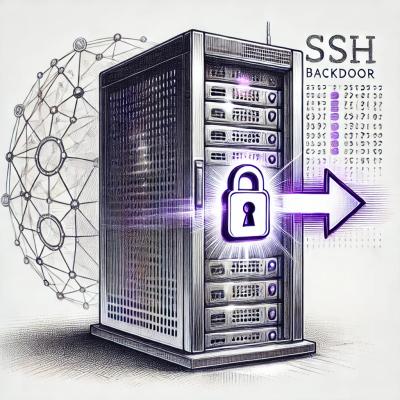
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@secux/app-btc
Advanced tools
@secux/app-btc
SecuX Hardware Wallet BTC API
import { SecuxBTC } from "@secux/app-btc";
First, create instance of ITransport.
Get address by purpose and script type.
const path = "m/84'/0'/0'/0/0";
const address = await device.getAddress(path);
/*
// transfer data to hardware wallet by custom transport layer.
const data = SecuxBTC.prepareAddress(path);
const response = await device.Exchange(data);
const address = SecuxBTC.resolveAddress(response, path);
*/
cnost address = await device.getAddress("m/49'/0'/0'/0/0");
const address = await device.getAddress("m/44'/0'/0'/0/0");
For bitcoin ecosystem, you can use specific cointype by the coin.
dogecoin
const address = await device.getAddress("m/84'/3'/0'/0/0");
litecoin
const address = await device.getAddress("m/49'/2'/0'/0/0");
bitcoincash
const address = await device.getAddress("m/44'/145'/0'/0/0");
Sign transaction.
const inputs = [
{
hash: "0b062e71e165fba9634d9fb1b5ba703e774bf374815b1f5a617c8d1e7d43dc01",
vout: 0,
// optional, give raw transaction data for checking
txHex: "0100000001b103a004f672080ceae8277e83c296b5ac090ae78157979211da3e2d41399d1b010000006b483045022100f19d88e6a17789dc399ff2a93b4516bb44af32928d4986138f1a4f7f37ab277b022046fc14c958bc8aa97fea1d2fbf80982534cf51634d46c4d5178e5ca6698bca07012102f8667cfb5b80c3695e3f0c9078589cb04e8d15e71bdae89ebf24b82f9d663d5cffffffff02bc020000000000001976a9145c592f40134c6179a1ce5b06b28d5c2ae443113188ac00040000000000001976a9146d65ced4ef49e23cdbb4be9d510b38e5be28e10688ac00000000",
satoshis: 700,
path: "m/44'/0'/0'/0/0",
// for custom transport layer, each utxo need publickey.
// publickey: "03aaeb52dd7494c361049de67cc680e83ebcbbbdbeb13637d92cd845f70308af5e"
},
{
hash: "07ad0a13e501d292bc8b9e16a3a8b62f99f77ab9e37ea8d3b8453984a2899984",
vout: 0,
// optional, you can use specific script for each input
// script: ScriptType.P2SH_P2PKH,
satoshis: 6000,
path: "m/49'/0'/0'/0/0",
// for custom transport layer, each utxo need publickey.
// publickey: "039b3b694b8fc5b5e07fb069c783cac754f5d38c3e08bed1960e31fdb1dda35c24"
},
{
hash: "8686aee2b9dcf559798b9718ed26ca92e0c64bef11c433e576cae658678c497d",
vout: 1,
satoshis: 1083,
path: "m/84'/0'/0'/1/0",
// for custom transport layer, each utxo need publickey.
// publickey: "03025324888e429ab8e3dbaf1f7802648b9cd01e9b418485c5fa4c1b9b5700e1a6"
}
];
const to = {
address: "bc1qs0k3ekx0z7a7yuq3lse7prw373s8cr8lhxvccd",
satoshis: 1500
};
const utxo = {
path: "m/44'/0'/0'/0/0",
satoshis: 6100,
// for custom transport layer, each utxo need publickey.
