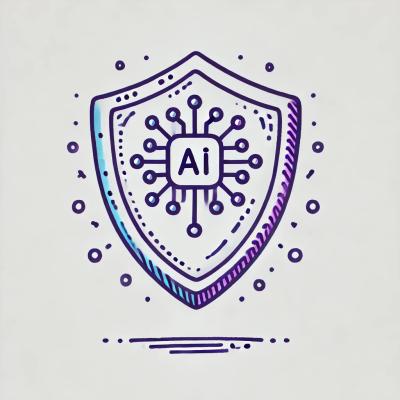
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
@porscheofficial/cookie-consent-banner-react
Advanced tools
<img src="https://github.com/porscheofficial/cookie-consent-banner/raw/main/assets/logo.svg" alt="" width="200" heigh
The Cookie Consent Banner is implemented as Web Component and additionally exported as React Component. This guide explains how to use the React Component.
Click here to have a look on the documentation of the Web Component.
Make sure to also have a look on the main repository.
// yarn
yarn add @porscheofficial/cookie-consent-banner-react
// or npm
npm i @porscheofficial/cookie-consent-banner-react --S
Property (React) | Default | Type | Description |
---|---|---|---|
availableCategories | [] | CategoryItem[] | Provide the available Categories. See Real World example |
cookieAttributes | {path: "/",expires: 7,domain: document.location.hostname} | CookieAttributes | Customization of the cookie attributes for the consent cookie (expires can either be a number of days or a Date object). |
cookieName | "cookies_accepted_categories" | string | |
disableResetSiteCookiesOnConsentWithdrawn | false | boolean | Prevent cookies from being deleted automatically if consent of the user changed. |
disableSlideInAnimation | false | boolean | Disable slide-in animation of banner (See #7) |
headline | undefined | string | |
btnLabelAcceptAndContinue | undefined | string | |
btnLabelAllAndContinue | undefined | string | |
btnLabelOnlyEssentialAndContinue | undefined | string | |
btnLabelPersistSelectionAndContinue | undefined | string | |
contentSettingsDescription | undefined | string | |
handlePreferencesRestored | undefined | ({ acceptedCategories, }: { acceptedCategories: string[]; }) => void | |
handlePreferencesUpdated | undefined | ({ acceptedCategories, }: { acceptedCategories: string[]; }) => void |
Instead of listening to the events directly, the props handlePreferencesRestored
and handlePreferencesUpdated
can be used.
Event | Description |
---|---|
cookie_consent_preferences_restored | Consent Settings have been saved previously and are now restored on Page Load. |
cookie_consent_preferences_updated | Consent Settings have been updated. Either initially set or changed. |
It's possible to send JavaScript Events to control the banner.
Event | Description |
---|---|
cookie_consent_show | Show the the Consent Banner. |
cookie_consent_details_show | Show the detailed settings (categories). Also the Consent Banner is shown if not done already. |
In order to allow the user to always update its preferences it's possible to trigger the banner by sending an event:
<a href="javascript:document.dispatchEvent(new Event('cookie_consent_show'))">
Show Cookie Settings
</a>
useCookieConsent
Subscribe to changes of the consent.
import { useCookieConsent } from "@porscheofficial/cookie-consent-banner-react";
export const SomePage: React.FC = () => {
const acceptedCategories = useCookieConsent();
//...
};
triggerCookieConsentBanner
Easily show the cookie consent banner.
import { triggerCookieConsentBanner } from "@porscheofficial/cookie-consent-banner-react";
// Only the banner
triggerCookieConsentBanner();
// Also show the details
triggerCookieConsentBanner({ showDetails: true });
Styling can be done in two different ways: Either via the availabe CSS Properties or via the CSS ::part
pseudo-element.
The appearance of the component can be influenced by updating the availabe CSS Properties. In most cases this is enough customization flexibility but since not every aspect is exposed, you might want to use the ::part
selectors if you need more flexibility.
