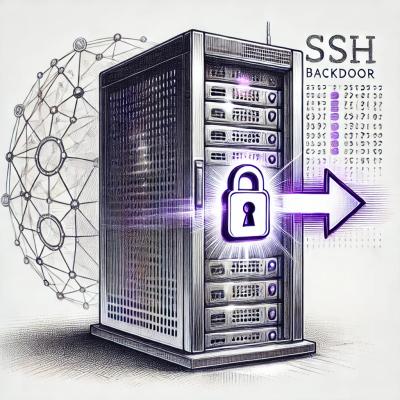
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@rodewitsch/carbone
Advanced tools
Fast, Simple and Powerful report generator. Injects JSON and produces PDF, DOCX, XLSX, ODT, PPTX, ODS, ...!
This is a fork of the carbone module. Added support for images, improved work with tables, formulas, graphs.
Fast, Simple and Powerful report generator in any format PDF, DOCX, XLSX, ODT, PPTX, ODS, XML, CSV...
... using your JSON data as input !
{d.companyName}
directly in your documentCarbone is a mustache-like template engine {d.companyName}
.
Template language documentation : https://carbone.io/documentation.html
Carbone analyzes your template and inject data in the document. The generated document can be exported as is, or converted to another format (PDF, ...) using LibreOffice if it is installed on the system. Carbone is working only on the server-side.
1 - Install it
npm install carbone
2 - Copy-paste this code in a new JS file, and execute it with node
const fs = require('fs');
const carbone = require('carbone');
// Data to inject
var data = {
firstname : 'John',
lastname : 'Doe'
};
// Generate a report using the sample template provided by carbone module
// This LibreOffice template contains "Hello {d.firstname} {d.lastname} !"
// Of course, you can create your own templates!
carbone.render('./node_modules/carbone/examples/simple.odt', data, function(err, result){
if (err) {
return console.log(err);
}
// write the result
fs.writeFileSync('result.odt', result);
});
Carbone uses efficiently LibreOffice to convert documents. Among all tested solutions, it is the most reliable and stable one in production for now.
Carbone does a lot of thing for you behind the scene:
Be careful, LibreOffice which is provided by the PPA libreoffice/ppa does not bundled python (mandatory for Carbone). The best solution is to download the LibreOffice Package from the official website and install it manually:
# remove all old version of LibreOffice
sudo apt remove --purge libreoffice*
sudo apt autoremove --purge
# Download LibreOffice debian package. Select the right one (64-bit or 32-bit) for your OS.
# Get the latest from http://download.documentfoundation.org/libreoffice/stable
# or download the version currently "carbone-tested":
wget https://downloadarchive.documentfoundation.org/libreoffice/old/5.3.2.2/deb/x86_64/LibreOffice_5.3.2.2_Linux_x86-64_deb.tar.gz
# Install required dependencies on ubuntu server for LibreOffice 5.0+
sudo apt install libxinerama1 libfontconfig1 libdbus-glib-1-2 libcairo2 libcups2 libglu1-mesa libsm6
# Uncompress package
tar -zxvf LibreOffice_5.3.2.2_Linux_x86-64_deb.tar.gz
cd LibreOffice_5.3.2.2_Linux_x86-64_deb/DEBS
# Install LibreOffice
sudo dpkg -i *.deb
# If you want to use Microsoft fonts in reports, you must install the fonts
# Andale Mono, Arial Black, Arial, Comic Sans MS, Courier New, Georgia, Impact,
# Times New Roman, Trebuchet, Verdana,Webdings)
sudo apt install ttf-mscorefonts-installer
# If you want to use special characters, such as chinese ideograms, you must install a font that support them
# For example:
sudo apt install fonts-wqy-zenhei
And now, you can use the converter, by passing options to render method.
Don't panic, only the first conversion is slow because LibreOffice must starts Once started, LibreOffice stays on to make new conversions faster
var data = {
firstname : 'John',
lastname : 'Doe'
};
var options = {
convertTo : 'pdf' //can be docx, txt, ...
