixins is kind of ugly, as you have to apply mixins over mixins, creating a nested hierarchy as shown below.
UserWithAttributes(UserWithAge(UserWithPassword(UserWithEmail(BaseModel))))
The compose
method is a small utility to improve the syntax a bit.
import { compose } from '@poppinss/utils/build/helpers'
class User extends compose(
BaseModel,
UserWithPassword,
UserWithEmail,
UserWithAge,
UserWithAttributes
) {}
Typescript has an open issue related to the constructor arguments of the mixin class or the base class.
Typescript expects all classes used in the mixin chain to have a constructor with only one argument of ...args: any[]
. For example: The following code will work fine at runtime, but the typescript compiler complains about it.
class BaseModel {
constructor(name: string) {}
}
const UserWithEmail = <T extends typeof BaseModel>(superclass: T) => {
return class extends superclass {
// ERROR: A mixin class must have a constructor with a single rest parameter of type 'any[]'.ts(2545)
public email: string
}
}
class User extends compose(BaseModel, UserWithEmail) {}
You can work around this by overriding the constructor of the base class.
import { NormalizeConstructor, compose } from '@poppinss/utils/build/helpers'
const UserWithEmail = <T extends NormalizeConstructor<typeof BaseModel>>(superclass: T) => {
return class extends superclass {
public email: string
}
}
The string
module includes a bunch of helper methods to work with strings.
Convert a string to its camelCase
version.
import { string } from '@poppinss/utils/build/helpers'
string.camelCase('hello-world') // helloWorld
Convert a string to its snake_case
version.
import { string } from '@poppinss/utils/build/helpers'
string.snakeCase('helloWorld') // hello_world
Convert a string to its dash-case
version. Optionally, you can also capitalize the first letter of each segment.
import { string } from '@poppinss/utils/build/helpers'
string.dashCase('helloWorld') // hello-world
string.dashCase('helloWorld', { capitalize: true }) // Hello-World
Convert a string to its PascalCase
version.
import { string } from '@poppinss/utils/build/helpers'
string.pascalCase('helloWorld') // HelloWorld
Capitalize a string
import { string } from '@poppinss/utils/build/helpers'
string.capitalCase('helloWorld') // Hello World
Convert string to a sentence
import { string } from '@poppinss/utils/build/helpers'
string.sentenceCase('hello-world') // Hello world
Convert string to its dot.case
version.
import { string } from '@poppinss/utils/build/helpers'
string.dotCase('hello-world') // hello.world
Remove all sorts of casing
import { string } from '@poppinss/utils/build/helpers'
string.noCase('hello-world') // hello world
string.noCase('hello_world') // hello world
string.noCase('helloWorld') // hello world
Convert a sentence to title case
import { string } from '@poppinss/utils/build/helpers'
string.titleCase('Here is a fox') // Here Is a fox
Pluralize a word.
import { string } from '@poppinss/utils/build/helpers'
string.pluralize('box') // boxes
string.pluralize('i') // we
You can also define your own irregular rules using the string.defineIrregularRule
method.
import { string } from '@poppinss/utils/build/helpers'
string.defineIrregularRule('auth', 'auth')
string.plural('auth') // auth
You can also define your own uncountable rules using the string.defineUncountableRule
method.
import { string } from '@poppinss/utils/build/helpers'
string.defineUncountableRule('login')
string.plural('login') // home
Truncate a string after a given number of characters
import { string } from '@poppinss/utils/build/helpers'
string.truncate(
'This is a very long, maybe not that long title',
12
) // This is a ve...
By default, the string is truncated exactly after the given characters. However, you can instruct the method to wait for the words to complete.
string.truncate(
'This is a very long, maybe not that long title',
12,
{
completeWords: true
}
) // This is a very...
Also, it is possible to customize the suffix.
string.truncate(
'This is a very long, maybe not that long title',
12,
{
completeWords: true,
suffix: ' <a href="/1"> Read more </a>',
}
) // This is a very <a href="/1"> Read more </a>
The excerpt
method is same as the truncate
method. However, it strips the HTML from the string.
import { string } from '@poppinss/utils/build/helpers'
string.excerpt(
'<p>This is a <strong>very long</strong>, maybe not that long title</p>',
12
) // This is a very...
