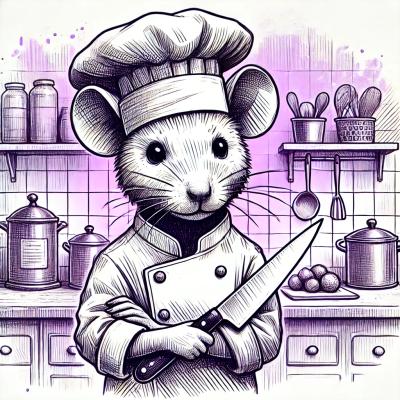
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@posva/vuefire-core
Advanced tools
Shared code for vue + Firebase apps used by vuefire and vuexfire
Core logic used for vuefire and vuexfire
This library is intended for internal usage. You are free to use it to create your own plugins but keep in mind that the main target is a Vue plugin
npm i @posva/vuefire-core
import { bindCollection, bindDocument, walkSet } from '@posva/vuefire-core'
// create an object of operations
const ops = {
set: (target, path, value) => walkSet(target, path, value),
add: (array, index, data) => array.splice(index, 0, data),
remove: (array, index) => array.splice(index, 1),
}
const vm = new Vue({
// options
})
const resolve = data => {
console.log('reference bound:', data)
}
const reject = err => {
console.log('error binding reference:', err)
}
// unbind is a function that tears down all listeners
const unbindItems = bindCollection(
{
// vm could be just an object
vm,
// key set on vm
key: 'items',
ops,
collection: db.collection('items'),
// this is to enable Promise based APIs
// callback on success
resolve,
// callback on error
reject,
},
// default options
{
maxRefDepth: 2,
}
)
const unbindItem = bindDocument(
{
// same options as bindCollection except for collection -> document
document: db.collection('items').doc('0'),
},
options
)
unbindItems()
bindCollection({
// bind a different collection
key: 'items',
})
FAQs
Shared code for vue + Firebase apps used by vuefire and vuexfire
We found that @posva/vuefire-core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.