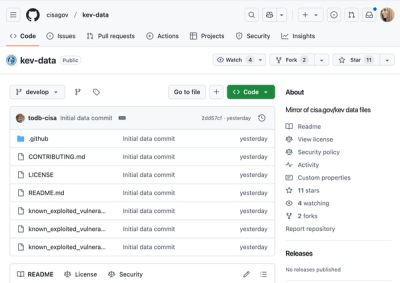
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
@progress/kendo-react-popup
Advanced tools
@progress/kendo-react-popup is a React component library that provides a Popup component for displaying content in a popup window. It is part of the KendoReact suite of UI components and is designed to be highly customizable and easy to integrate into React applications.
Basic Popup
This code demonstrates a basic usage of the Popup component. It toggles the visibility of the popup when the button is clicked and anchors the popup to a specific element.
import React from 'react';
import { Popup } from '@progress/kendo-react-popup';
const BasicPopup = () => {
const [show, setShow] = React.useState(false);
return (
<div>
<button onClick={() => setShow(!show)}>Toggle Popup</button>
<Popup show={show} anchor={document.getElementById('anchor')}>Hello, World!</Popup>
<div id="anchor" style={{ marginTop: '20px' }}>Anchor Element</div>
</div>
);
};
export default BasicPopup;
Custom Popup Position
This code demonstrates how to customize the position of the Popup component. The popup is displayed at a specific position relative to the anchor element.
import React from 'react';
import { Popup } from '@progress/kendo-react-popup';
const CustomPositionPopup = () => {
const [show, setShow] = React.useState(false);
return (
<div>
<button onClick={() => setShow(!show)}>Toggle Popup</button>
<Popup show={show} anchor={document.getElementById('anchor')} position={{ top: 50, left: 100 }}>Custom Position</Popup>
<div id="anchor" style={{ marginTop: '20px' }}>Anchor Element</div>
</div>
);
};
export default CustomPositionPopup;
Popup with Animation
This code demonstrates how to add animations to the Popup component. The popup will animate when it opens and closes with specified durations.
import React from 'react';
import { Popup } from '@progress/kendo-react-popup';
const AnimatedPopup = () => {
const [show, setShow] = React.useState(false);
return (
<div>
<button onClick={() => setShow(!show)}>Toggle Popup</button>
<Popup show={show} anchor={document.getElementById('anchor')} animate={{ openDuration: 300, closeDuration: 300 }}>Animated Popup</Popup>
<div id="anchor" style={{ marginTop: '20px' }}>Anchor Element</div>
</div>
);
};
export default AnimatedPopup;
reactjs-popup is a simple and customizable popup component for React. It provides similar functionality to @progress/kendo-react-popup, including basic popups, custom positioning, and animations. However, it may not offer the same level of integration and customization options as the KendoReact suite.
react-modal is a popular package for creating modal dialogs in React. While it is primarily focused on modals, it can be used to create popup-like components. It offers a high level of customization and accessibility features, but it may require more configuration compared to @progress/kendo-react-popup.
react-tooltip is a lightweight package for creating tooltips in React. It provides functionality for displaying content in a popup-like manner, similar to @progress/kendo-react-popup. However, it is more specialized for tooltips and may not offer the same flexibility for general popup use cases.
This package is part of the Kendo UI for React suite.
This is commercial software. To use it, you need to agree to the Telerik End User License Agreement for Kendo UI Complete. If you do not own a commercial license, this file shall be governed by the trial license terms.
All available Kendo UI commercial licenses may be obtained at http://www.telerik.com/purchase/kendo-ui.
Copyright © 2018 Progress Software Corporation and/or its subsidiaries or affiliates. All Rights Reserved.
Progress, Telerik, and certain product names used herein are trademarks or registered trademarks of Progress Software Corporation and/or one of its subsidiaries or affiliates in the U.S. and/or other countries.
FAQs
React Popup positions a piece of content next to a specific anchor component. KendoReact Popup package
The npm package @progress/kendo-react-popup receives a total of 91,382 weekly downloads. As such, @progress/kendo-react-popup popularity was classified as popular.
We found that @progress/kendo-react-popup demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.