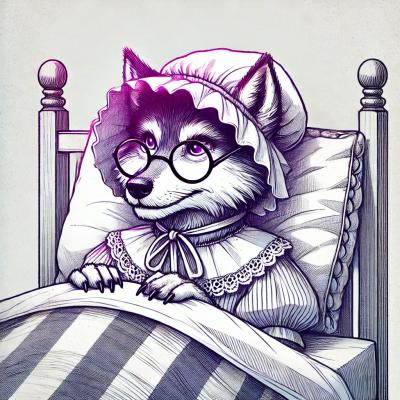
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@salesforce/apex-node
Advanced tools
@salesforce/apex-node is an npm package that allows developers to interact with Salesforce Apex classes and methods from Node.js applications. It provides functionalities to execute Apex code, run tests, and retrieve test results programmatically.
Execute Anonymous Apex
This feature allows you to execute anonymous Apex code on a Salesforce instance. The code sample demonstrates how to log in to Salesforce and execute a simple Apex code snippet.
const { Connection, ExecuteAnonymousResponse } = require('@salesforce/apex-node');
async function executeAnonymousApex() {
const conn = new Connection({ loginUrl: 'https://login.salesforce.com' });
await conn.login('username', 'password');
const apexCode = 'System.debug(\'Hello, World!\');';
const result = await conn.tooling.executeAnonymous(apexCode);
console.log(result);
}
executeAnonymousApex();
Run Apex Tests
This feature allows you to run Apex tests asynchronously. The code sample demonstrates how to log in to Salesforce and run tests for a specified list of test classes.
const { Connection, RunTestsAsynchronousResponse } = require('@salesforce/apex-node');
async function runApexTests() {
const conn = new Connection({ loginUrl: 'https://login.salesforce.com' });
await conn.login('username', 'password');
const testClasses = ['MyTestClass'];
const result = await conn.tooling.runTestsAsynchronous(testClasses);
console.log(result);
}
runApexTests();
Retrieve Test Results
This feature allows you to retrieve the results of previously run Apex tests. The code sample demonstrates how to log in to Salesforce and query the ApexTestResult object to get test results.
const { Connection, QueryResult } = require('@salesforce/apex-node');
async function getTestResults() {
const conn = new Connection({ loginUrl: 'https://login.salesforce.com' });
await conn.login('username', 'password');
const query = 'SELECT Id, Status, ApexClassId FROM ApexTestResult';
const result = await conn.tooling.query(query);
console.log(result.records);
}
getTestResults();
Jsforce is a powerful library for Salesforce API integration in JavaScript. It provides a wide range of functionalities including data manipulation, metadata API access, and Apex execution. Compared to @salesforce/apex-node, Jsforce offers a broader set of features for interacting with Salesforce, but may require more configuration for specific Apex-related tasks.
Typescript library to support the Salesforce Extensions for VS Code and the Apex Plugin for the Salesforce CLI.
Note: Please report any issues via the Issues tab.
If you're interested in contributing, take a look at the CONTRIBUTING guide.
If you're interested in building the plugin and library locally, take a look at the Developing doc.
FAQs
Salesforce JS library for Apex
We found that @salesforce/apex-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.