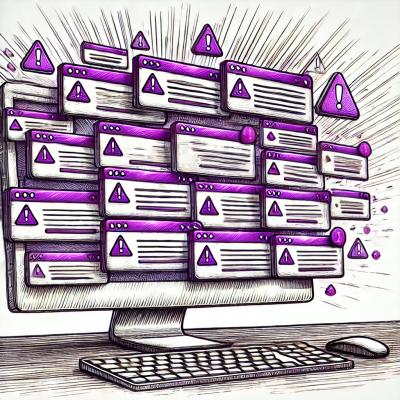
Security News
Combatting Alert Fatigue by Prioritizing Malicious Intent
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
@sapphire/async-queue
Advanced tools
Sequential asynchronous lock-based queue for promises
@sapphire/async-queue is a utility for managing asynchronous tasks in a sequential manner. It ensures that tasks are executed one after another, preventing race conditions and ensuring order.
Basic Queue Usage
This code demonstrates the basic usage of @sapphire/async-queue. It creates a queue and ensures that task1 and task2 are executed sequentially.
const { AsyncQueue } = require('@sapphire/async-queue');
const queue = new AsyncQueue();
async function task1() {
await queue.wait();
console.log('Task 1 started');
setTimeout(() => {
console.log('Task 1 completed');
queue.shift();
}, 1000);
}
async function task2() {
await queue.wait();
console.log('Task 2 started');
setTimeout(() => {
console.log('Task 2 completed');
queue.shift();
}, 500);
}
task1();
task2();
Handling Errors in Queue
This code demonstrates how to handle errors within the queue. Even if a task throws an error, the queue continues to process the next task.
const { AsyncQueue } = require('@sapphire/async-queue');
const queue = new AsyncQueue();
async function taskWithError() {
await queue.wait();
console.log('Task with error started');
setTimeout(() => {
console.log('Task with error encountered an error');
queue.shift();
throw new Error('Task error');
}, 1000);
}
async function taskAfterError() {
await queue.wait();
console.log('Task after error started');
setTimeout(() => {
console.log('Task after error completed');
queue.shift();
}, 500);
}
taskWithError().catch(console.error);
taskAfterError();
The 'async' package provides a wide range of utilities for working with asynchronous JavaScript. It includes functions for parallel and sequential execution, among others. Compared to @sapphire/async-queue, 'async' offers more comprehensive functionality but can be more complex to use for simple sequential task management.
The 'p-queue' package is a promise queue with concurrency control. It allows you to manage the execution of promises with a specified concurrency level. While 'p-queue' offers more advanced features like concurrency control, @sapphire/async-queue focuses on simple sequential execution.
The 'queue' package is a simple and efficient queue implementation for Node.js. It supports basic queue operations and is easy to use. Compared to @sapphire/async-queue, it lacks built-in support for asynchronous task management but can be extended for such use cases.
Table of Contents
You can use the following command to install this package, or replace npm install
with your package manager of choice.
npm install @sapphire/async-queue
Note: While this section uses require
, the imports match 1:1 with ESM imports. For example const { AsyncQueue } = require('@sapphire/async-queue')
equals import { AsyncQueue } from '@sapphire/async-queue'
.
// Require the AsyncQueue class
const { AsyncQueue } = require('@sapphire/async-queue');
const queue = new AsyncQueue();
async function request(url, options) {
// Wait and lock the queue
await queue.wait();
try {
// Perform the operation sequentially
return await fetch(url, options);
} finally {
// Unlock the next promise in the queue
queue.shift();
}
}
request(someUrl1, someOptions1);
// Will call fetch() immediately
request(someUrl2, someOptions2);
// Will call fetch() after the first finished
request(someUrl3, someOptions3);
// Will call fetch() after the second finished
Sapphire Community is and always will be open source, even if we don't get donations. That being said, we know there are amazing people who may still want to donate just to show their appreciation. Thank you very much in advance!
We accept donations through Open Collective, Ko-fi, PayPal, Patreon, and GitHub Sponsorships. You can use the buttons below to donate through your method of choice.
Donate With | Address |
---|---|
Open Collective | Click Here |
Ko-fi | Click Here |
Patreon | Click Here |
PayPal | Click Here |
Please make sure to read the Contributing Guide before making a pull request.
Thank you to all the people who already contributed to Sapphire!
FAQs
Sequential asynchronous lock-based queue for promises
The npm package @sapphire/async-queue receives a total of 184,851 weekly downloads. As such, @sapphire/async-queue popularity was classified as popular.
We found that @sapphire/async-queue demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.