What is @react-native-community/slider?
@react-native-community/slider is a React Native component for creating sliders, which are UI elements that allow users to select a value from a range by moving a thumb along a track. This package is useful for scenarios where you need to capture a range of values, such as volume control, brightness adjustment, or any other numeric input.
What are @react-native-community/slider's main functionalities?
Basic Slider
This code demonstrates a basic slider with a minimum value of 0 and a maximum value of 1. The slider has custom colors for the minimum and maximum track.
import Slider from '@react-native-community/slider';
import React from 'react';
import { View } from 'react-native';
const BasicSlider = () => {
return (
<View>
<Slider
style={{width: 200, height: 40}}
minimumValue={0}
maximumValue={1}
minimumTrackTintColor="#FFFFFF"
maximumTrackTintColor="#000000"
/>
</View>
);
};
export default BasicSlider;
Slider with Custom Thumb
This code demonstrates a slider with a custom thumb image. The thumbImage prop is used to set a custom image for the slider's thumb.
import Slider from '@react-native-community/slider';
import React from 'react';
import { View, Image } from 'react-native';
const CustomThumbSlider = () => {
return (
<View>
<Slider
style={{width: 200, height: 40}}
minimumValue={0}
maximumValue={100}
thumbImage={require('./path/to/thumbImage.png')}
/>
</View>
);
};
export default CustomThumbSlider;
Slider with Step Value
This code demonstrates a slider with a step value of 1. The step prop ensures that the slider's thumb moves in increments of 1.
import Slider from '@react-native-community/slider';
import React from 'react';
import { View } from 'react-native';
const StepSlider = () => {
return (
<View>
<Slider
style={{width: 200, height: 40}}
minimumValue={0}
maximumValue={10}
step={1}
/>
</View>
);
};
export default StepSlider;
Other packages similar to @react-native-community/slider
react-native-slider
react-native-slider is another popular package for creating sliders in React Native. It offers similar functionality to @react-native-community/slider, including support for custom thumb images, track colors, and step values. However, @react-native-community/slider is more actively maintained and is part of the React Native Community, which may offer better support and integration with other community packages.
react-native-multi-slider
