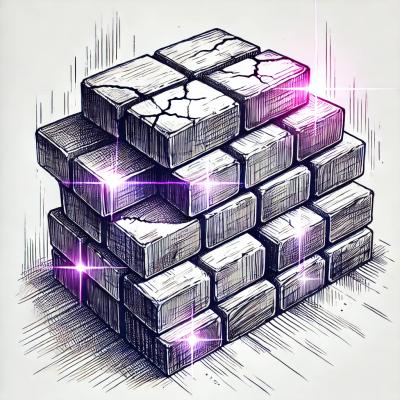
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
@react-native-community/async-storage
Advanced tools
Main, public-facing components of Async Storage. The core module contains a factory to create an `AsyncStorage` instance and `IStorageBackend`, that needs to be implemented by any Backend Storage in order to be compatible.
@react-native-community/async-storage is an asynchronous, unencrypted, persistent, key-value storage system for React Native. It is designed to store small amounts of data, such as user preferences, app settings, or other lightweight data that needs to persist across app sessions.
Store Data
This feature allows you to store a key-value pair in the storage. The `setItem` method is used to save the data asynchronously.
async function storeData(key, value) {
try {
await AsyncStorage.setItem(key, value);
} catch (e) {
// saving error
console.error(e);
}
}
Retrieve Data
This feature allows you to retrieve a value by its key from the storage. The `getItem` method is used to fetch the data asynchronously.
async function retrieveData(key) {
try {
const value = await AsyncStorage.getItem(key);
if (value !== null) {
// value previously stored
return value;
}
} catch (e) {
// error reading value
console.error(e);
}
}
Remove Data
This feature allows you to remove a key-value pair from the storage. The `removeItem` method is used to delete the data asynchronously.
async function removeData(key) {
try {
await AsyncStorage.removeItem(key);
} catch (e) {
// remove error
console.error(e);
}
}
Merge Data
This feature allows you to merge an existing value with a new value for a given key. The `mergeItem` method is used to update the data asynchronously.
async function mergeData(key, value) {
try {
await AsyncStorage.mergeItem(key, value);
} catch (e) {
// merge error
console.error(e);
}
}
Clear All Data
This feature allows you to clear all key-value pairs from the storage. The `clear` method is used to remove all data asynchronously.
async function clearAllData() {
try {
await AsyncStorage.clear();
} catch (e) {
// clear error
console.error(e);
}
}
react-native-mmkv is a fast, small, and easy-to-use key-value storage library for React Native. It is based on Facebook's MMKV storage library and offers better performance and lower memory usage compared to @react-native-community/async-storage.
react-native-encrypted-storage provides a secure and encrypted storage solution for React Native applications. It uses the device's secure storage mechanisms to store data, making it more secure than @react-native-community/async-storage, which does not encrypt data.
redux-persist is a library that allows you to persist and rehydrate a Redux store. It can be used with various storage backends, including AsyncStorage. It is more suitable for applications that use Redux for state management and need to persist the entire state.
Main, public-facing components of Async Storage. The core module contains a factory to create an AsyncStorage
instance
and IStorageBackend
, that needs to be implemented by any Backend Storage in order to be compatible.
$ yarn install @react-native-community/async-storage
AsyncStorageFactory
A factory module for AsyncStorage
instance with selected storage backend attached.
// storage.js
import ASFactory from '@react-native-community/async-storage'
// use any available Storage Backend
const storageBackend = new StorageBackend();
const mobileStorage = ASFactory.create(storageBackend, options);
export default mobileStorage;
Factory options
AsyncStorageFactory.create
accepts an options object, that enables additional features.
type logger = ((action: LoggerAction) => void) | boolean;
Used to log AsyncStorage
method calls and used arguments.
You can provide your own implementation or use provided default logger (enabled by default in DEV mode).
type errorHandler = ((error: Error | string) => void) | boolean;
Used to report any errors thrown. You can provide your own implementation or use provided default error handler (enabled by default in DEV mode).
Providing a storage model
If you know the structure of the stored data upfront, you can use the full potential of the type system, by providing a type argument to AsyncStorageFactory.create<T>
method.
import ASFactory from '@react-native-community/async-storage'
type StorageModel = {
user: {
name: string;
age: number;
};
preferences: {
darkModeEnabled: boolean;
};
subscribedChannels: Array<string>;
onboardingCompleted: boolean;
};
// use any available Storage Backend
const storageBackend = new StorageBackend();
const storage = ASFactory.create<StorageModel>(storageBackend);
IStorageBackend
In order to let AsyncStorage
use a storage backend, it has to implement this interface.
Contains methods to get, set and remove data, return used keys or drop the whole storage.
import {
IStorageBackend,
} from '@react-native-community/async-storage';
type Model = {
count: number
user: {
name: string,
rating: number
}
}
class MyStorageSolution implements IStorageBackend<Model> {
// implement necessary methods
}
MIT
FAQs
Asynchronous, persistent, key-value storage system for React Native.
The npm package @react-native-community/async-storage receives a total of 108,376 weekly downloads. As such, @react-native-community/async-storage popularity was classified as popular.
We found that @react-native-community/async-storage demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.