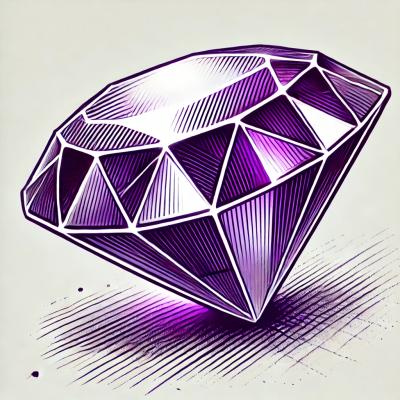
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@rapidui/quiver-http
Advanced tools
Methods:
| Name | Type | Description |
| --- | --- | 650--- |
| delete
| function(url: string, options: RequestOptionsArgs)
| Uses underlying @angular [http] to request a DELETE method to a URL, executing the interceptors as part of the request pipeline.
| get
| function(url: string, options: RequestOptionsArgs)
| Uses underlying @angular [http] to request a GET method to a URL, executing the interceptors as part of the request pipeline.
| head
| function(url: string, options: RequestOptionsArgs)
| Uses underlying @angular [http] to request a HEAD method to a URL, executing the interceptors as part of the request pipeline.
| patch
| function(url: string, data: any, options: RequestOptionsArgs)
| Uses underlying @angular [http] to request a PATCH method to a URL, executing the interceptors as part of the request pipeline.
| post
| function(url: string, data: any, options: RequestOptionsArgs)
| Uses underlying @angular [http] to request a POST method to a URL, executing the interceptors as part of the request pipeline.
| put
| function(url: string, data: any, options: RequestOptionsArgs)
| Uses underlying @angular [http] to request a PUT method to a URL, executing the interceptors as part of the request pipeline.
| request
| function(url: string | Request, options: RequestOptionsArgs)
| Uses underlying @angular [http] to request a generic request to a URL, executing the interceptors as part of the request pipeline.
Service provided with methods that wrap the @angular [Http] service and provide an easier experience for interceptor implementation.
To add a desired interceptor, it needs to implement the [IHttpInterceptor] interface.
export interface IHttpInterceptor {
onRequest?: (requestOptions: RequestOptionsArgs) => RequestOptionsArgs;
onRequestError?: (requestOptions: RequestOptionsArgs) => RequestOptionsArgs;
onResponse?: (response: Response) => Response;
onResponseError?: (error: Response) => Response;
}
Every method is optional, so you can just implement the ones that are needed.
Create your custom interceptors by implementing [IHttpInterceptor]:
import { Injectable } from '@angular/core';
import { IHttpInterceptor } from '@covalent/http';
@Injectable()
export class CustomInterceptor implements IHttpInterceptor {
onRequest(requestOptions: RequestOptionsArgs): RequestOptionsArgs {
... // do something to requestOptions before a request
... // if something is wrong, throw an error to execute onRequestError (if there is an onRequestError hook)
if (/*somethingWrong*/) {
throw new Error('error message for subscription error callback');
}
return requestOptions;
}
onRequestError(requestOptions: RequestOptionsArgs): RequestOptionsArgs {
... // do something to try and recover from an error thrown `onRequest`
... // and return the requestOptions needed for the request
... // else return 'undefined' or throw an error to execute the error callback of the subscription
if (cantRecover) {
throw new Error('error message for subscription error callback'); // or return undefined;
}
return requestOptions;
}
onResponse(response: Response): Response {
... // check response status and do something
return response;
}
onResponseError(error: Response): Response {
... // check error status and do something
return error;
}
}
Then, import the [QuiverHttpModule] using the forRoot() method with the desired interceptors and paths to intercept in your NgModule:
import { NgModule, Type } from '@angular/core';
import { HttpModule } from '@angular/http';
import { QuiverHttpModule, IHttpInterceptor } from '@covalent/http';
import { CustomInterceptor } from 'dir/to/interceptor';
const httpInterceptorProviders: Type<IHttpInterceptor>[] = [
CustomInterceptor,
...
