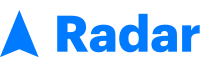

Radar is the location platform for mobile apps.
Installation
Install the Cordova plugin:
$ cordova plugin install cordova-plugin-radar
The Cordova plugin installs the Radar SDK using CocoaPods.
Before writing any JavaScript, you must integrate the Radar SDK with your iOS and Android apps by following the Configure project and Add SDK to project steps in the SDK documentation.
On iOS, you must add location usage descriptions and background modes to your Info.plist
. Initialize the SDK in application:didFinishLaunchingWithOptions:
in AppDelegate.m
, passing in your publishable API key.
#import <RadarSDK/RadarSDK.h>
[Radar initializeWithPublishableKey:publishableKey];
On Android, you must add the Google Play Services library to your project. Initialize the SDK in onCreate()
in MainApplication.java
, passing in your publishable API key:
import io.radar.sdk.Radar;
Radar.initialize(publishableKey);
Usage
Identify user
Until you identify the user, Radar will automatically identify the user by device ID.
To identify the user when logged in, call:
cordova.plugins.radar.setUserId(userId);
where userId
is a stable unique ID string for the user.
Do not send any PII, like names, email addresses, or publicly available IDs, for userId
. See privacy best practices for more information.
To set an optional description for the user, displayed in the dashboard, call:
cordova.plugins.radar.setDescription(description);
where description
is a string.
You only need to call these methods once, as these settings will be persisted across app sessions.
Request permissions
Before tracking the user's location, the user must have granted location permissions for the app.
To determine the whether user has granted location permissions for the app, call:
cordova.plugins.radar.getPermissionsStatus().then((status) => {
});
status
will be a string, one of:
To request location permissions for the app, call:
cordova.plugins.radar.requestPermissions(background);
where background
is a boolean indicating whether to request background location permissions or foreground location permissions. On Android, background
will be ignored.
Foreground tracking
Once you have initialized the SDK, you have identified the user, and the user has granted permissions, you can track the user's location.
To track the user's location in the foreground, call:
cordova.plugins.radar.trackOnce().then((result) => {
}).catch((err) => {
});
err
will be a string, one of:
ERROR_PUBLISHABLE_KEY
: the SDK was not initializedERROR_USER_ID
: the user was not identifiedERROR_PERMISSIONS
: the user has not granted location permissions for the appERROR_LOCATION
: location services were unavailable, or the location request timed outERROR_NETWORK
: the network was unavailable, or the network connection timed outERROR_UNAUTHORIZED
: the publishable API key is invalidERROR_SERVER
: an internal server error occurredERROR_UNKNOWN
: an unknown error occurred
Background tracking
Once you have initialized the SDK, you have identified the user, and the user has granted permissions, you can start tracking the user's location in the background.
To start tracking the user's location in the background, call:
cordova.plugins.radar.startTracking();
Assuming you have configured your project properly, the SDK will wake up while the user is moving (usually every 3-5 minutes), then shut down when the user stops (usually within 5-10 minutes). To save battery, the SDK will not wake up when stopped, and the user must move at least 100 meters from a stop (sometimes more) to wake up the SDK. Note that location updates may be delayed significantly by iOS Low Power Mode, by Android Doze Mode and App Standby and Background Location Limits, or if the device has connectivity issues, low battery, or wi-fi disabled. These constraints apply to all uses of background location services on iOS and Android, not just Radar. See more about accuracy and reliability.
To stop tracking the user's location in the background (e.g., when the user logs out), call:
cordova.plugins.radar.stopTracking();
You only need to call these methods once, as these settings will be persisted across app sessions.
To listen for events and errors, you can add event listeners:
cordova.plugins.radar.onEvents((events, user) => {
});
cordova.plugins.radar.onError((err) => {
});
You should remove event listeners when you are done with them:
cordova.plugins.radar.offEvents();
cordova.plugins.radar.offError();
Manual tracking
You can manually update the user's location by calling:
const location = {
latitude: 39.2904,
longitude: -76.6122,
accuracy: 65
};
cordova.plugins.radar.updateLocation(location).then((result) => {
}).catch((err) => {
});