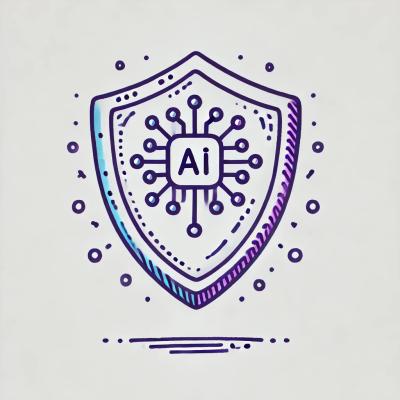
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
@rustable/utils
Advanced tools
Utility TypeScript utilities inspired by Rust, providing type-safe implementations of HashMap, TypeId, deep cloning, hashing, and equality comparison
This is the utilities package of the Rustable project, providing fundamental utilities and type management functionalities. The package implements essential features like type identification, object cloning, hashing, string manipulation, and mutable references.
npm install @rustable/utils
# or
yarn add @rustable/utils
# or
pnpm add @rustable/utils
type_id.ts
)stringify.ts
)hash.ts
)clone.ts
)eq.ts
)mut.ts
)ref.ts
)Import the required utilities from the package:
import { typeId, clone, hash, stringify, Mut, Ref } from '@rustable/utils';
class MyClass {}
const id = typeId(MyClass); // Get type ID for class
// With generic parameters
class Container<T> {}
const stringContainerId = typeId(Container, [String]);
import { deepCopy } from '@rustable/utils';
// Cloning simple objects
const original = { name: 'John', age: 30 };
const clone = deepCopy(original);
// Cloning complex objects with circular references
const complexObj = {
data: [1, 2, 3],
date: new Date(),
};
complexObj.self = complexObj; // circular reference
const cloned = deepCopy(complexObj);
import { hash } from '@rustable/utils';
// Hash simple values
const numberHash = hash(42);
const stringHash = hash('Hello World');
// Hash objects
const objectHash = hash({ x: 1, y: 2 });
const arrayHash = hash([1, 2, 3]);
import { equals } from '@rustable/utils';
// Compare simple values
console.log(equals(5, 5)); // true
console.log(equals('hello', 'hello')); // true
// Compare objects
const obj1 = { x: 1, y: [1, 2, 3] };
const obj2 = { x: 1, y: [1, 2, 3] };
console.log(equals(obj1, obj2)); // true
import { Mut } from '@rustable/utils';
// Create a mutable object reference
let obj = { name: 'Alice', age: 30 };
const mutRef = Mut.of({
get: () => obj,
set: (newValue) => {
obj = newValue;
},
});
// Access and modify properties directly
console.log(mutRef.name); // Output: 'Alice'
mutRef.age = 31;
console.log(obj.age); // Output: 31
// Replace entire object using Mut.ptr
mutRef[Mut.ptr] = { name: 'Bob', age: 25 };
console.log(obj); // Output: { name: 'Bob', age: 25 }
// Get current value using Mut.ptr
console.log(mutRef[Mut.ptr]); // Output: { name: 'Bob', age: 25 }
// Replace using Mut.replace helper
Mut.replace(mutRef, { name: 'Charlie', age: 20 });
console.log(obj); // Output: { name: 'Charlie', age: 20 }
// Working with nested objects
let nested = {
info: {
name: 'Alice',
hobbies: ['reading'],
},
};
const nestedRef = Mut.of({
get: () => nested,
set: (newValue) => {
nested = newValue;
},
});
// Modify nested properties
nestedRef.info.hobbies.push('coding');
console.log(nested.info.hobbies); // Output: ['reading', 'coding']
// Replace nested object
Mut.replace(nestedRef, {
info: {
name: 'Bob',
hobbies: ['gaming'],
},
});
console.log(nested); // Output: { info: { name: 'Bob', hobbies: ['gaming'] } }
import { Ref } from '@rustable/utils';
// Create a reference
const obj = { name: 'Alice', age: 30 };
const ref = Ref.of(obj);
// Modify the reference
ref.name = 'Bob';
console.log(ref.name); // 'Bob'
// Original remains unchanged
console.log(obj.name); // 'Alice'
// Access original through ptr
console.log(ref[Ref.ptr].name); // 'Alice'
The Ref
type provides a way to create immutable references to values. Unlike Mut
, which tracks mutations to the original value, Ref
creates an independent copy that can be modified without affecting the original.
import { Ref } from '@congeer/utils';
// Create a reference
const obj = { name: 'Alice', age: 30 };
const ref = Ref.of(obj);
// Modify the reference
ref.name = 'Bob';
console.log(ref.name); // 'Bob'
// Original remains unchanged
console.log(obj.name); // 'Alice'
// Access original through ptr
console.log(ref[Ref.ptr].name); // 'Alice'
Ref.ptr
symbol// Arrays
const arr = [1, 2, 3];
const arrRef = Ref.of(arr);
arrRef.push(4);
console.log([...arrRef]); // [1, 2, 3, 4]
console.log(arr); // [1, 2, 3]
// Objects with Methods
class User {
constructor(public name: string) {}
setName(name: string) {
this.name = name;
return this;
}
}
const user = new User('Alice');
const userRef = Ref.of(user);
userRef.setName('Bob');
console.log(userRef.name); // 'Bob'
console.log(user.name); // 'Alice'
While Mut
tracks and propagates changes to the original value, Ref
provides isolation:
// Mut modifies original
const mut = Mut.of({ value: 1 });
mut.value = 2;
console.log(mut[Mut.ptr].value); // 2
// Ref keeps original unchanged
const ref = Ref.of({ value: 1 });
ref.value = 2;
console.log(ref[Ref.ptr].value); // 1
Mut
type provides a proxy-based mutable reference that allows direct property access and modificationRef
type provides an immutable reference to values, creating a deep clone of the original valueMIT illuxiza
FAQs
Essential utilities for object cloning, string manipulation, and value comparison in TypeScript, inspired by Rust's standard library.
The npm package @rustable/utils receives a total of 0 weekly downloads. As such, @rustable/utils popularity was classified as not popular.
We found that @rustable/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.