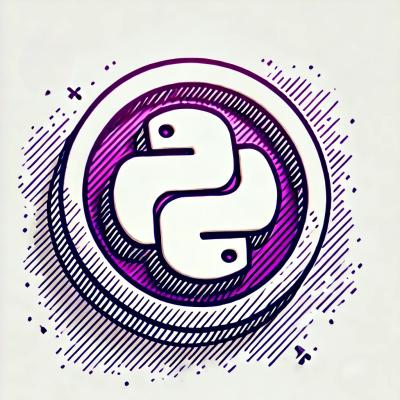
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
@segment/analytics-node
Advanced tools
> ### Warning: This library is in [public beta](https://segment.com/legal/first-access-beta-preview) ⚠️
@segment/analytics-node is a Node.js client for Segment, a customer data platform that helps you collect, clean, and control your customer data. This package allows you to send data from your Node.js applications to Segment, which can then route it to various analytics and marketing tools.
Track
The `track` method allows you to record any actions your users perform. It is useful for tracking events like purchases, sign-ups, or any other user activity.
const Analytics = require('@segment/analytics-node');
const analytics = new Analytics('YOUR_WRITE_KEY');
analytics.track({
userId: 'user123',
event: 'Item Purchased',
properties: {
item: 'T-shirt',
price: 19.99
}
});
Identify
The `identify` method lets you tie a user to their actions and record traits about them. This is useful for associating user data like name, email, and other attributes.
const Analytics = require('@segment/analytics-node');
const analytics = new Analytics('YOUR_WRITE_KEY');
analytics.identify({
userId: 'user123',
traits: {
name: 'John Doe',
email: 'john.doe@example.com'
}
});
Group
The `group` method allows you to associate an individual user with a group, such as a company or organization. This is useful for B2B applications where you need to track users within the context of their organization.
const Analytics = require('@segment/analytics-node');
const analytics = new Analytics('YOUR_WRITE_KEY');
analytics.group({
userId: 'user123',
groupId: 'group123',
traits: {
name: 'Company Inc.',
industry: 'Technology'
}
});
Page
The `page` method is used to record page views on your website. This is useful for tracking which pages your users are visiting.
const Analytics = require('@segment/analytics-node');
const analytics = new Analytics('YOUR_WRITE_KEY');
analytics.page({
userId: 'user123',
category: 'Docs',
name: 'Node.js SDK',
properties: {
url: 'https://example.com/docs/nodejs-sdk'
}
});
Alias
The `alias` method is used to merge two user identities, effectively linking an anonymous user with an identified user. This is useful for scenarios where a user initially interacts anonymously and later signs up or logs in.
const Analytics = require('@segment/analytics-node');
const analytics = new Analytics('YOUR_WRITE_KEY');
analytics.alias({
previousId: 'temp_user123',
userId: 'user123'
});
Mixpanel is an advanced analytics service that helps improve web and mobile applications by tracking how users interact and engage with them. It offers similar functionalities to @segment/analytics-node, such as tracking events, identifying users, and analyzing user behavior. However, Mixpanel is a standalone analytics platform, whereas Segment acts as a data hub that can route data to multiple analytics and marketing tools.
Amplitude is a product analytics platform that provides insights into user behavior and helps drive product strategy. Like @segment/analytics-node, it allows you to track events and user actions. Amplitude focuses more on in-depth product analytics and user behavior insights, while Segment provides a broader data routing and integration service.
Keen.io is a data analytics platform that allows you to collect, analyze, and visualize event data. It offers similar event tracking and user identification features as @segment/analytics-node. Keen.io is more focused on custom analytics and data visualization, whereas Segment provides a more comprehensive data integration and routing solution.