react-native-multi-slider is a package that allows for the creation of multi-point sliders, where users can select multiple values along a range. This package is useful for more complex scenarios where a single slider is not sufficient. It offers more advanced features compared to @react-native-community/slider, but may be overkill for simpler use cases.

React Native component used to select a single value from a range of values.
Getting started
yarn add @react-native-community/slider
or
npm install @react-native-community/slider --save
Mostly automatic installation
react-native link @react-native-community/slider
Manual installation
Manually link the library on iOS
Open project.xcodeproj in Xcode
Drag RNCSlider.xcodeproj
to your project on Xcode (usually under the Libraries group on Xcode):
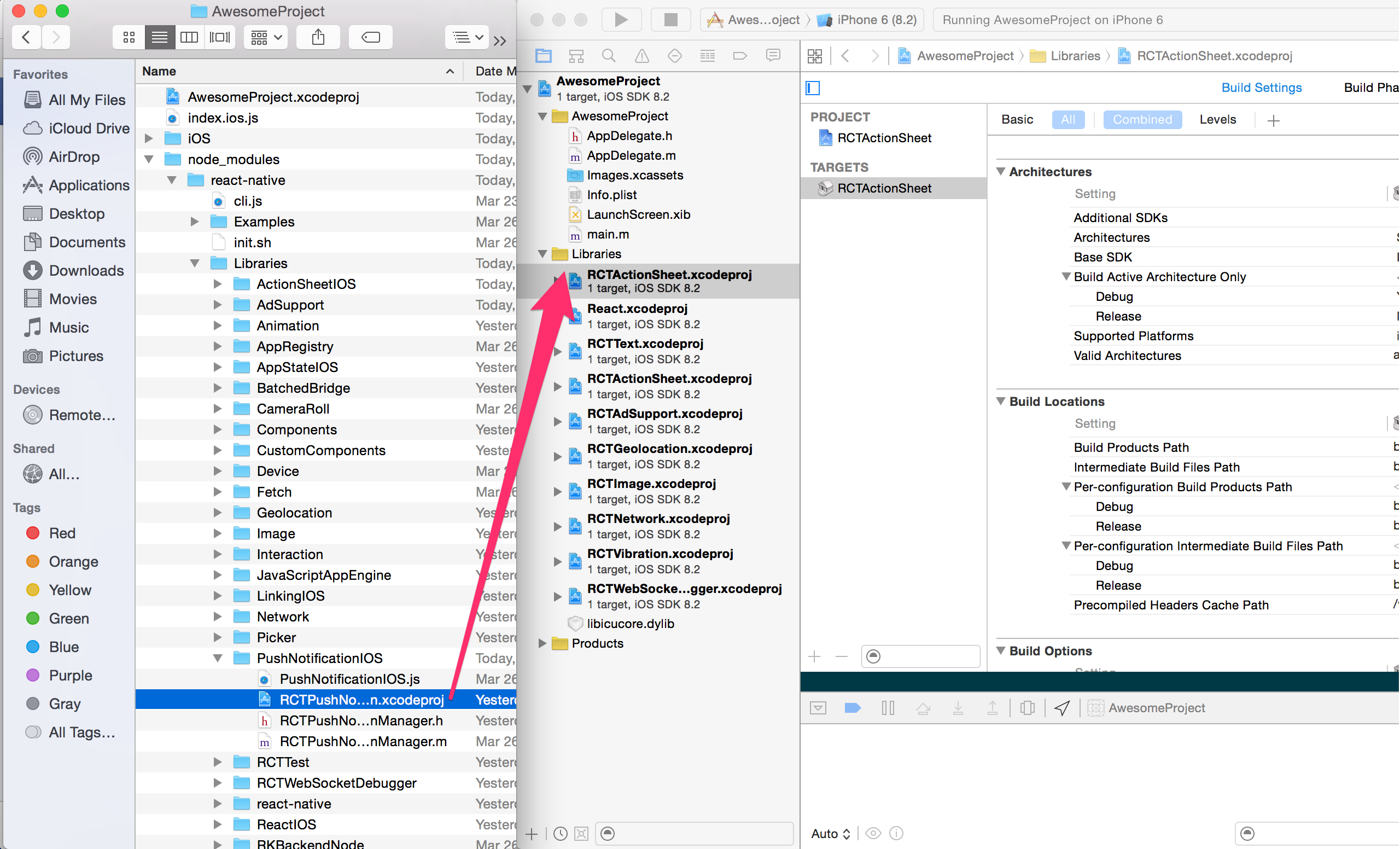
Link libRNCSlider.a
binary with libraries
Click on your main project file (the one that represents the .xcodeproj
) select Build Phases
and drag the static library from the Products
folder inside the Library you are importing to Link Binary With Libraries
(or use the +
sign and choose library from the list):
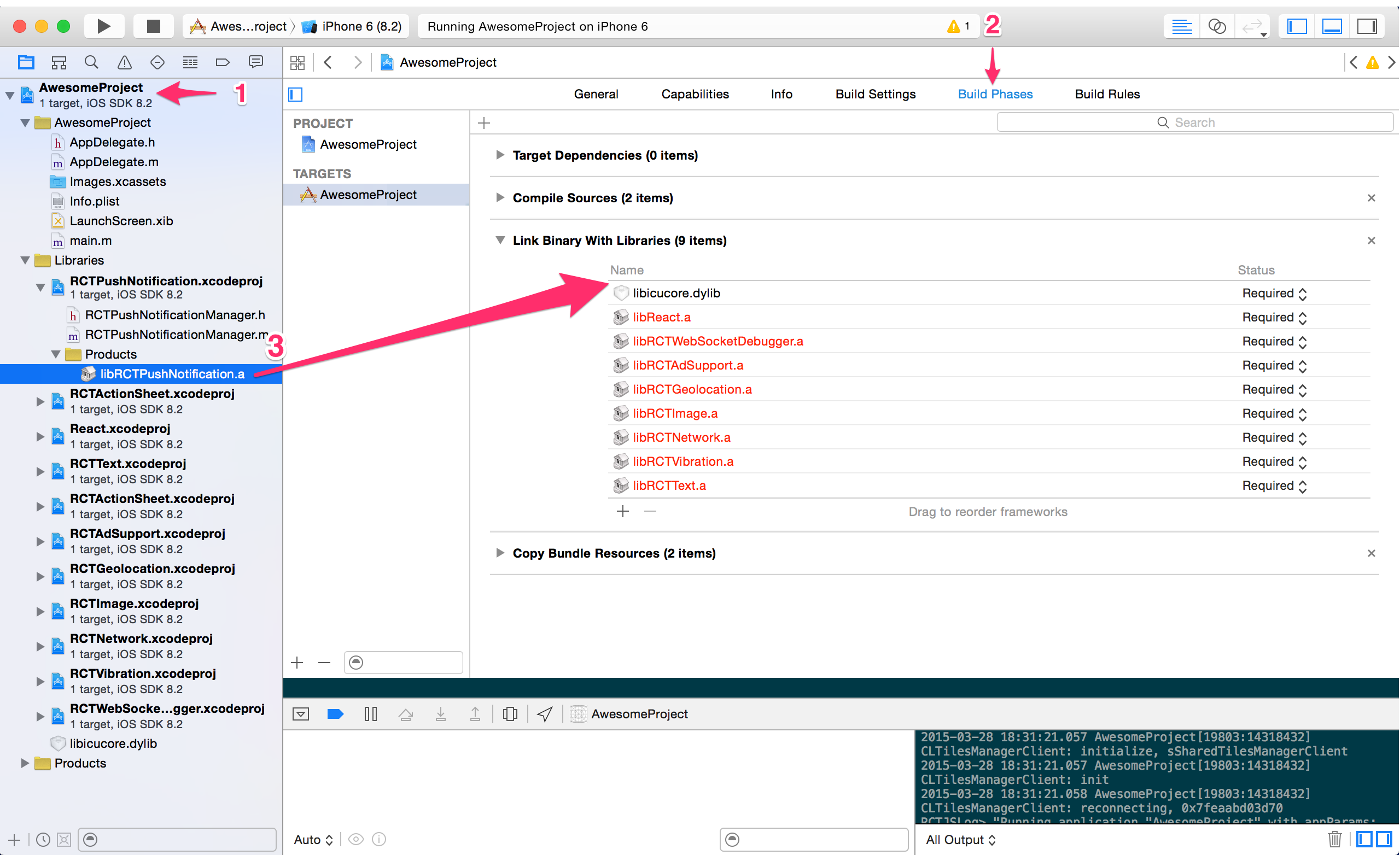
Manually link the library on Android
android/settings.gradle
include ':@react-native-community/slider'
project(':@react-native-community/slider').projectDir = new File(rootProject.projectDir, '../node_modules/@react-native-community/slider/android')
android/app/build.gradle
dependencies {
...
implementation project(':@react-native-community/slider')
}
android/app/src/main/.../MainApplication.java
On top, where imports are:
import com.reactnativecommunity.netinfo.ReactSliderPackage;
Add the ReactSliderPackage
class to your list of exported packages.
@Override
protected List<ReactPackage> getPackages() {
return Arrays.asList(
new MainReactPackage(),
new ReactSliderPackage()
);
}
Migrating from the core react-native
module
This module was created when the Slider was split out from the core of React Native. To migrate to this module you need to follow the installation instructions above and then change you imports from:
import { Slider } from 'react-native';
to:
import Slider from '@react-native-community/slider';
Usage
Example
import Slider from '@react-native-community/slider';
<Slider
style={{width: 200, height: 40}}
minimumValue={0}
maximumValue={1}
minimumTrackTintColor="#FFFFFF"
maximumTrackTintColor="#000000"
thumbTouchSize={{width: 50, height: 40}}
/>
Check out the example project for more examples.