<style>
:root {
/* COLORS */
--cookie-consent-banner-colors-primary: #81c784;
--cookie-consent-banner-colors-primary-border: #81c784;
--cookie-consent-banner-colors-primary-content: #fff;
--cookie-consent-banner-colors-secondary: transparent;
--cookie-consent-banner-colors-secondary-border: #fff;
--cookie-consent-banner-colors-secondary-content: #fff;
--cookie-consent-banner-colors-background-body: rgba(25, 31, 34, 0.92);
--cookie-consent-banner-colors-text: #fff;
/* BORDER-RADIUS */
--cookie-consent-banner-border-radius-buttons: 1rem;
--cookie-consent-banner-border-radius-body: 0;
// BOX-SHADOW
--cookie-consent-banner-box-shadow: 0px -3px 13px 0px rgba(57, 57, 57, 0.38);
/* SPACINGS */
--cookie-consent-banner-spacings-container-padding-top: 1rem;
--cookie-consent-banner-spacings-container-padding-left: 1rem;
--cookie-consent-banner-spacings-container-padding-bottom: 1rem;
--cookie-consent-banner-spacings-container-padding-right: 1rem;
--cookie-consent-banner-spacings-body-padding-top: 0;
--cookie-consent-banner-spacings-body-padding-left: 2rem;
--cookie-consent-banner-spacings-body-padding-bottom: 0;
--cookie-consent-banner-spacings-body-padding-right: 2rem;
/* Z-INDEX */
--cookie-consent-banner-z-index-container: 99;
/* FONTS */
--cookie-consent-banner-font-family-headline: inherit;
--cookie-consent-banner-font-size-headline: 1.5rem;
--cookie-consent-banner-font-family-body: inherit;
--cookie-consent-banner-font-size-body: 0.875rem;
}
</style>
::part
selectorsFor full control over the styles we provide you these CSS parts to customize completely by your own:
cookie-consent-banner::part(button-accept-all)
for styling the primary button which triggers "Accept All Cookies"cookie-consent-banner::part(button-persist-selection)
for styling the secondary button which triggers "Save Selection"cookie-consent-banner::part(button-essential-only)
for styling the secondary button which triggers "Only required Cookies"cookie-consent-banner::part(checkbox)
for styling the checkboxescookie-consent-banner::part(description-main)
for styling the main description textcookie-consent-banner::part(description-settings)
for styling the description text on the expanded settingscookie-consent-banner::part(headline)
for styling the headlinecookie-consent-banner::part(body)
for styling the banner body<style>
cookie-consent-banner::part(button-accept-all) {
text-transform: uppercase;
}
cookie-consent-banner::part(button-persist-selection),
cookie-consent-banner::part(button-essential-only) {
text-transform: uppercase;
box-shadow: 0 2px 5px 0 rgba(0, 0, 0, 0.26);
}
cookie-consent-banner::part(checkbox) {
accent-color: rgb(24, 251, 107);
}
cookie-consent-banner::part(description),
cookie-consent-banner::part(headline) {
font-family: "Arial", sans-serif;
}
</style>
This example shows how the component could be integrated into your web application.
Additional scripts that set cookies, for which the consent of the visitor is required, can either be loaded via a tag manager or programmatically.
In the following example, an ErrorTrackingService
is initialized after the consent has been given and a Tag Manager Script is added to the head of the page.
Once the visitor stores the consent settings, two things happen: The consent data is stored as a cookie cookies_accepted_categories
and the tag manager is initialized, which takes care of other scripts (tags).
Have a look on the main repository for an example consent flow.
import {
CookieConsentBanner,
triggerCookieConsentBanner,
} from "@porscheofficial/cookie-consent-banner-react";
const initConsent = ({ acceptedCategories }) => {
// -------------------------------------------------------------------------
// Error Tracking Service
// Analytics
// -------------------------------------------------------------------------
if (acceptedCategories.includes("analytics")) {
ErrorTrackingService.init({
dsn: process.env.DSN,
environment: process.env.ENV,
});
}
};
const Root = ({ children }) => {
const [acceptedCategories, setAcceptedCategories] = useState([]);
return (
<div>
<Helmet>
{(Boolean(acceptedCategories.includes("analytics")) ||
Boolean(acceptedCategories.includes("marketing"))) &&
process.env.ENV === "prod" && (
<script id="tagmanager-script" key="tagmanager-script">
{/* Load Tag Manager Script */}
</script>
)}
</Helmet>
<div>{children}</div>
<CookieConsentBanner
handlePreferencesUpdated={initConsent}
handlePreferencesRestored={initConsent}
btnLabelAcceptAndContinue="Agree and continue"
btnLabelSelectAllAndContinue="Select all and continue"
btnLabelOnlyEssentialAndContinue="Continue with technically required cookies only"
btnLabelPersistSelectionAndContinue="Save selection and continue"
contentSettingsDescription="You can decide which cookies are used by selecting the respective options below. Please note that your selection may impair in the functionality of the service."
availableCategories={[
{
description:
"Enable you to navigate and use the basic functions and to store preferences.",
key: "technically_required",
label: "Technically necessary cookies",
isMandatory: true,
},
{
description:
"Enable us to determine how visitors interact with our service in order to improve the user experience.",
key: "analytics",
label: "Analysis cookies",
},
{
description:
"Enable us to offer and evaluate relevant content and interest-based advertising.",
key: "marketing",
label: "Marketing cookies",
},
]}
>
We use cookies and similar technologies to provide certain features,
enhance the user experience and deliver content that is relevant to your
interests. Depending on their purpose, analysis and marketing cookies
may be used in addition to technically necessary cookies. By clicking on
"Agree and continue", you declare your consent to the use of
the aforementioned cookies.
<button
onClick={() => {
triggerCookieConsentBanner({ showDetails: true });
}}
onKeyPress={() => {
triggerCookieConsentBanner({ showDetails: true });
}}
type="button"
>
Here
</button> you can make detailed settings or revoke your consent (in part
if necessary) with effect for the future. For further information, please
refer to our
<a href="/privacy-policy">Privacy Policy</a>.
</CookieConsentBanner>
</div>
);
};
Please note that you must individually assess the legal requirements regarding the implementation of the Cookie Consent Banner, in particular which choices to offer in which granularity and which information to provide in which detail and at which point of the user journey. The examples mentioned are not intended to provide any advice regarding legal requirements. All responsibility for the implementation of the Cookie Consent Banner and its compliance with legal requirements lies with the user.
See LICENSE.
FAQs
<img src="https://github.com/porscheofficial/cookie-consent-banner/raw/main/assets/logo.svg" alt="" width="200" heigh
The npm package @porscheofficial/cookie-consent-banner-react receives a total of 85 weekly downloads. As such, @porscheofficial/cookie-consent-banner-react popularity was classified as not popular.
We found that @porscheofficial/cookie-consent-banner-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.