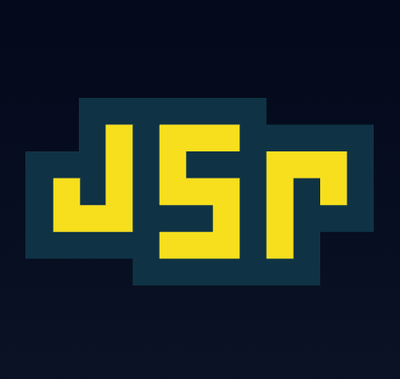
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@react-aria/textfield
Advanced tools
@react-aria/textfield is a React library that provides accessible text field components. It is part of the React Aria collection, which is designed to help developers build accessible web applications. The package offers a set of hooks and components that manage the state and behavior of text fields, ensuring they are accessible to users with disabilities.
Basic TextField
This code demonstrates how to create a basic accessible text field using the useTextField hook from @react-aria/textfield. The hook manages the state and behavior of the text field, ensuring it is accessible.
import { useTextField } from '@react-aria/textfield';
import { useRef } from 'react';
function TextField(props) {
let ref = useRef();
let { labelProps, inputProps } = useTextField(props, ref);
return (
<div>
<label {...labelProps}>{props.label}</label>
<input {...inputProps} ref={ref} />
</div>
);
}
export default function App() {
return <TextField label="Enter your name:" />;
}
TextField with Validation
This code demonstrates how to create a text field with validation using the useTextField hook. The text field checks if the input value is at least 5 characters long and displays an error message if it is not.
import { useTextField } from '@react-aria/textfield';
import { useRef, useState } from 'react';
function TextField(props) {
let ref = useRef();
let { labelProps, inputProps, errorMessageProps } = useTextField(props, ref);
let [value, setValue] = useState('');
let [error, setError] = useState(null);
function validate(value) {
if (value.length < 5) {
setError('Value must be at least 5 characters long');
} else {
setError(null);
}
}
return (
<div>
<label {...labelProps}>{props.label}</label>
<input
{...inputProps}
ref={ref}
value={value}
onChange={(e) => {
setValue(e.target.value);
validate(e.target.value);
}}
/>
{error && <span {...errorMessageProps}>{error}</span>}
</div>
);
}
export default function App() {
return <TextField label="Enter your name:" />;
}
TextField with Custom Styles
This code demonstrates how to create a text field with custom styles using the useTextField hook. The text field is styled with padding, border, and border-radius to enhance its appearance.
import { useTextField } from '@react-aria/textfield';
import { useRef } from 'react';
function TextField(props) {
let ref = useRef();
let { labelProps, inputProps } = useTextField(props, ref);
return (
<div style={{ margin: '20px 0' }}>
<label {...labelProps} style={{ display: 'block', marginBottom: '8px' }}>{props.label}</label>
<input {...inputProps} ref={ref} style={{ padding: '8px', border: '1px solid #ccc', borderRadius: '4px' }} />
</div>
);
}
export default function App() {
return <TextField label="Enter your name:" />;
}
react-hook-form is a library for managing form state and validation in React applications. It provides a set of hooks for handling form inputs, including text fields, and offers built-in validation and error handling. Compared to @react-aria/textfield, react-hook-form focuses more on form state management and validation rather than accessibility.
formik is a popular library for building and managing forms in React. It provides a set of components and hooks for handling form state, validation, and submission. While formik offers some accessibility features, it is not as focused on accessibility as @react-aria/textfield, which is specifically designed to ensure accessible text fields.
react-final-form is a library for managing form state in React applications. It provides a set of components and hooks for handling form inputs, validation, and submission. Like formik and react-hook-form, react-final-form focuses more on form state management and validation, whereas @react-aria/textfield is specifically designed for accessibility.
This package is part of react-spectrum. See the repo for more details.
FAQs
Spectrum UI components in React
We found that @react-aria/textfield demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.