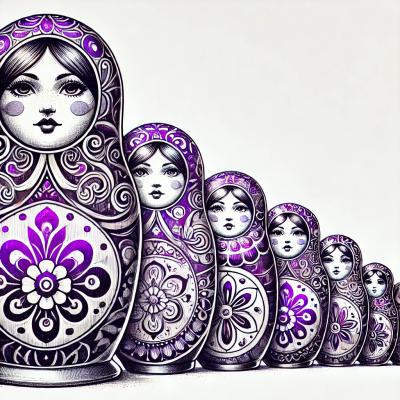
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@sanity/block-tools
Advanced tools
Can format HTML, Slate JSON or Sanity block array into any other format.
@sanity/block-tools is a utility library for working with Sanity's block content. It provides tools for parsing, serializing, and manipulating block content, which is a rich text format used in Sanity's content management system.
Parsing Block Content
This feature allows you to parse HTML content into Sanity block content. The `htmlToBlocks` function converts HTML strings into an array of block objects that can be used within Sanity.
const { htmlToBlocks } = require('@sanity/block-tools');
const html = '<p>Hello, world!</p>';
const blocks = htmlToBlocks(html, { type: 'block' });
console.log(blocks);
Serializing Block Content
This feature allows you to serialize Sanity block content back into HTML. The `blocksToHtml` function takes an array of block objects and converts them into an HTML string.
const { blocksToHtml } = require('@sanity/block-tools');
const blocks = [{ _type: 'block', children: [{ _type: 'span', text: 'Hello, world!' }] }];
const html = blocksToHtml({ blocks });
console.log(html);
Custom Block Serializers
This feature allows you to define custom serializers for different block types. The `blocksToHtml` function can take a `serializers` object to customize how different block types are converted to HTML.
const { blocksToHtml } = require('@sanity/block-tools');
const blocks = [{ _type: 'block', children: [{ _type: 'span', text: 'Hello, world!' }] }];
const customSerializers = {
types: {
block: (props) => `<p style="color: red;">${props.children}</p>`
}
};
const html = blocksToHtml({ blocks, serializers: customSerializers });
console.log(html);
Draft.js is a rich text editor framework for React, built by Facebook. It provides a set of tools for building rich text editors, including parsing and serializing content. Compared to @sanity/block-tools, Draft.js is more focused on providing a complete editor experience, while @sanity/block-tools is more about manipulating block content.
Slate is another rich text editor framework for React. It provides a highly customizable and extensible architecture for building rich text editors. Like Draft.js, Slate offers tools for parsing and serializing content, but it also provides more flexibility in terms of editor customization. Compared to @sanity/block-tools, Slate is more focused on the editor experience rather than just content manipulation.
ProseMirror is a toolkit for building rich text editors. It provides a set of tools for parsing, serializing, and manipulating rich text content. ProseMirror is highly customizable and can be used to build complex editor experiences. Compared to @sanity/block-tools, ProseMirror offers more low-level control over the editor and content manipulation.
Various tools for processing Sanity block content. Mostly used internally in the Studio code, but it got some nice functions (especially htmlToBlocks
) which is handy when you are importing data from HTML into your dataset as block text.
NOTE: To use @sanity/block-tools
in a Node.js script, you will need to provide a parseHtml
method - generally using JSDOM
. Read more.
Let's start with a complete example:
import {Schema} from '@sanity/schema'
import {htmlToBlocks, getBlockContentFeatures} from '@sanity/block-tools'
// Start with compiling a schema we can work against
const defaultSchema = Schema.compile({
name: 'myBlog',
types: [
{
type: 'object',
name: 'blogPost',
fields: [
{
title: 'Title',
type: 'string',
name: 'title',
},
{
title: 'Body',
name: 'body',
type: 'array',
of: [{type: 'block'}],
},
],
},
],
})
// The compiled schema type for the content type that holds the block array
const blockContentType = defaultSchema
.get('blogPost')
.fields.find((field) => field.name === 'body').type
// Convert HTML to block array
const blocks = htmlToBlocks('<html><body><h1>Hello world!</h1><body></html>', blockContentType)
// Outputs
//
// {
// _type: 'block',
// style: 'h1'
// children: [
// {
// _type: 'span'
// text: 'Hello world!'
