What is @react-email/render?
@react-email/render is a package designed to help developers render React components to HTML for email templates. It simplifies the process of creating and managing email templates using React, allowing for dynamic and reusable components.
What are @react-email/render's main functionalities?
Render React Components to HTML
This feature allows you to render React components to HTML, which can then be used as the content of an email. The code sample demonstrates rendering a simple React component to HTML.
const { render } = require('@react-email/render');
const React = require('react');
const MyEmail = () => (
<div>
<h1>Hello, World!</h1>
<p>This is a test email.</p>
</div>
);
const html = render(<MyEmail />);
console.log(html);
Support for JSX
This feature supports JSX syntax, making it easier to write and manage email templates. The code sample shows a React component using JSX syntax.
const { render } = require('@react-email/render');
const React = require('react');
const MyEmail = () => (
<div>
<h1>Hello, World!</h1>
<p>This is a test email with <strong>JSX</strong> support.</p>
</div>
);
const html = render(<MyEmail />);
console.log(html);
Dynamic Content
This feature allows for dynamic content in email templates by passing props to React components. The code sample demonstrates rendering a personalized email with a dynamic name.
const { render } = require('@react-email/render');
const React = require('react');
const MyEmail = ({ name }) => (
<div>
<h1>Hello, {name}!</h1>
<p>This is a personalized email.</p>
</div>
);
const html = render(<MyEmail name="John" />);
console.log(html);
Other packages similar to @react-email/render
react-html-email
react-html-email is a package that provides tools for building and sending HTML emails using React components. It offers similar functionality to @react-email/render, including the ability to create reusable components and render them to HTML. However, it also includes additional features like CSS inlining and email validation.
mjml-react
mjml-react is a package that allows developers to use MJML (a markup language designed for responsive email) with React components. It provides a higher-level abstraction for creating responsive email templates, which can be more convenient for complex layouts compared to @react-email/render.
react-email-components
react-email-components is a library of pre-built React components specifically designed for email templates. It simplifies the process of creating email layouts by providing a set of common components like buttons, headers, and footers. While @react-email/render focuses on rendering any React component to HTML, react-email-components provides ready-to-use components for common email elements.
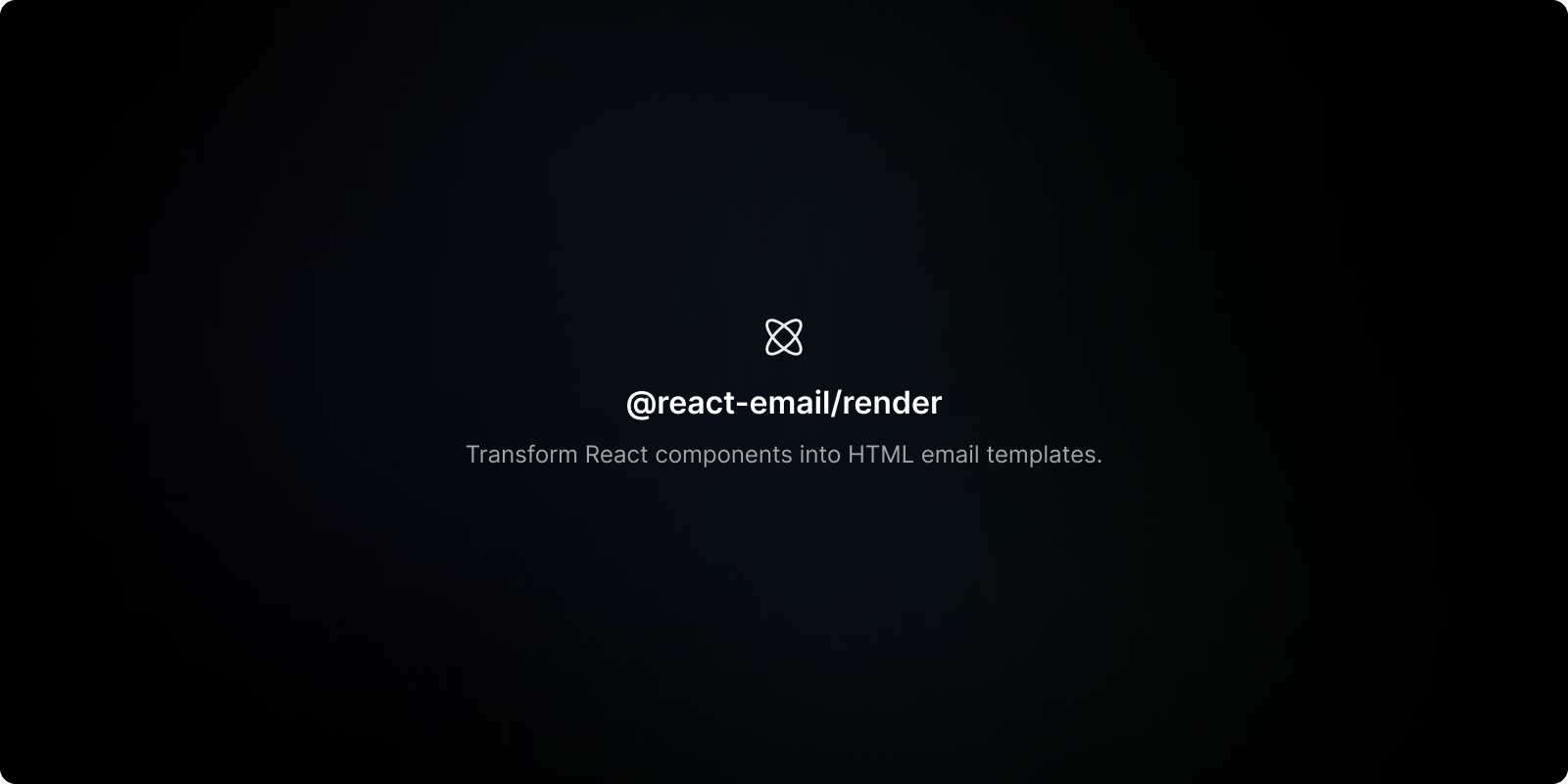
@react-email/render
Transform React components into HTML email templates.
Install
Install component from your command line.
With yarn
yarn add @react-email/render -E
With npm
npm install @react-email/render -E
Getting started
Convert React components into a HTML string.
import { MyTemplate } from "../components/MyTemplate";
import { render } from "@react-email/render";
const html = render(<MyTemplate firstName="Jim" />);
License
MIT License