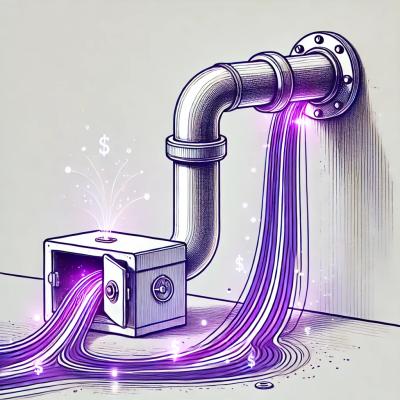
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@remix-run/server-runtime
Advanced tools
@remix-run/server-runtime is a package that provides server-side utilities and abstractions for building web applications with Remix. It allows developers to handle server-side rendering, data loading, and other server-side logic in a structured and efficient manner.
Server-Side Rendering
This feature allows you to handle server-side rendering with Remix. The `createRequestHandler` function is used to create a request handler that can be integrated with an Express server.
const { createRequestHandler } = require('@remix-run/server-runtime');
const express = require('express');
const app = express();
app.all('*', createRequestHandler({
getLoadContext() {
// Provide context for loaders
return {};
}
}));
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Data Loading
This feature allows you to load data on the server side before rendering a page. The `json` function is used to return JSON responses from loaders.
const { json } = require('@remix-run/server-runtime');
async function loader({ request }) {
const data = await fetchDataFromAPI();
return json(data);
}
Error Handling
This feature allows you to handle errors in your server-side logic. The `json` function can be used to return error responses with appropriate status codes.
const { json } = require('@remix-run/server-runtime');
async function loader({ request }) {
try {
const data = await fetchDataFromAPI();
return json(data);
} catch (error) {
return json({ error: error.message }, { status: 500 });
}
}
Next.js is a popular React framework that provides server-side rendering, static site generation, and API routes. It offers a more opinionated and integrated approach compared to @remix-run/server-runtime, which is more focused on providing utilities for server-side logic in Remix applications.
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. While it does not provide server-side rendering out of the box, it can be used in conjunction with other libraries to achieve similar functionality to @remix-run/server-runtime.
Koa is a new web framework designed by the team behind Express, which aims to be a smaller, more expressive, and more robust foundation for web applications and APIs. Like Express, it does not provide server-side rendering out of the box but can be extended with middleware to achieve similar functionality.
Remix supports multiple server runtimes:
Support for each runtime is provided by a corresponding Remix package:
This package defines a "Remix server runtime interface" that each runtime package must conform to.
Each Remix server runtime package MUST:
interface.ts
reexport.ts
Each Remix server runtime package MAY:
v2.6.0
Date: 2024-02-01
As we continue moving towards stabilizing the Vite plugin, we've introduced a few breaking changes to the unstable Vite plugin in this release. Please read the @remix-run/dev
changes below closely and update your app accordingly if you've opted into using the Vite plugin.
We've also removed the unstable_
prefix from the serverBundles
option as we're now confident in the API (#8596).
🎉 And last, but certainly not least - we've added much anticipated Cloudflare support in #8531! To get started with Cloudflare, you can use the unstable-vite-cloudflare
template:
npx create-remix@latest --template remix-run/remix/templates/unstable-vite-cloudflare
For more information, please refer to the docs at Future > Vite > Cloudflare and Future > Vite > Migrating > Migrating Cloudflare Functions.
@remix-run/server-runtime
- Add future.v3_throwAbortReason
flag to throw request.signal.reason
when a request is aborted instead of an Error
such as new Error("query() call aborted: GET /path")
(#8251)@remix-run/server-runtime
- Unwrap thrown Response
's from entry.server
into ErrorResponse
's and preserve the status code (#8577)
@remix-run/dev
- Vite: Add manifest
option to Vite plugin to enable writing a .remix/manifest.json
file to the build directory (#8575)
build/server/bundles.json
file has been superseded by the more general build/.remix/manifest.json
manifest
option@remix-run/dev
- Vite: Rely on Vite plugin ordering (#8627)
⚠️ This is a breaking change for projects using the unstable Vite plugin
The Remix plugin expects to process JavaScript or TypeScript files, so any transpilation from other languages must be done first.
