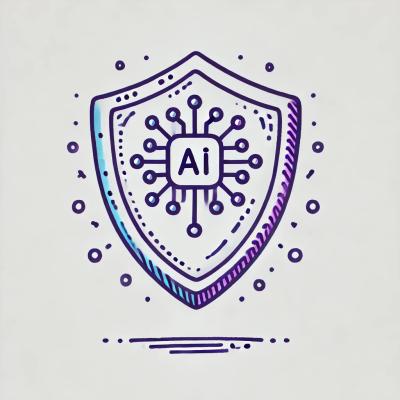
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
@rustable/utils
Advanced tools
Essential utilities for object cloning, string manipulation, and value comparison in TypeScript, inspired by Rust's standard library.
Essential utilities for object cloning, string manipulation, and value comparison in TypeScript, inspired by Rust's standard library.
npm install @rustable/utils
# or with yarn
yarn add @rustable/utils
# or with pnpm
pnpm add @rustable/utils
stringify.ts
)import { stringify } from '@rustable/utils';
// Basic value stringification
stringify(42); // '42'
stringify('hello'); // 'hello'
stringify(null); // ''
stringify(undefined); // ''
// Complex object stringification
const user = {
name: 'John',
info: { age: 30 },
};
stringify(user); // '{info:{age:30},name:"John"}'
// Special types
stringify(Symbol('key')); // 'Symbol(key)'
stringify(42n); // '42'
stringify(() => {}); // 'function...'
// Arrays and Maps
stringify([1, 2, 3]); // '[1,2,3]'
stringify(new Map([['a', 1]])); // 'Map{a:1}'
// Dates
stringify(new Date(1234567890)); // 'Date("1234567890")'
hash.ts
)import { hash } from '@rustable/utils';
// Primitive values
hash('hello'); // djb2 hash of the string
hash(42); // 42 (number as is)
hash(true); // 1
hash(false); // 0
hash(null); // -1
hash(undefined); // -1
// Objects are hashed based on their string representation
const obj = { x: 1, y: 2 };
hash(obj); // hash of '{x:1,y:2}'
clone.ts
)import { deepClone } from '@rustable/utils';
// Clone primitive values
const num = deepClone(42);
const str = deepClone('hello');
// Clone complex objects
const original = {
date: new Date(),
regex: /test/g,
set: new Set([1, 2, 3]),
map: new Map([
['a', 1],
['b', 2],
]),
nested: { array: [1, 2, 3] },
};
const cloned = deepClone(original);
// Each property is properly cloned:
// - date is a new Date instance
// - regex is a new RegExp
// - set is a new Set
// - map is a new Map
// - nested objects are deeply cloned
// Custom clone method support
class Point {
constructor(
public x: number,
public y: number,
) {}
clone() {
return new Point(this.x, this.y);
}
}
const point = new Point(1, 2);
const clonedPoint = deepClone(point); // Uses Point's clone method
eq.ts
)import { equals } from '@rustable/utils';
// Compare primitive values
equals(42, 42); // true
equals('hello', 'hello'); // true
// Compare objects
equals({ x: 1 }, { x: 1 }); // true
equals([1, 2], [1, 2]); // true
// Compare nested structures
const obj1 = { data: { points: [1, 2] } };
const obj2 = { data: { points: [1, 2] } };
equals(obj1, obj2); // true
val.ts
)import { Val } from '@rustable/utils';
// Create an immutable reference
const original = { count: 0, data: [1, 2, 3] };
const val = Val(original);
// Modifications don't affect original
val.count = 1;
val.data.push(4);
console.log(original.count); // Still 0
console.log(original.data); // Still [1, 2, 3]
// Access original through symbol
const originalRef = val[Val.ptr];
console.log(originalRef === original); // true
ptr.ts
)import { Ptr } from '@rustable/utils';
// Basic pointer usage with method support
class Counter {
count = 0;
increment() {
this.count++;
}
}
let counter = new Counter();
const ptr = Ptr({
get: () => counter,
set: (v) => (counter = v),
});
// Method calls and property access work transparently
ptr.increment();
console.log(counter.count); // 1
// Value replacement
ptr[Ptr.ptr] = new Counter();
MIT © illuxiza
FAQs
Essential utilities for object cloning, string manipulation, and value comparison in TypeScript, inspired by Rust's standard library.
The npm package @rustable/utils receives a total of 0 weekly downloads. As such, @rustable/utils popularity was classified as not popular.
We found that @rustable/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.