];
@NgModule({
imports: [
HttpModule,
QuiverHttpModule.forRoot({
interceptors: [{
interceptor: CustomInterceptor, paths: ['**'],
}],
}),
...
],
providers: [
httpInterceptorProviders,
...
],
...
})
export class MyModule {}
After that, just inject [HttpInterceptorService] and use it for your requests.
The following characters are accepted as a path to intercept
**
is a wildcard for [a-zA-Z0-9-_]
(including /
)*
is a wildcard for [a-zA-Z0-9-_]
(excluding /
)[a-zA-Z0-9-_]
Example 1
/users/*/groups
intercepts:
www.url.com/users/id-of-user/groups
www.url.com/users/id/groups
/users/*/groups
DOES NOT intercept:
www.url.com/users/id-of-user/groups/path
www.url.com/users/id-of-user/path/groups
www.url.com/users/groups
Example 2
/users/**/groups
intercepts:
www.url.com/users/id-of-user/groups
www.url.com/users/id/groups
www.url.com/users/id-of-user/path/groups
/users/**/groups
DOES NOT intercept:
www.url.com/users/id-of-user/groups/path
www.url.com/users/groups
Example 3
/users/**
intercepts:
www.url.com/users/id-of-user/groups
www.url.com/users/id/groups
www.url.com/users/id-of-user/path/groups
www.url.com/users/id-of-user/groups/path
www.url.com/users/groups
/users/**
DOES NOT intercept:
www.url.com/users
Example 4
/users**
intercepts:
www.url.com/users/id-of-user/groups
www.url.com/users/id/groups
www.url.com/users/id-of-user/path/groups
www.url.com/users/id-of-user/groups/path
www.url.com/users/groups
www.url.com/users
Methods:
| Name | Type | Description |
| --- | --- | 650--- |
| query
| function(query?: IRestQuery, transform?: IRestTransform)
| Creates a GET request to the generated endpoint URL.
| get
| function(id: string | number, transform?: IRestTransform)
| Creates a GET request to the generated endpoint URL, adding the ID at the end.
| create
| function(obj: T, transform?: IRestTransform)
| Creates a POST request to the generated endpoint URL.
| update
| function(id: string | number, obj: T, transform?: IRestTransform)
| Creates a PATCH request to the generated endpoint URL, adding the ID at the end.
| delete
| function(id: string | number, transform?: IRestTransform)
| Creates a DELETE request to the generated endpoint URL, adding the ID at the end.
| buildUrl
| function(id?: string | number, query?: IRestQuery)
| Builds the endpoint URL with the configured properties and arguments passed in the method.
Abstract class provided with methods that wraps http services to facilitate REST API calls.
Example:
import { Injectable } from '@angular/core';
import { Response, Http, Headers } from '@angular/http';
import { RESTService, HttpInterceptorService } from '@covalent/http';
@Injectable()
export class CustomRESTService extends RESTService<any> {
constructor(private _http: Http /* or HttpInterceptorService */) {
super(_http, {
baseUrl: 'www.api.com',
path: '/path/to/endpoint',
headers: new Headers(),
dynamicHeaders: () => new Headers(),
transform: (res: Response): any => res.json(),
});
}
}
Note: the constructor takes any object that implements the methods in [IHttp] interface. This can be the @angular [Http] service, the covalent [HttpInterceptorService] or a custom service.
export interface IHttp {
delete: (url: string, options?: RequestOptionsArgs) => Observable<Response>;
get: (url: string, options?: RequestOptionsArgs) => Observable<Response>;
head: (url: string, options?: RequestOptionsArgs) => Observable<Response>;
patch: (url: string, body: any, options?: RequestOptionsArgs) => Observable<Response>;
post: (url: string, body: any, options?: RequestOptionsArgs) => Observable<Response>;
put: (url: string, body: any, options?: RequestOptionsArgs) => Observable<Response>;
request: (url: string | Request, options: RequestOptionsArgs) => Observable<Response>;
}
FAQs
Rapid UI HTTP Module
The npm package @rapidui/quiver-http receives a total of 0 weekly downloads. As such, @rapidui/quiver-http popularity was classified as not popular.
We found that @rapidui/quiver-http demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.