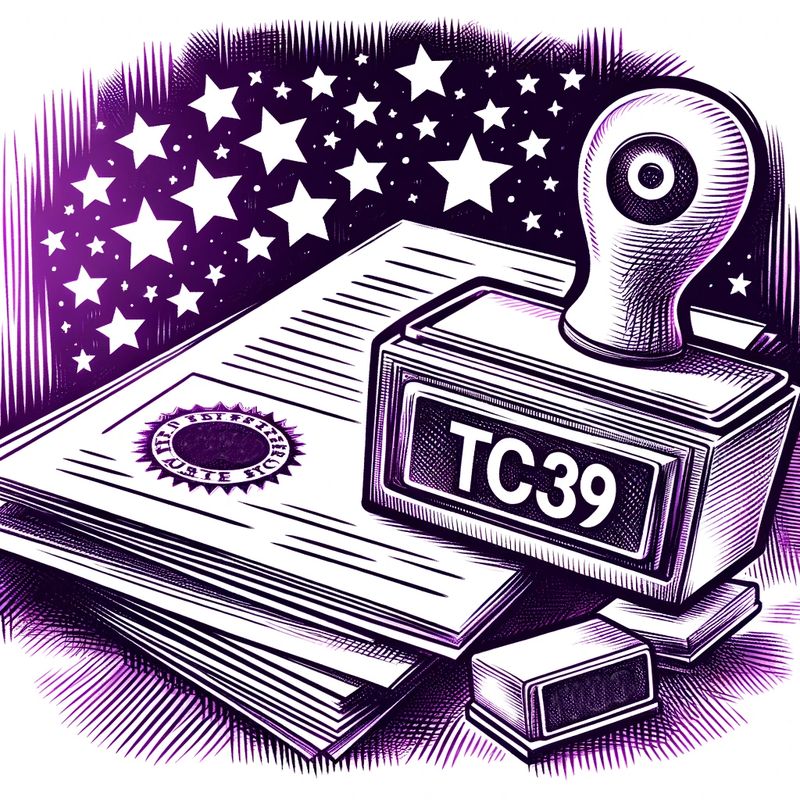
Security News
TC39 Advances Key Proposals: Deferred Import Evaluation, Error.isError(), RegExp Escaping, Promise.try
Ecma TC39 is meeting this week and has moved key ECMAScript proposals forward, advancing Deferred Import Evaluation, Error.isError(), RegExp Escaping, and Promise.try to the next stages.