Condense whitespaces from a given string. The method removes the whitespace from the left
, right
and multiple whitespace in between the words.
import { string } from '@poppinss/utils/build/helpers'
string.condenseWhitespace(' hello world ')
// hello world
Escape HTML from the string
import { string } from '@poppinss/utils/build/helpers'
string.escapeHTML('<p> foo © bar </p>')
// <p> foo © bar </p>
Additonally, you can also encode non-ascii symbols
import { string } from '@poppinss/utils/build/helpers'
string.escapeHTML(
'<p> foo © bar </p>',
{
encodeSymbols: true
}
)
// <p> foo © bar </p>
Encode symbols. Checkout he for available options
import { string } from '@poppinss/utils/build/helpers'
string.encodeSymbols('foo © bar')
// foo © bar
Join an array of words with a separator.
import { string } from '@poppinss/utils/build/helpers'
string.toSentence([
'route',
'middleware',
'controller'
]) // route, middleware, and controller
string.toSentence([
'route',
'middleware'
]) // route and middleware
You can also customize
separator
: The value between two words except the last onepairSeparator
: The value between the first and the last word. Used, only when there are two wordslastSeparator
: The value between the second last and the last word. Used, only when there are more than two wordsstring.toSentence([
'route',
'middleware',
'controller'
], {
separator: '/ ',
lastSeparator: '/or '
}) // route/ middleware/or controller
Convert bytes value to a human readable string. For options, recommend the bytes package.
import { string } from '@poppinss/utils/build/helpers'
string.prettyBytes(1024) // 1KB
string.prettyBytes(1024, { unitSeparator: ' ' }) // 1 KB
Convert human readable string to bytes. This method is the opposite of the prettyBytes
method.
import { string } from '@poppinss/utils/build/helpers'
string.toBytes('1KB') // 1024
Convert time in milliseconds to a human readable string
import { string } from '@poppinss/utils/build/helpers'
string.prettyMs(60000) // 1min
string.prettyMs(60000, { long: true }) // 1 minute
Convert human readable string to milliseconds. This method is the opposite of the prettyMs
method.
import { string } from '@poppinss/utils/build/helpers'
string.toMs('1min') // 60000
Ordinalize a string or a number value
import { string } from '@poppinss/utils/build/helpers'
string.ordinalize(1) // 1st
string.ordinalize(99) // 99th
Generate a cryptographically strong random string
import { string } from '@poppinss/utils/build/helpers'
string.generateRandom(32)
Find if a value is empty. Also checks for empty strings with all whitespace
import { string } from '@poppinss/utils/build/helpers'
string.isEmpty('') // true
string.isEmpty(' ') // true
The types module allows distinguishing between different Javascript datatypes. The typeof
returns the same type for many different values. For example:
typeof ({}) // object
typeof ([]) // object
typeof (null) // object
WHAT??? Yes, coz everything is an object in Javascript. To have better control, you can make use of the types.lookup
method.
Returns a more accurate type for a given value.
