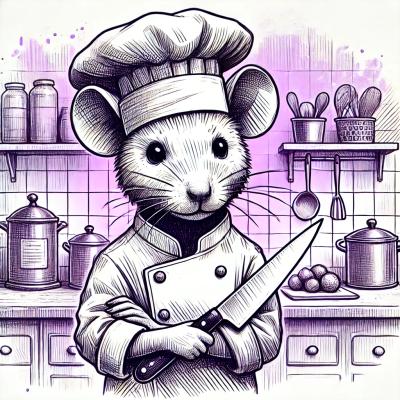
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@pradeepvish1213/dataseers-google-place-api
Advanced tools
Node client for Google Places API (placeSearch, placeDetails, placePhoto). Written by pradeep.
Node client to run the basic google places API requests (using axios). Published on NPM.
$ npm install dcts-google-places-api
const apiKey = "INSERT_YOUR_API_KEY"; // define API key
const GooglePlacesApi = require('dcts-google-places-api');
const googleapi = new GooglePlacesApi(apiKey); // initialize
const searchQuery = "Pizza New York"; // example search
// with promises
googleapi.runPlaceSearch(searchQuery).then(placeId => {
console.log(placeId); // returns placeId (as string) or null
});
// with async await
(async () => {
let placeId = await googleapi.runPlaceSearch(searchQuery);
console.log(placeId);
})();;
Get place details by place_id
.
const placeId = "ChIJHegKoJUfyUwRjMxaCcviZDA"; // placeId of "Pizza Chicken New York"
// with promises
googleapi.runPlaceDetails(placeId).then(placeDetails => {
console.log(`place found: ${placeDetails.name}`);
})
// with async await
(async () => {
let placeDetails = await googleapi.runPlaceDetails(placeId);
console.log(`place found: ${placeDetails.name}`);
})();
Get place details by cid
. This is a deprecated endpoint and not documented in the official google api documentation, but it still works. See also this answer on stackoverflow
const cid = "10056734717913051463"; // cid of "Brooklyn Boulders Chicago"
// with promises
googleapi.runPlaceDetailsCid(cid).then(placeDetails => {
console.log(`place found: ${placeDetails.name} (${placeDetails.cid})`);
});
// with async await
(async () => {
let placeDetails = await googleapi.runPlaceDetailsCid(cid);
console.log(`place found: ${placeDetails.name} (${placeDetails.cid})`);
})();
⚠️ Be Aware: this function returns only the photoUrl, not the image itself.
const photoReference = 'CmRaAAAAqYV1efHXXLX3UB1msekeprgOUD362n4-8lxwYI3aSFANLw51oE1_KeNziEgnnbr5WQzJtQo9SbNnZFRfymg594T9h7yRWnLQL8w1n_ekN6BbyJzg1k0hadSJ4N0i63TmEhA3NIzf_JWUEZcW3VgXJ5FqGhRq7ij6D2Vl8DOSF2yHY1iuTYuAKA';
// with promises
googleapi.runPlacePhotos(photoReference).then(photoUrl => {
console.log(`photo url: ${photoUrl}`);
});
// with async await
(async () => {
let photoUrl = await googleapi.runPlacePhotos(photoReference);
console.log(`photo url: ${photoUrl}`);
})();
const textSearch = "Nolita Pizza New York";
// with promises
googleapi.runPlaceSearch(textSearch).then(placeId => {
console.log(`place id found: ${placeId}`);
return googleapi.runPlaceDetails(placeId);
}).then(placeDetails => {
console.log(`place details fetched for ${placeDetails.name} (${placeDetails.place_id})`);
const promises = [];
placeDetails.photos.map(photo => {
promises.push(googleapi.runPlacePhotos(photo.photo_reference));
});
return Promise.all(promises);
}).then(placePhotoUrls => {
console.log(`${placePhotoUrls.length} photo urls fetched:`);
console.log(placePhotoUrls);
});
// with async await
(async () => {
let placeId = await googleapi.runPlaceSearch(textSearch)
console.log(`place id found: ${placeId}`);
let placeDetails = await googleapi.runPlaceDetails(placeId);
console.log(`place details fetched for ${placeDetails.name} (${placeDetails.place_id})`);
const placePhotoUrls = [];
for (const photo of placeDetails.photos) {
placePhotoUrls.push(await googleapi.runPlacePhotos(photo.photo_reference));
}
console.log(`${placePhotoUrls.length} photo urls fetched:`);
console.log(placePhotoUrls);
})();
npm run test
npm run test
to see missing tests.Written by pradeepvish1213 for personal use only.
FAQs
Node client for Google Places API (placeSearch, placeDetails, placePhoto). Written by pradeep.
We found that @pradeepvish1213/dataseers-google-place-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.