// }
// ]
// }
// Get the feature-set of a blockContentType
const features = getBlockContentFeatures(blockContentType)
htmlToBlocks(html, blockContentType, options)
(html deserializer)This will deserialize the input html (string) into blocks.
html
The stringified version of the HTML you are importing
blockContentType
A compiled version of the block content schema type.
The deserializer will respect the schema when deserializing the HTML elements to blocks.
It only supports a subset of HTML tags. Any HTML tag not in the block-tools whitelist will be deserialized to normal blocks/spans.
For instance, if the schema doesn't allow H2 styles, all H2 HTML elements will be output like this:
{
_type: 'block',
style: 'normal'
children: [
{
_type: 'span'
text: 'Hello world!'
}
]
}
options
(optional)parseHtml
The HTML-deserialization is done by default by the browser's native DOMParser.
On the server side you can give the function parseHtml
that parses the html into a DOMParser compatible model / API.
const {JSDOM} = require('jsdom')
const {htmlToBlocks} = require('@sanity/block-tools')
const blocks = htmlToBlocks('<html><body><h1>Hello world!</h1><body></html>', blockContentType, {
parseHtml: (html) => new JSDOM(html).window.document,
})
rules
You may add your own rules to deal with special HTML cases.
htmlToBlocks(
'<html><body><pre><code>const foo = "bar"</code></pre></body></html>',
blockContentType,
{
parseHtml: (html) => new JSDOM(html),
rules: [
// Special rule for code blocks
{
deserialize(el, next, block) {
if (el.tagName.toLowerCase() != 'pre') {
return undefined
}
const code = el.children[0]
const childNodes =
code && code.tagName.toLowerCase() === 'code' ? code.childNodes : el.childNodes
let text = ''
childNodes.forEach((node) => {
text += node.textContent
})
// Return this as an own block (via block helper function), instead of appending it to a default block's children
return block({
_type: 'code',
language: 'javascript',
text: text,
})
},
},
],
},
)
normalizeBlock(block, [options={}])
Normalize a block object structure to make sure it has what it needs.
import {normalizeBlock} from '@sanity/block-tools'
const partialBlock = {
_type: 'block',
children: [
{
_type: 'span',
text: 'Foobar',
marks: ['strong', 'df324e2qwe'],
},
],
}
normalizeBlock(partialBlock, {allowedDecorators: ['strong']})
Will produce
{
_key: 'randomKey0',
_type: 'block',
children: [
{
_key: 'randomKey00',
_type: 'span',
marks: ['strong'],
text: 'Foobar'
}
],
markDefs: []
}
getBlockContentFeatures(blockContentType)
Will return an object with the features enabled for the input block content type.
{
annotations: [{title: 'Link', value: 'link'}],
decorators: [
{title: 'Strong', value: 'strong'},
{title: 'Emphasis', value: 'em'},
{title: 'Code', value: 'code'},
{title: 'Underline', value: 'underline'},
{title: 'Strike', value: 'strike-through'}
],
styles: [
{title: 'Normal', value: 'normal'},
{title: 'Heading 1', value: 'h1'},
{title: 'H2', value: 'h2'},
{title: 'H3', value: 'h3'},
{title: 'H4', value: 'h4'},
{title: 'H5', value: 'h5'},
{title: 'H6', value: 'h6'},
{title: 'Quote', value: 'blockquote'}
]
}
FAQs
Can format HTML, Slate JSON or Sanity block array into any other format.
The npm package @sanity/block-tools receives a total of 90,067 weekly downloads. As such, @sanity/block-tools popularity was classified as popular.
We found that @sanity/block-tools demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 64 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.