import { types } from '@poppinss/utils/build/helpers'
types.lookup({}) // object
types.lookup([]) // array
types.lookup(Object.create(null)) // object
types.lookup(null) // null
types.lookup(function () {}) // function
types.lookup(class Foo {}) // class
types.lookup(new Map()) // map
Find if the given value is null
import { types } from '@poppinss/utils/build/helpers'
types.isNull(null)) // true
Find if the given value is a boolean
import { types } from '@poppinss/utils/build/helpers'
types.isBoolean(true)) // true
Find if the given value is a buffer
import { types } from '@poppinss/utils/build/helpers'
types.isBuffer(new Buffer())) // true
Find if the given value is a number
import { types } from '@poppinss/utils/build/helpers'
types.isNumber(100)) // true
Find if the given value is a string
import { types } from '@poppinss/utils/build/helpers'
types.isString('hello')) // true
Find if the given value is an arguments object
import { types } from '@poppinss/utils/build/helpers'
function foo() {
types.isArguments(arguments)) // true
}
Find if the given value is a plain object
import { types } from '@poppinss/utils/build/helpers'
types.isObject({})) // true
Find if the given value is a date object
import { types } from '@poppinss/utils/build/helpers'
types.isDate(new Date())) // true
Find if the given value is an array
import { types } from '@poppinss/utils/build/helpers'
types.isArray([1, 2, 3])) // true
Find if the given value is an regular expression
import { types } from '@poppinss/utils/build/helpers'
types.isRegexp(/[a-z]+/)) // true
Find if the given value is an instance of the error object
import { types } from '@poppinss/utils/build/helpers'
import { Exception } from '@poppinss/utils'
types.isError(new Error('foo'))) // true
types.isError(new Exception('foo'))) // true
Find if the given value is a function
import { types } from '@poppinss/utils/build/helpers'
types.isFunction(function foo() {})) // true
Find if the given value is a class constructor. Uses regex to distinguish between a function and a class.
import { types } from '@poppinss/utils/build/helpers'
class User {}
types.isClass(User) // true
types.isFunction(User) // true
Find if the given value is an integer.
import { types } from '@poppinss/utils/build/helpers'
types.isInteger(22.00) // true
types.isInteger(22) // true
types.isInteger(-1) // true
types.isInteger(-1.00) // true
types.isInteger(22.10) // false
types.isInteger(.3) // false
types.isInteger(-.3) // false
Find if the given value is an float number.
import { types } from '@poppinss/utils/build/helpers'
types.isFloat(22.10) // true
types.isFloat(-22.10) // true
types.isFloat(.3) // true
types.isFloat(-.3) // true
types.isFloat(22.00) // false
types.isFloat(-22.00) // false
types.isFloat(-22) // false
Find if the given value has a decimal. The value can be a string or a number. The number values are casted to a string by calling the toString()
method on the value itself.
The string conversion is peformed to test the value against a regex. Since, there is no way to natively find a decimal value in Javascript.
import { types } from '@poppinss/utils/build/helpers'
types.isDecimal('22.10') // true
types.isDecimal(22.1) // true
types.isDecimal('-22.10') // true
types.isDecimal(-22.1) // true
types.isDecimal('.3') // true
types.isDecimal(0.3) // true
types.isDecimal('-.3') // true
types.isDecimal(-0.3) // true
types.isDecimal('22.00') // true
types.isDecimal(22.0) // false (gets converted to 22)
types.isDecimal('-22.00') // true
types.isDecimal(-22.0) // false (gets converted to -22)
types.isDecimal('22') // false
types.isDecimal(22) // false
types.isDecimal('0.0000000000001') // true
types.isDecimal(0.0000000000001) // false (gets converted to 1e-13)
A very simple class to conditionally builder an object. Quite often, I create a new object from an existing one and wants to avoid writing undefined values to it. For example
const obj = {
...(user.username ? { username: user.username } : {}),
...(user.id ? { id: user.id } : {}),
...(user.createdAt ? { createdAt: user.createdAt.toString() } : {}),
}
Not only the above code is harder to write. It is performance issues as well, since we are destructuring too many objects.
To address this use case, you can make use of the ObjectBuilder
class as follows
import { ObjectBuilder } from '@poppinss/utils/build/helpers'
const obj = new ObjectBuilder()
.add('username', user.username)
.add('id', user.id)
.add('createdAt', user.createdAt && user.createdAt.toString())
.value // returns the underlying object
The add
method ignores the value if its undefined
. So it never gets added to the object at all. You can also ignore null
properties by passing a boolean flag to the constructor.
new ObjectBuilder(true) // ignore null as well
FAQs
Handy utilities for repetitive work
The npm package @poppinss/utils receives a total of 91,364 weekly downloads. As such, @poppinss/utils popularity was classified as popular.
We found that @poppinss/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.