Warning: This library is in public beta ⚠️
https://www.npmjs.com/package/@segment/analytics-node
# npm
npm install @segment/analytics-node
# yarn
yarn add @segment/analytics-node
# pnpm
pnpm install @segment/analytics-node
Assuming some express-like web framework.
import { Analytics } from '@segment/analytics-node'
// or, if you use require:
const { Analytics } = require('@segment/analytics-node')
// instantiation
const analytics = new Analytics({ writeKey: '<MY_WRITE_KEY>' })
app.post('/login', (req, res) => {
analytics.identify({
userId: req.body.userId,
previousId: req.body.previousId
})
res.sendStatus(200)
})
app.post('/cart', (req, res) => {
analytics.track({
userId: req.body.userId,
event: 'Add to cart',
properties: { productId: '123456' }
})
res.sendStatus(201)
});
Avoid losing events on exit!
.closeAndFlush()
to stop collecting new events and flush all existing events.await analytics.closeAndFlush()
// or
await analytics.closeAndFlush({ timeout: 5000 }) // force resolve after 5000ms
const app = express()
const server = app.listen(3000)
const onExit = async () => {
await analytics.closeAndFlush()
server.close(() => {
console.log("Gracefully closing server...")
process.exit()
})
}
['SIGINT', 'SIGTERM'].forEach((code) => process.on(code, onExit))
If you absolutely need to preserve all possible events in the event of a forced timeout, even ones that came in after analytics.closeAndFlush()
was called, you can collect those events.
const unflushedEvents = []
analytics.on('call_after_close', (event) => unflushedEvents.push(events))
await analytics.closeAndFlush()
console.log(unflushedEvents) // all events that came in after closeAndFlush was called
See complete list of settings in the AnalyticsSettings interface.
const analytics = new Analytics({
writeKey: '<MY_WRITE_KEY>',
host: 'https://api.segment.io',
path: '/v1/batch',
maxRetries: 3,
maxEventsInBatch: 15,
flushInterval: 10000,
// ... and more!
})
For Business plans with access to Regional Segment, you can use the host configuration parameter to send data to the desired region:
Oregon (Default) — api.segment.io/v1 Dublin — events.eu1.segmentapis.com An example of setting the host to the EU endpoint using the Node library would be:
const analytics = new Analytics({
...
host: "https://events.eu1.segmentapis.com"
});
Our libraries are built to support high performance environments. That means it is safe to use our Node library on a web server that’s serving thousands of requests per second.
Every method you call does not result in an HTTP request, but is queued in memory instead. Messages are then flushed in batch in the background, which allows for much faster operation.
By default, our library will flush:
settings.maxEventsInBatch
).settings.flushInterval
)There is a maximum of 500KB per batch request and 32KB per call.
If you don’t want to batch messages, you can turn batching off by setting the maxEventsInBatch
setting to 1, like so:
const analytics = new Analytics({
...
maxEventsInBatch: 1
});
Batching means that your message might not get sent right away. But every method call takes an optional callback, which you can use to know when a particular message is flushed from the queue, like so:
analytics.track({
userId: '019mr8mf4r',
event: 'Ultimate Played',
},
(err, ctx) => {
...
}
)
Subscribe and log all event delivery errors.
const analytics = new Analytics({ writeKey: '<MY_WRITE_KEY>' })
analytics.on('error', (err) => console.error(err))
You can see the complete list of emitted events in emitter.ts
analytics.on('error', (err) => console.error(err))
analytics.on('identify', (ctx) => console.log(ctx))
analytics.on('track', (ctx) => console.log(ctx))
analytics.on('http_request', (event) => console.log(event))
// when triggered, emits an event of the shape:
{
url: 'https://api.segment.io/v1/batch',
method: 'POST',
headers: {
'Content-Type': 'application/json',
...
},
body: '...',
}
Different parts of your application may require different types of batching, or even sending to multiple Segment sources. In that case, you can initialize multiple instances of Analytics with different settings:
const marketingAnalytics = new Analytics({ writeKey: 'MARKETING_WRITE_KEY' });
const appAnalytics = new Analytics({ writeKey: 'APP_WRITE_KEY' });
Double check that you’ve followed all the steps in the Quick Start.
