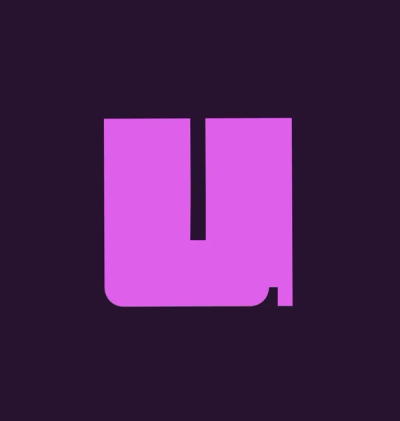
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@putout/engine-runner
Advanced tools
Run putout plugins.
npm i @putout/engine-runner
Replacer
converts code in declarative way. Simplest possible form, no need to use fix
. Just from
and to
parts.
Simplest replace example:
module.exports.report = () => 'any message here';
module.exports.replace = () => {
'const a = 1': 'const b = 1',
};
// optional
module.exports.filter = (path) => {
return true;
};
// optional
module.exports.match = () => ({
'const __a = 1': ({__a}) {
return true;
}
})
// optional
module.exports.exclude = () => [
`const hello = 'world'`,
];
Simplest remove example:
module.exports.report = () => 'debugger should not be used';
module.exports.replace = () => {
'debugger': '',
};
Templates:
module.exports.report = () => 'any message here';
module.exports.replace = () => {
'var __a = 1': 'const __a = 1',
};
A couple variables example:
module.exports.report = () => 'any message here';
module.exports.replace = () => {
'const __a = __b': 'const __b = __a',
};
You can pass a function as object value for more soficticated processing.
Remove node:
module.exports.report = () => 'any message here';
module.exports.replace = () => {
'for (const __a of __b) __c': ({__a, __b, __c}, path) => {
// remove node
return '';
},
};
Update node:
module.exports.report = () => 'any message here';
module.exports.replace = () => {
'for (const __a of __array) __c': ({__a, __array, __c}, path) => {
// update __array elements count
path.node.right.elements = [];
return path;
},
};
Update node using template variables:
module.exports.report = () => 'any message here';
module.exports.replace = () => {
'for (const __a of __array) __c': ({__a, __array, __c}, path) => {
// update the whole node using template string
return 'for (const x of y) z';
},
};
includer
is the most prefarable format of a plugin, simplest to use (after replacer
)
module.exports.report = () => 'debugger statement should not be used';
module.exports.fix = (path) => {
path.remove();
};
module.exports.include = () => [
'debugger',
];
// optional
module.exports.exclude = () => {
};
// optional
module.exports.filter = (path) => {
return true;
};
include
and exclude
returns an array of @babel/types, or code blocks:
const __ = 'hello';
Where __
can be any node. All this possible with help of @putout/compare. Templates format supported in traverse
and find
plugins as well.
Traverse plugins
gives you more power to filter
and fix
nodes you need.
module.exports.report = () => 'debugger statement should not be used';
module.exports.fix = (path) => {
path.remove();
};
module.exports.traverse = ({push}) => {
return {
'debugger'(path) {
push(path);
}
}
};
To keep things during traverse in a safe way listStore
can be used.
module.exports.traverse = ({push, listStore}) => {
return {
'debugger'(path) {
listStore('x');
push(path);
},
Program: {
exit: {
console.log(listStore());
// returns
['x', 'x', 'x']
// for code
'debugger; debugger; debugger'
}
}
}
};
store
is prefered way of keeping array elements, because of caching of putout
, traverse
init function called only once, and any other way
of handling variables will most likely will lead to bugs.
When you need key-value
storage store
can be used.
module.exports.traverse = ({push, store}) => {
return {
'debugger'(path) {
store('hello', 'world');
push(path);
},
Program: {
exit: {
store()
// returns
['world']
store.entries();
// [['hello', 'world']]
store('hello');
// 'world'
}
}
}
};
Find plugins
gives you all the control over traversing, but it's the slowest format.
Because traversers
not merged in contrast with other plugin formats.
module.exports.report = () => 'debugger statement should not be used';
module.exports.fix = (path) => {
path.remove();
};
module.exports.find = (ast, {push, traverse}) => {
traverse(ast, {
'debugger'(path) {
push(path);
}
}
};
const {runPlugins} = require('@putout/engine-runner');
const {parse} = require('@putout/engin-parser');
const plugins = [{
rule: "remove-debugger",
msg: "", // optional
options: {}, // optional
plugin:
include: () => ['debugger'],
fix: (path) => path.remove(),
report: () => `debugger should not be used`,
}];
const ast = parse('const m = "hi"; debugger');
const places = runPlugins({
ast,
shebang: false, // default
fix: true, // default
fixCount: 1 // default
plugins,
parser: 'babel', // default
});
To see logs, use:
DEBUG=putout:runner:*
DEBUG=putout:runner:fix
DEBUG=putout:runner:find
DEBUG=putout:runner:template
MIT
FAQs
Run 🐊Putout plugins
The npm package @putout/engine-runner receives a total of 6,104 weekly downloads. As such, @putout/engine-runner popularity was classified as popular.
We found that @putout/engine-runner demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.