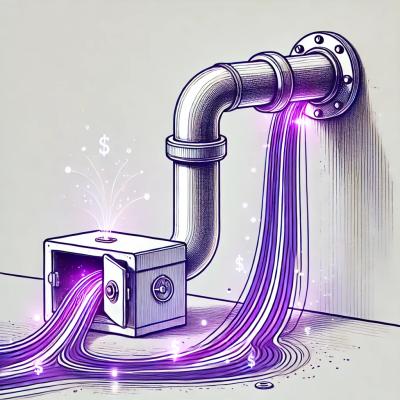
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@printloop/frame-sync
Advanced tools
Minimal iframe synchronization for React with Zod validation
@printloop/frame-sync
A minimal iframe synchronization library for React with runtime type checking via Zod.
NOTE I thought about this API for half a second, so it's probably not the best. I haven't even written tests for it yet. Use at your own risk.
npm install --save @printloop/frame-sync
GuestFrame
component with the schema and the URL of the guestGuestFrame
component as propsimport { z } from 'zod';
import { getGuestFrame } from '@printloop/frame-sync';
const schema = z.object({
name: z.string(),
age: z.number(),
email: z.string().optional(),
});
const GuestFrame = getGuestFrame({
schema,
// the URL of the guest
src: "https://guest-url.com/path/to/page",
// the origin of the guest
targetOrigin: "https://guest-url.com",
});
<GuestFrame name="Randall" age={50} email="randall@printloop.dev" />;
Host
object with the schema, the origin of the host, and initial state to use before the host sends dataHost
through useHostProps
import { z } from 'zod';
import { getHost } from '@printloop/frame-sync';
const schema = z.object({
name: z.string(),
age: z.number(),
email: z.string().optional(),
});
const { useHostProps } = getHost({
schema,
// the origin of the host
origin: "http://host-url.com",
// initial state to use before the host sends data
initialState: {
name: "Alex",
age: 50,
email: "alex@example.com"
}
});
export function SomeGuestComponent () {
const { name, age, email } = useHostProps(Host.Context);
return (
<div>
<p>Name: {name}</p>
<p>Age: {age}</p>
<p>Email: {email}</p>
</div>
);
}
© 2023 Kristian Muñiz
FAQs
Minimal iframe synchronization for React with Zod validation
The npm package @printloop/frame-sync receives a total of 0 weekly downloads. As such, @printloop/frame-sync popularity was classified as not popular.
We found that @printloop/frame-sync demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.