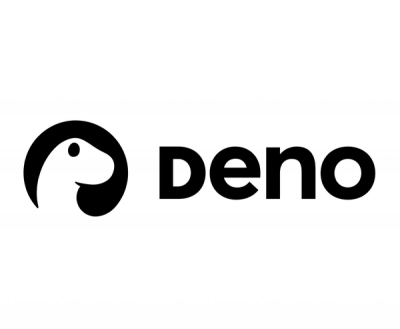
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@react-md/menu
Advanced tools
Create menus that auto-position themselves within the viewport and adhere to the accessibility guidelines
Create accessible dropdown menus that auto-position themselves to stay within the viewport. The menus are entirely navigable with a keyboard along with some additional behavior:
ArrowUp
and ArrowDown
to focus the previous/next MenuItem
that also
allows wrappingHome
and End
to focus the first/last Menuitem
in the menuMenuItem
to focus itMore information on the built-in accessibility can be found in the accessibility example on the documentation site.
npm install --save @react-md/menu
You will also need to install the following packages for their styles:
npm install --save @react-md/theme \
@react-md/typography \
@react-md/icon \
@react-md/list \
@react-md/button \
@react-md/states \
@react-md/utils
You should check out the full documentation for live examples and more customization information, but an example usage is shown below.
import { render } from "react-dom";
import { DropdownMenu } from "@react-md/menu";
const App = () => (
<DropdownMenu
id="example-dropdown-menu"
items={[
{ onClick: () => console.log("Clicked Item 1"), children: "Item 1" },
{ onClick: () => console.log("Clicked Item 2"), children: "Item 2" },
{ onClick: () => console.log("Clicked Item 3"), children: "Item 3" },
]}
>
Dropdown
</DropdownMenu>
);
render(<App />, document.getElementById("root"));
FAQs
Create menus that auto-position themselves within the viewport and adhere to the accessibility guidelines
We found that @react-md/menu demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.