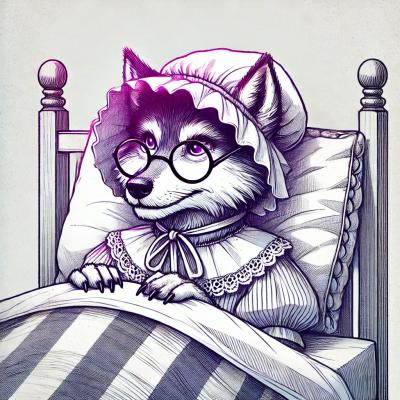
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@salesforce/sf-plugins-core
Advanced tools
@salesforce/sf-plugins-core is a core library for building Salesforce CLI plugins. It provides a set of utilities and base classes to streamline the development of plugins for the Salesforce CLI, enabling developers to create commands, handle user input, manage configuration, and interact with Salesforce APIs.
Command Base Class
The Command Base Class feature allows developers to create new CLI commands by extending the SfCommand class. This provides a structured way to define command behavior and output.
const { SfCommand } = require('@salesforce/sf-plugins-core');
class MyCommand extends SfCommand {
async run() {
this.log('Hello, Salesforce CLI!');
}
}
module.exports = MyCommand;
User Input Handling
User Input Handling feature allows developers to define and parse command-line flags and arguments using the Flags utility. This makes it easy to handle user input in a consistent manner.
const { SfCommand, Flags } = require('@salesforce/sf-plugins-core');
class MyCommand extends SfCommand {
static flags = {
name: Flags.string({ char: 'n', description: 'name to print' })
};
async run() {
const { flags } = this.parse(MyCommand);
this.log(`Hello, ${flags.name}!`);
}
}
module.exports = MyCommand;
Configuration Management
Configuration Management feature provides utilities to load and manage configuration settings for the CLI. This helps in maintaining consistent configuration across different commands and plugins.
const { SfCommand, Config } = require('@salesforce/sf-plugins-core');
class MyCommand extends SfCommand {
async run() {
const config = await Config.load();
this.log(`Current config: ${JSON.stringify(config)}`);
}
}
module.exports = MyCommand;
Salesforce API Interaction
Salesforce API Interaction feature allows developers to easily create connections to Salesforce and perform API operations such as queries. This simplifies the process of interacting with Salesforce data from CLI commands.
const { SfCommand, Connection } = require('@salesforce/sf-plugins-core');
class MyCommand extends SfCommand {
async run() {
const conn = await Connection.create({ authInfo: this.authInfo });
const result = await conn.query('SELECT Id, Name FROM Account');
this.log(`Fetched ${result.records.length} accounts`);
}
}
module.exports = MyCommand;
oclif is a framework for building command-line interfaces (CLIs) in Node.js. It provides a robust set of tools for creating commands, handling input, and managing plugins. Compared to @salesforce/sf-plugins-core, oclif is more general-purpose and not specifically tailored to Salesforce, but it offers similar functionality for building CLI applications.
commander is a popular Node.js library for building command-line interfaces. It provides a simple and flexible way to define commands, options, and arguments. While it does not offer the same level of integration with Salesforce as @salesforce/sf-plugins-core, it is widely used for general CLI development.
yargs is another powerful library for building command-line tools in Node.js. It focuses on parsing command-line arguments and generating user-friendly interfaces. Like commander, yargs is not specific to Salesforce but provides a comprehensive set of features for CLI development.
The @salesforce/sf-plugins-core provides utilities for writing sf plugins.
Docs: https://salesforcecli.github.io/sf-plugins-core
The SfCommand abstract class extends @oclif/core's Command class for examples of how to build a definition. ) class and adds useful extensions to ease the development of commands for use in the Salesforce Unified CLI.
--json
flag.Flags is a convenience reference to @oclif/core#Flags
These flags can be imported into a command and used like any other flag. See code examples in the links
Want to verify SfCommand Ux behavior (warnings, tables, spinners, prompts)? Check out the functions in `stubUx``.
FAQs
Utils for writing Salesforce CLI plugins
The npm package @salesforce/sf-plugins-core receives a total of 993,832 weekly downloads. As such, @salesforce/sf-plugins-core popularity was classified as popular.
We found that @salesforce/sf-plugins-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.