For example, that means putting the MDX plugin before the Remix plugin:
import mdx from "@mdx-js/rollup";
import { unstable_vitePlugin as remix } from "@remix-run/dev";
import { defineConfig } from "vite";
export default defineConfig({
plugins: [
+ mdx(),
remix()
- mdx(),
],
});
Previously, the Remix plugin misused enforce: "post"
from Vite's plugin API to ensure that it ran last
However, this caused other unforeseen issues
Instead, we now rely on standard Vite semantics for plugin ordering
The official Vite React SWC plugin also relies on plugin ordering for MDX
@remix-run/dev
- Vite: Remove interop with <LiveReload />
, rely on <Scripts />
instead (#8636)
⚠️ This is a breaking change for projects using the unstable Vite plugin
Vite provides a robust client-side runtime for development features like HMR, making the <LiveReload />
component obsolete
In fact, having a separate dev scripts component was causing issues with script execution order
To work around this, the Remix Vite plugin used to override <LiveReload />
into a bespoke implementation that was compatible with Vite
Instead of all this indirection, now the Remix Vite plugin instructs the <Scripts />
component to automatically include Vite's client-side runtime and other dev-only scripts
To adopt this change, you can remove the LiveReload component from your root.tsx
component:
import {
- LiveReload,
Outlet,
Scripts,
}
export default function App() {
return (
<html>
<head>
</head>
<body>
<Outlet />
<Scripts />
- <LiveReload />
</body>
</html>
)
}
@remix-run/dev
- Vite: Only write Vite manifest files if build.manifest
is enabled within the Vite config (#8599)
⚠️ This is a breaking change for consumers of Vite's manifest.json
files
To explicitly enable generation of Vite manifest files, you must set build.manifest
to true
in your Vite config:
export default defineConfig({
build: { manifest: true },
// ...
});
@remix-run/dev
- Vite: Add new buildDirectory
option with a default value of "build"
(#8575)
⚠️ This is a breaking change for consumers of the Vite plugin that were using the assetsBuildDirectory
and serverBuildDirectory
options
This replaces the old assetsBuildDirectory
and serverBuildDirectory
options which defaulted to "build/client"
and "build/server"
respectively
The Remix Vite plugin now builds into a single directory containing client
and server
directories
If you've customized your build output directories, you'll need to migrate to the new buildDirectory
option, e.g.:
import { unstable_vitePlugin as remix } from "@remix-run/dev";
import { defineConfig } from "vite";
export default defineConfig({
plugins: [
remix({
- serverBuildDirectory: "dist/server",
- assetsBuildDirectory: "dist/client",
+ buildDirectory: "dist",
})
],
});
@remix-run/dev
- Vite: Write Vite manifest files to build/.vite
directory rather than being nested within build/client
and build/server
directories (#8599)
manifest.json
filesmanifest.json
build/.vite/client-manifest.json
and build/.vite/server-manifest.json
, or build/.vite/server-{BUNDLE_ID}-manifest.json
when using server bundles@remix-run/dev
- Vite: Remove unstable
prefix from serverBundles
option (#8596)
@remix-run/dev
- Vite: Add --sourcemapClient
and --sourcemapServer
flags to remix vite:build
(#8613)
--sourcemapClient
, --sourcemapClient=inline
, or --sourcemapClient=hidden
--sourcemapServer
, --sourcemapServer=inline
, or --sourcemapServer=hidden
@remix-run/dev
- Vite: Validate IDs returned from the serverBundles
function to ensure they only contain alphanumeric characters, hyphens and underscores (#8598)
@remix-run/dev
- Vite: Fix "could not fast refresh" false alarm (#8580)
meta
for fast refresh, which removes the false alarm.@remix-run/dev
- Vite: Cloudflare Pages support (#8531)
@remix-run/dev
- Vite: Add getRemixDevLoadContext
option to Cloudflare preset (#8649)
@remix-run/dev
- Vite: Remove undocumented backwards compatibility layer for Vite v4 (#8581)
@remix-run/dev
- Vite: Add presets
option to ease integration with different platforms and tools (#8514)
@remix-run/dev
- Vite: Add buildEnd
hook (#8620)
@remix-run/dev
- Vite: Add mode
field into generated server build (#8539)
@remix-run/dev
- Vite: Reduce network calls for route modules during HMR (#8591)
@remix-run/dev
- Vite: Export Unstable_ServerBundlesFunction
and Unstable_VitePluginConfig
types (#8654)
create-remix
@remix-run/architect
@remix-run/cloudflare
@remix-run/cloudflare-pages
@remix-run/cloudflare-workers
@remix-run/css-bundle
@remix-run/deno
@remix-run/dev
@remix-run/eslint-config
@remix-run/express
@remix-run/node
@remix-run/react
@remix-run/serve
@remix-run/server-runtime
@remix-run/testing
Full Changelog: v2.5.1...v2.6.0
FAQs
Server runtime for Remix
The npm package @remix-run/server-runtime receives a total of 807,253 weekly downloads. As such, @remix-run/server-runtime popularity was classified as popular.
We found that @remix-run/server-runtime demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.