};
carbone.render('./node_modules/carbone/examples/simple.odt', data, options, function(err, result){
if (err) return console.log(err);
fs.writeFileSync('result.pdf', result);
process.exit(); // to kill automatically LibreOffice workers
});
var data = [
{
movieName : 'Matrix',
actors : [{
firstname : 'Keanu',
lastname : 'Reeves'
},{
firstname : 'Laurence',
lastname : 'Fishburne'
},{
firstname : 'Carrie-Anne',
lastname : 'Moss'
}]
},
{
movieName : 'Back To The Future',
actors : [{
firstname : 'Michael',
lastname : 'J. Fox'
},{
firstname : 'Christopher',
lastname : 'Lloyd'
}]
}
];
carbone.render('./node_modules/carbone/examples/movies.docx', data, function(err, result){
if (err) return console.log(err);
fs.writeFileSync('movies_result.docx', result);
});
carbone.render('./node_modules/carbone/examples/flat_table.ods', data, function(err, result){
if (err) return console.log(err);
fs.writeFileSync('flat_table_result.ods', result);
});
To check out the api reference and the documentation, visit carbone.io.
To checkout out the Carbone CLI documentation, visit carbone.io
If you're facing any issues, search a similar issue to ensure it doesn't already exist on Github. Otherwhise, create an issue to help us.
The roadmap is pinned on on the github issues list.
Report generation speed (without network latency), using a basic one-page DOCX template:
10 ms / report
without document conversion (analyzing, injection, rendering)50 ms / report
with a PDF conversion (100 loops, 3 LibreOffice workers, without cold-start)On a MacBook Pro Mid-2015, 2,2 Ghz i7, 16Go.
It could be even better when "code cache" will be activated. Coming soon...
There are two editions of Carbone:
We want to follow the model of Gitlab. The free version must be and must stay generous.
Our ultimate goal
2% percent of our hosted solution revenues will go to charity in three domains: open source software we love, education and environment.
We already know that beneficiaries will be, at least :heart:
Thanks to all Ideolys's direct contributors (random order)
Thanks to all French citizens (Crédit Impôt Recherche, Jeune Entreprise Innovante, BPI)!
Usage (callback style):
carbone.renderCallback('template.docx', data, (err, result, templateName) => {
if(err) {
console.error(err);
} else {
fs.writeFileSync('result.docx', result);
}
});
or:
carbone.render('template.docx', data, (err, result, templateName) => {
if(err) {
console.error(err);
} else {
fs.writeFileSync('result.docx', result);
}
});
Usage (promise style):
carbone.renderPromise('template.docx', data))
.then(({ result }) => {
fs.writeFileSync('result.docx', result);
})
.catch((err) => console.error(err));
or
carbone.render('template.docx', data))
.then(({ result }) => {
fs.writeFileSync('result.docx', result);
})
.catch((err) => console.error(err));
Usage (promise style, executes in worker):
carbone.renderParallel('template.docx', data))
.then(({ result }) => {
fs.writeFileSync('result.docx', result);
})
.catch((err) => console.error(err));
Added formatter :html()
Example: {d.html:html()}
Original | Current |
---|---|
![]() | ![]() |
Added formatter :imageSize(width[,height])
Example: {d.image:imageSize(100,200)}
Original | Current |
---|---|
![]() | ![]() |
Added formatter :imageSize(width[,height])
Example: {d.image:imageSize(100,200)}
Original | Current |
---|---|
![]() | ![]() |
Original | Current |
---|---|
![]() | ![]() |
Original | Current |
---|---|
![]() | ![]() |
Original | Current |
---|---|
![]() | ![]() |
Original | Current |
---|---|
![]() | ![]() |
FAQs
Fast, Simple and Powerful report generator. Injects JSON and produces PDF, DOCX, XLSX, ODT, PPTX, ODS, ...!
The npm package @rodewitsch/carbone receives a total of 27 weekly downloads. As such, @rodewitsch/carbone popularity was classified as not popular.
We found that @rodewitsch/carbone demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.