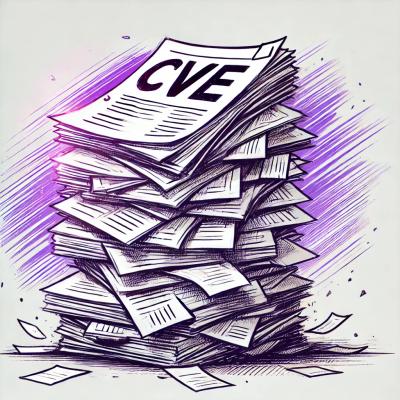
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@rtcamp/wporg-api-client
Advanced tools
WordPress.org API client built in node.js
npm install wporg-api-client
Note: add --save
if you are using npm < 5.0.0
//To Fetch All Plugins List
const { getPluginsList } = require("wporg-api-client");
const pluginsList = async () => {
let data = {};
try {
data = await getPluginsList();
console.log(data, "data");
} catch (error) {
console.log(error.message, "error");
}
};
pluginsList();
Note: Asterisk(*) is used to denote required arguments
Available API versions: 1.0
getCoreTranslations(wp_version, api_version)
Note: wp_version starts from 4.0
getCoreTranslations('4.9.5')
Available API versions: 1.7
getCoreVersionInfo(wp_version, locale, api_version)
getCoreVersionInfo('4.0.1', 'eu')
Available API versions: 1.1
getCoreCreditDetails(wp_version, locale, api_version)
getCoreCreditDetails('4.0.1', 'eu')
Available API versions: 1.0
getCoreChecksums(wp_version*, locale, api_version)
getCoreChecksums('5.5.1', 'eu')
Available API versions: 1.1
getBrowserInfo(useragent*)
getBrowserInfo('Chrome/86.0')
Available API versions: 1.0
getCoreVersionStabilityInfo(wp_version, api_version)
getCoreVersionStabilityInfo() //returns all versions info
getCoreVersionStabilityInfo('4.6.20') //returns specific version info
Available API versions: 1.0
getCoreStats(api_version)
getCoreStats()
Filters list
search(string): Textual search, using a free-form string
tag('string' | 'array'): Return themes with a specified tag or set of tags(array of strings)
author(string): WordPress.org username of the author, pass this filter to return plugins authored by them
page(number): page number default: 1
per_page(number): Plugins to show per page default: 24
browse(string): Predefined themes ordering. Possible values are
Available API versions: 1.1, 1.2
getPluginsList(filters, api_version)
getPluginsList()
getPluginsList({
tag: ['photography', 'blue'],
browse: 'popular',
page: 1,
per_page: 10,
//... other filters
})
filterPluginsBy(filter_key*, filter_value*, page, per_page, api_version)
filterPluginsBy('search', 'buddypress', 1, 5)
filterPluginsBy('tag', ['popup', 'slideshow'])
filterPluginsBy('author', 'wordpressdotorg', 2, 3)
filterPluginsBy('browse', 'popular')
getPluginInfo(plugin_slug, api_version)
getPluginInfo('wordpress-seo');
getPluginHotTagsList(api_version)
Note: tags_count is not implemented in the original api yet
getPluginHotTagsList()
Available API versions: 1.0
getPluginTranslations(slug, plugin_version, api_version)
getPluginTranslations('classic-editor', 1.5);
Available API versions: 1.0
getPluginDownloads(plugin_slug*, limit, api_version)
limit: Downloads in last {limit} days
getPluginDownloads('classic-editor', 7)
getPluginStats(plugin_slug*, api_version)
getPluginStats('classic-editor)
Filters list
search(string): Textual search, using a free-form string
tag('string' | 'array'): Return themes with a specified tag or set of tags(array of strings)
theme(string): Slug of a specific theme to return
author(string): WordPress.org username of the author, pass this filter to return themes authored by them
page(number): page number default: 1
per_page(number): Themes to show per page default: 24
browse(string): Predefined themes ordering. Possible values are
Available API versions: 1.1, 1.2
getPluginsList(filters, api_version)
getPluginsList()
getPluginsList({
tag: ['photography', 'blue'],
browse: 'popular',
page: 1,
per_page: 10,
//... other filters
})
filterThemesBy(filter_key*, filter_value*, page, per_page, api_version)
filterThemesBy('search', 'grid', 1, 15)
filterThemesBy('tag', ['photography', 'blue'])
filterThemesBy('theme', 'gridmag')
filterThemesBy('author', 'wordpressdotorg', 2, 3)
filterThemesBy('browse', 'popular')
getThemeInfo(theme_slug, api_version)
getThemeInfo('simple-grid')
getPopularThemeTags(tags_count, api_version)
getPopularThemeTags()
getPopularThemeTags(5)
Available API versions: 1.0
getThemeTranslations(theme_slug*, theme_version, api_version)
getThemeTranslations('grocery-store', 1.0.2)
Filters list
location(string):
latitude(number | string) and longitude(number | string)
ip(string)
country(string): Country name
timezone(string)
number(number): No of events to show
locale(string)
getEventDetails(params, api_version)
getEventDetails({ country: 'IT' });
getEventDetails({ ip: '136.0.16.1' })
getEventDetails({
latitude: '41.900001525879'
longitude: '12.479999542236'
})
getEventDetails({ location: 'Australia' })
getEventDetails({ number:5, location:'Australia' })
Available API versions: 1.0
getStats(type, api_version)
getStats('php')
getStats('mysql')
getStats('wordpress')
Available API versions: 1.1
generateSecretKey(api_version)
generateSecretKey()
Available API version: 1.1
getPopularImportPlugins(api_version)
getPopularImportPlugins()
FAQs
Node.js client for wordpress.org APIs
We found that @rtcamp/wporg-api-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.