Props
style
Used to style and layout the Slider
. See StyleSheet.js
and ViewStylePropTypes.js
for more info.
disabled
If true the user won't be able to move the slider. Default value is false.
maximumValue
Initial maximum value of the slider. Default value is 1.
minimumTrackTintColor
The color used for the track to the left of the button. Overrides the default blue gradient image on iOS.
minimumValue
Initial minimum value of the slider. Default value is 0.
onSlidingComplete
Callback that is called when the user releases the slider, regardless if the value has changed. The current value is passed as an argument to the callback handler.
onValueChange
Callback continuously called while the user is dragging the slider.
step
Step value of the slider. The value should be between 0 and (maximumValue - minimumValue). Default value is 0.
maximumTrackTintColor
The color used for the track to the right of the button. Overrides the default gray gradient image on iOS.
testID
Used to locate this view in UI automation tests.
value
Initial value of the slider. The value should be between minimumValue and maximumValue, which default to 0 and 1 respectively. Default value is 0.
This is not a controlled component, you don't need to update the value during dragging.
thumbTintColor
Color of the foreground switch grip.
Type | Required | Platform |
---|
color | No | Android |
maximumTrackImage
Assigns a maximum track image. Only static images are supported. The leftmost pixel of the image will be stretched to fill the track.
Type | Required | Platform |
---|
Image.propTypes.source | No | iOS |
minimumTrackImage
Assigns a minimum track image. Only static images are supported. The rightmost pixel of the image will be stretched to fill the track.
Type | Required | Platform |
---|
Image.propTypes.source | No | iOS |
thumbImage
Sets an image for the thumb. Only static images are supported.
Type | Required | Platform |
---|
Image.propTypes.source | No | iOS |
trackImage
Assigns a single image for the track. Only static images are supported. The center pixel of the image will be stretched to fill the track.
Type | Required | Platform |
---|
Image.propTypes.source | No | iOS |
Contributors
This module was extracted from react-native
core. Please reffer to https://github.com/react-native-community/react-native-slider/graphs/contributors for the complete list of contributors.
License
The library is released under the MIT licence. For more information see LICENSE
.