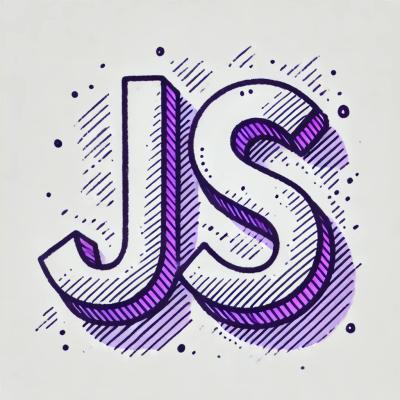
Security News
JavaScript Leaders Demand Oracle Release the JavaScript Trademark
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
@rushstack/node-core-library
Advanced tools
Core libraries that every NodeJS toolchain project should use
The @rushstack/node-core-library provides a collection of essential utilities and lightweight polyfills designed for use in Node.js applications. It aims to offer a standardized set of tools for common operations such as file system manipulation, URL and path handling, and advanced logging capabilities, among others. This library is part of the Rush Stack family of projects, which are focused on providing scalable and reliable engineering tools.
FileSystem operations
Provides a set of utilities for performing file system operations, such as reading, writing, and deleting files, with improved error messages and handling over the native Node.js fs module.
const { FileSystem } = require('@rushstack/node-core-library');
FileSystem.writeFile({
filePath: './example.txt',
contents: 'Hello, world!'
});
Advanced logging
Enables advanced logging capabilities, including support for verbose and error messages, and the ability to customize the output through terminal providers.
const { Terminal, ConsoleTerminalProvider } = require('@rushstack/node-core-library');
const terminalProvider = new ConsoleTerminalProvider();
const terminal = new Terminal(terminalProvider);
terminal.writeLine('Hello, world!');
JSON file handling
Simplifies reading from and writing to JSON files, including support for JSON schema validation and pretty-printing.
const { JsonFile } = require('@rushstack/node-core-library');
const jsonObject = { key: 'value' };
JsonFile.save(jsonObject, './example.json');
fs-extra extends the Node.js native fs module with additional methods, such as copy, move, and remove, making it a popular choice for file system operations. Compared to @rushstack/node-core-library, fs-extra focuses more exclusively on file system enhancements.
winston is a versatile logging library for Node.js. It supports multiple transports (e.g., console, file, HTTP) for logging messages. While @rushstack/node-core-library provides basic logging capabilities, winston offers more extensive features for custom logging scenarios.
chalk is a library for styling terminal text with colors and styles. Unlike @rushstack/node-core-library, which includes a broader set of utilities, chalk focuses specifically on enhancing terminal output aesthetics.
This library provides a conservative set of "core" NodeJS utilities whose purpose is to standardize how we solve common problems across our tooling projects. In order to be considered "core", the class must meet ALL of these criteria:
small and self-contained (i.e. quick to install)
solves a ubiquitous problem (i.e. having one standardized approach is better than allowing each developer to use their favorite library)
broad enough applicability to justify imposing it as a dependency for nearly every NodeJS project
code is design reviewed, API approved, and documented
Examples of "core" functionality that meets this criteria:
This package is NOT intended to be a dumping ground for arbitrary utilities that seem like they might be useful. Code should start somewhere else, and then graduate to node-core-library after its value has already been demonstrated. If in doubt, create your own NPM package.
@rushstack/node-core-library
is part of the Rush Stack family of projects.
FAQs
Core libraries that every NodeJS toolchain project should use
The npm package @rushstack/node-core-library receives a total of 1,957,512 weekly downloads. As such, @rushstack/node-core-library popularity was classified as popular.
We found that @rushstack/node-core-library demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In an open letter, JavaScript community leaders urge Oracle to give up the JavaScript trademark, arguing that it has been effectively abandoned through nonuse.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.