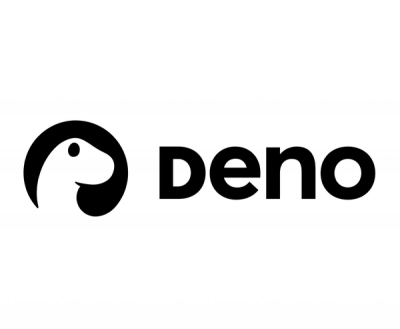
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@salesforce/kit
Advanced tools
The @salesforce/kit npm package is a utility library designed to help developers interact with Salesforce environments in a more streamlined and efficient manner. It provides a set of tools and functionalities that simplify common tasks related to configuration, environment handling, and other utilities necessary for Salesforce development.
Configuration Management
This feature allows developers to aggregate and manage configuration settings from various sources. The code sample demonstrates how to create a ConfigAggregator instance and retrieve configuration information.
const { ConfigAggregator } = require('@salesforce/kit');
async function getConfig() {
const config = await ConfigAggregator.create();
console.log('Loaded config:', config.getInfo());
}
getConfig();
Environment Detection
This feature helps in detecting and managing different environment settings. The code sample checks if the application is running in a production environment using an environment variable.
const { env } = require('@salesforce/kit');
if (env.getBoolean('IS_PRODUCTION')) {
console.log('Running in production mode');
} else {
console.log('Running in development mode');
}
Jsforce is another popular npm package for Salesforce development. It primarily focuses on Salesforce API connections and data manipulation, providing a robust set of features for interacting directly with Salesforce data. Unlike @salesforce/kit, which is more about configuration and environment management, jsforce offers extensive capabilities for CRUD operations, querying, and streaming API support.
Nforce is also tailored for Salesforce development but is more lightweight compared to jsforce. It provides essential functionalities for authentication, data manipulation, and streaming but with a simpler interface. While @salesforce/kit focuses on configuration and environment aspects, nforce provides basic tools for direct Salesforce API interaction.
A collection of commonly needed utilities used by the Salesforce CLI and the libraries it is built on. It includes high level support for parsing and working with JSON data, interacting with environment variables, a common error base type, a minimal lodash replacement, and support for commonly needed design patterns, among other things. It is intended specifically for use in Node.js (version 8 or newer) projects -- YMMV in the browser.
see the API documentation for more details on each of the utilities that kit
provides.
This library depends upon another Salesforce TypeScript library, @salesforce/ts-types. The API documentation for this library refers to several types that you will find in ts-types
. Some lodash
replacement functions are also found in ts-types
.
FAQs
Commonly needed utilities for TypeScript and JavaScript
The npm package @salesforce/kit receives a total of 665,252 weekly downloads. As such, @salesforce/kit popularity was classified as popular.
We found that @salesforce/kit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.