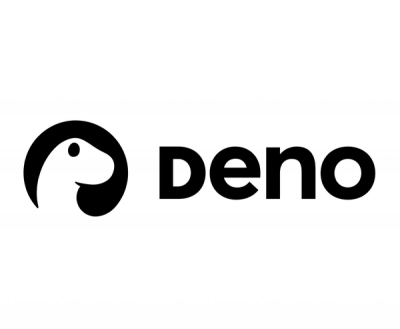
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@sa11y/jest
Advanced tools
jest
Accessibility matcher for Jest
The accessibility matcher helper APIs need to be registered with Jest before they can be used in tests.
You can set up the a11y matchers once at the project level to make it available to all the Jest tests in the project. For an example look at the Integration tests.
jest-setup.js
) and add the following code that registers the a11y matchersconst { registerSa11yMatcher } = require('@sa11y/jest');
registerSa11yMatcher();
jest.config.js
at the root of your project, add:module.exports = {
setupFilesAfterEnv: ['<rootDir>/jest-setup.js'],
};
Invoke registerSa11yMatcher
before using the accessibility matchers in the tests e.g.
import { registerSa11yMatcher } from '@sa11y/jest';
beforeAll(() => {
registerSa11yMatcher();
});
toBeAccessible
must be invoked with await
or the equivalent supported async method in your env.
toBeAccessible
jest matcher can be invoked on a document
(JSDOM) or an HTML element
to check for accessibilityimport { recommended } from '@sa11y/preset-rules';
import { registerSa11yMatcher } from '@sa11y/jest';
beforeAll(() => {
registerSa11yMatcher();
});
it('should be accessible', async () => {
// Setup DOM to be tested for accessibility
//...
// assert that DOM is accessible (using extended preset-rule)
await expect(document).toBeAccessible();
// Can be used to test accessibility of a specific HTML element
const elem = document.getElementById('foo');
await expect(elem).toBeAccessible();
// use recommended preset-rule
await expect(document).toBeAccessible(recommended);
});
FAQs
Accessibility testing matcher for Jest
The npm package @sa11y/jest receives a total of 9,992 weekly downloads. As such, @sa11y/jest popularity was classified as popular.
We found that @sa11y/jest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.