Make sure that you’re calling a Segment API method once the library is successfully installed: identify, track, etc.
Log events.
['initialize', 'call_after_close',
'screen', 'identify', 'group',
'track', 'ready', 'alias',
'page', 'error', 'register',
'deregister'].forEach((event) => analytics.on(event, console.log)
// Note: nock will _not_ work if polyfill fetch with something like undici, as nock uses the http module. Undici has its own interception method.
import nock from 'nock'
nock('https://api.segment.io')
.post('/v1/batch')
.reply(201)
.persist()
// old
import Analytics from 'analytics-node'
// new
import { Analytics } from '@segment/analytics-node'
// old
var analytics = new Analytics('YOUR_WRITE_KEY'); // not supported
// new!
const analytics = new Analytics({ writeKey: '<MY_WRITE_KEY>' })
// old
await analytics.flush(function(err, batch) {
console.log('Flushed, and now this program can exit!');
});
// new
await analytics.closeAndFlush()
Other Differences:
enable
configuration option has been removed-- see "Disabling Analytics" sectionerrorHandler
configuration option has been remove -- see "Error Handling" sectionflushAt
configuration option -> maxEventsInBatch
.callback
call signature is different// old
(err, batch) => void
// new
(err, ctx) => void
track
properties have been removed. // old, undocumented behavior
analytics.track({
...
event: 'Ultimate Played',
myProp: 'abc'
})
// new
analytics.track({
...
event: 'Ultimate Played',
properties: {
myProp: 'abc'
}
})
import type { Plugin } from '@segment/analytics-node'
export const lowercase: Plugin = {
name: 'Lowercase events',
type: 'enrichment',
version: '1.0.0',
isLoaded: () => true,
load: () => Promise.resolve(),
track: (ctx) => {
ctx.event.event = ctx.event.event.toLowerCase()
return ctx
}
}
analytics.register(lowercase)
The alias, group, identify, page and track calls can all be passed an object of integrations that lets you turn certain destinations on or off. By default all destinations are enabled.
Here’s an example with the integrations object shown:
analytics.track({
event: 'Membership Upgraded',
userId: '97234974',
integrations: {
'All': false,
'Vero': true,
'Google Analytics': false
}
})
In this case, we’re specifying that we want this track to only go to Vero. All: false says that no destination should be enabled unless otherwise specified. Vero: true turns on Vero, etc.
Destination flags are case sensitive and match the destination’s name in the docs (i.e. “AdLearn Open Platform”, “awe.sm”, “MailChimp”, etc.). In some cases, there may be several names for a destination; if that happens you’ll see a “Adding (destination name) to the Integrations Object” section in the destination’s doc page with a list of valid names.
Note:
Available at the business level, filtering track calls can be done right from the Segment UI on your source schema page. We recommend using the UI if possible since it’s a much simpler way of managing your filters and can be updated with no code changes on your side.
If you are on a grandfathered plan, events sent server-side that are filtered through the Segment dashboard will still count towards your API usage.
Here is an example of using analytics.js within a handler:
const { Analytics } = require("@segment/analytics-node");
// since analytics has the potential to be stateful if there are any plugins added,
// to be on the safe side, we should instantiate a new instance of analytics on every request (the cost of instantiation is low).
const analytics = () => new Analytics({
maxEventsInBatch: 1,
writeKey: '<MY_WRITE_KEY>',
})
.on("error", console.error);
module.exports.handler = async (event) => {
...
// we need to await before returning, otherwise the lambda will exit before sending the request.
await new Promise((resolve) =>
analytics().track({ event: 'My Event', anonymousId: 'foo' }, resolve)
)
return {
statusCode: 200,
};
....
};
FAQs
https://www.npmjs.com/package/@segment/analytics-node
The npm package @segment/analytics-node receives a total of 302,287 weekly downloads. As such, @segment/analytics-node popularity was classified as popular.
We found that @segment/analytics-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 286 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.