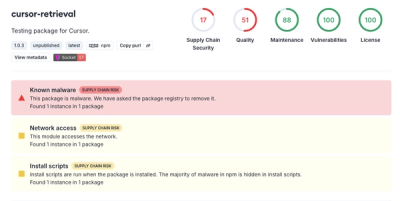
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@procore-canary/redux-modules
Advanced tools
A library for defining clear, boilerplate free Redux reducers.
redux-modules
is a refinement on the Redux module concept with developer experience in mind. It provides:
npm install redux-modules --save
Here's an example of a simple todo app. First create a module that allows todos to be created and destroyed.
import { createModule } from 'redux-modules';
import { propCheck } from 'redux-modules-middleware';
import { fromJS, List } from 'immutable';
import { shape, string, number } from 'prop-types';
export default createModule({
name: 'todos',
initialState: List(),
selector: state => ({ todos: state.get('todos') }),
transformations: {
create: {
middleware: [
middleware.propCheck(
shape({ description: string.isRequired })
),
],
reducer: (state, { payload }) =>
state.update('collection', todos => todos.push(fromJS(payload))),
},
destroy: {
middleware: [
middleware.propCheck(number.isRequired),
],
reducer: (state, { payload }) =>
state.update('collection', todos => todos.delete(payload)),
},
},
});
Once the module is complete, the reducer has to be added to the store.
const store = createStore(todoModule.reducer, {});
export default const App = props => (
<Provider store={store}>
<Todos {...props}/>
</Provider>
)
Alternatively, use ModuleProvider
to allow reducers to be automatically added to the store at runtime.
import { ModuleProvider } from 'redux-modules';
const store = createStore(state => state, {});
export default const App = props => (
<ModuleProvider store={store}>
<Todos {...props}/>
</ModuleProvider>
)
The last step is to connect the module to the view. This works like a normal Redux connect
with the added bonus of auto dispatching and namespacing actions.
import { connectModule } from 'redux-modules';
import { Component, PropTypes } from 'react';
import { array, func, shape } from 'prop-types';
@connectModule(todoModule)
export default class Todos extends Component {
static propTypes = {
todos: array,
actions: shape({
create: func,
destroy: func,
}),
};
That's it! Check the documentation for comparisons with idiomatic Redux, in depth examples, and advanced usage.
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
A library for defining clear, boilerplate free Redux reducers.
The npm package @procore-canary/redux-modules receives a total of 0 weekly downloads. As such, @procore-canary/redux-modules popularity was classified as not popular.
We found that @procore-canary/redux-modules demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.