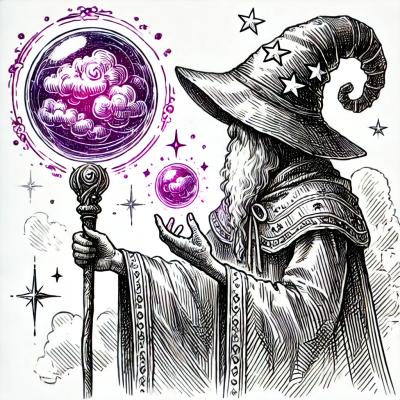
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@scalecube/browser
Advanced tools
[](https://gitter.im/scalecube-js/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
This is part of scalecube-js project, see more at https://github.com/scalecube/scalecube-js
Full documentation
This package provides Scalecube's solution with default setting for working in browsers.
yarn add @scalecube/browser
or npm i @scalecube/browser
import { createMicroservice, ASYNC_MODEL_TYPES } from '@scalecube/browser';
create a seed
export const MySeedAddress: 'seed';
// Create a service
createMicroservice({
address : MySeedAddress
});
Create a service
// Create service definition
export const greetingServiceDefinition = {
serviceName: 'GreetingService',
methods: {
hello: {
asyncModel: ASYNC_MODEL_TYPES.REQUEST_RESPONSE,
}
},
};
// Create a service
createMicroservice({
service : [{
definition: greetingServiceDefinition,
reference: {
hello : (name) => `Hello ${name}`
},
}],
seedAddress : MySeedAddress
});
Use a service
const microservice = createMicroservice({seedAddress : MySeedAddress})
// With proxy
const greetingService = microservice.createProxy({
serviceDefinition: greetingServiceDefinition
});
greetingService.hello('ME').then(console.log) // Hello ME
We let Scalecube choose our addresses for us, we know only the seed address.
After we connected to the seed we will see the whole cluster.
In the browser we don't need to import modules, we can create multiple bundles, scalecube will discover the available services
Dependency Injection
createMicroservice({
seedAddress : MySeedAddress,
services: [
{
definition: serviceB,
reference: ({ createProxy, createServiceCall }) => {
const greetingService = createProxy({serviceDefinition: greetingServiceDefinition });
return new ServiceB(greetingService);
}
}
]
})
please Read before starting to work with scalecube.
this package already transpile the code to es5.
for old browser support please add:
<script nomodule src="https://cdnjs.cloudflare.com/ajax/libs/babel-polyfill/7.6.0/polyfill.min.js"></script>
<script nomodule src="https://cdn.jsdelivr.net/npm/proxy-polyfill@0.3.0/proxy.min.js"></script>
FAQs
[](https://gitter.im/scalecube-js/Lobby?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
The npm package @scalecube/browser receives a total of 3 weekly downloads. As such, @scalecube/browser popularity was classified as not popular.
We found that @scalecube/browser demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.