// publickey: "03aaeb52dd7494c361049de67cc680e83ebcbbbdbeb13637d92cd845f70308af5e"
};
const { raw_tx } = await device.sign(inputs, { to, utxo });
/*
// transfer data to hardware wallet by custom transport layer.
const { commandData, rawTx } = SecuxBTC.prepareSign(inputs, { to, utxo });
const rsp = await device.Exchange(commandData);
const signed = SecuxBTC.resloveTransaction(rsp, rawTx, inputs.map(x => x.publickey));
*/
BTC package for SecuX device
Kind: global class
communicationData
string
string
communicationData
string
communicationData
string
prepared
Array.<string>
string
communicationData
Prepare data for address generation.
Returns: communicationData
- data for sending to device
Param | Type | Description |
---|---|---|
path | string | BIP32 path, ex: m/44'/0'/0'/0/0 |
[option] | AddressOption | for path validation |
string
Convert publickey to BTC address.
Returns: string
- address
Param | Type | Description |
---|---|---|
publickey | string | Buffer | secp256k1 publickey (hex string or buffer) |
path | string | PathObject | BIP32 path, ex: m/44'/0'/0'/0/0 |
string
Generate address from response data.
Returns: string
- address
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
path | string | PathObject | BIP32 path, ex: m/44'/0'/0'/0/0 |
communicationData
Prepare data for secp256k1 publickey.
Returns: communicationData
- data for sending to device
Param | Type | Description |
---|---|---|
path | string | BIP32 path, ex: m/44'/0'/0'/0/0 |
[option] | AddressOption | for path validation |
string
Resolve secp256k1 publickey from response data.
Returns: string
- secp256k1 publickey (hex string)
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
communicationData
Prepare data for extended publickey generation.
Returns: communicationData
- data for sending to device
Param | Type | Description |
---|---|---|
path | string | BIP32 path, ex: m/44'/0'/0'/0/0 |
string
Generate extended publickey from response data.
Returns: string
- extended publickey (xpub, ypub or zpub)
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
path | string | BIP32 path, ex: m/44'/0'/0'/0/0 |
prepared
Prepare data for signing.
Param | Type | Description |
---|---|---|
inputs | txInput | array of utxo object |
outputs | txOutput | output object |
Array.<string>
Reslove signature from response data.
Returns: Array.<string>
- signature array of hex string
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
string
Serialize transaction wtih signature for broadcasting.
Returns: string
- signed raw transaction
Param | Type | Description |
---|---|---|
response | communicationData | data from device |
unsigned | string | unsigned raw transaction |
publickeys | Array.<communicationData> | secp256k1 publickey correspond to each input |
[coin] | CoinType | default: CoinType.BITCOIN |
Properties
Name | Type | Description |
---|---|---|
path | string | BIP32 path refer to utxo |
publickey | string | Buffer | scep256k1 publickey from path |
hash | string | referenced transaction hash |
vout | number | referenced transaction output index |
satoshis | number | referenced transaction output amount |
[script] | ScriptType | script type related to path |
[txHex] | string | referenced raw transaction for validation |
Properties
Name | Type | Description |
---|---|---|
to | txOutputAddress | txOutputScriptExtened | receiving address information |
[utxo] | txOutputScriptExtened | changes |
Properties
Name | Type | Description |
---|---|---|
address | string | receiving address |
satoshis | number | receiving amount |
Properties
Name | Type | Description |
---|---|---|
path | string | BIP32 path |
publickey | string | Buffer | scep256k1 publickey from path |
satoshis | number | amount |
[script] | ScriptType | script type related to path |
Properties
Name | Type | Description |
---|---|---|
commandData | communicationData | data for sending to device |
rawTx | string | unsigned raw transaction |
Properties
Name | Type | Description |
---|---|---|
[coin] | CoinType | enum |
[script] | ScriptType | enum |
© 2018-21 SecuX Technology Inc.
authors:
andersonwu@secuxtech.com
FAQs
SecuX Hardware Wallet BTC API
The npm package @secux/app-btc receives a total of 36 weekly downloads. As such, @secux/app-btc popularity was classified as not popular.
We found that @secux/app-btc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.