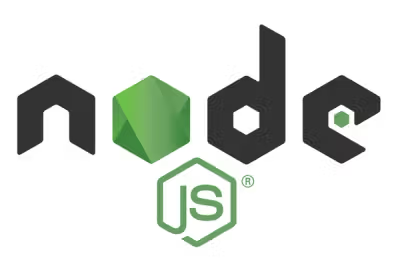
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
@semantic-ui/utils
Advanced tools
**Utils is a tiny 3kb library for simplifying common javascript boilerplate.**
Utils is a tiny 3kb library for simplifying common javascript boilerplate.
Utils has three primary advantages over custom implementations.
Tree Shaking - Many components will need to do similar things. When these components are bundled together its more efficient for them to reference a single code implementation than several unique ones. This means smaller bundled size when using more than one component together in a bundle.
Code Readability - Utility libraries offer a level of familiarity and consistency that can be missing with native ECMAScript features. Removing rough edges reduces the learning curve when looking at unfamiliar code so you can focus on the intention not the implementation.
Gotcha Handling - More Robust Implementations: When using utility libraries for common operations like object manipulation, you're not just avoiding gotchas (consider non-enumerated properties with object manipulation like extend/clone.); you're also leveraging a community-tested solution. These libraries often include safeguards against edge cases and peculiarities of JavaScript that a typical custom implementation might overlook. This results in more robust code, reducing the likelihood of bugs related to subtle language intricacies.
Utility includes the following helpers:
unique(arr)
- Removes duplicates from arraysfilterEmpty(arr)
- Removes falsey values from an arraylast(array, number)
- Returns last (n) elements from arrayfirst(array, number)
- Returns first (n) elements from arrayfirstMatch(array, callback)
- Returns the first value that matches the provided callback functionfindIndex(array, callback)
- Finds the index of the first element in the array that satisfies the provided callback functionremove(array, callbackOrValue)
- Removes elements from an array that match the given callback function or valueinArray(value, array)
- Returns whether a value is present in an arrayrange(start, stop, step)
- Creates an array of numbers progressing from start up to, but not including, endwhere(array, properties)
- Returns only objs with given properties in an array of objectsflatten(arr)
- Flattens an array of arrayssome/any(arr, truthFunc)
- Returns true if any/some values match truthFuncsum(values)
- Sums an array of numbersmoveItem(array, callbackOrValue, index)
- Moves element to specified index (supports 'first'/'last')moveToFront(array, callbackOrValue)
- Moves element to start of arraymoveToBack(array, callbackOrValue)
- Moves element to end of arraysortBy(array, key, comparator)
- Sort array by object key with optional comparatorgroupBy(array, property)
- Group array items by property valueroundNumber(number, digits)
- Rounds a number to given significant digitscopyText(text)
- Copies text to clipboardopenLink(url, options)
- Opens a link with configurable optionsgetText(src)
- Fetches text content from a URLgetJSON(src)
- Fetches and parses JSON from a URLgetKeyFromEvent(event)
- Extracts standardized key from keyboard eventkeys(obj)
- Return the keys of an objectvalues(obj)
- Return the values of an objectmapObject(obj, callback)
- Creates an object with transformed valuesfilterObject(obj, callback)
- Creates object with filtered key-value pairsextend(obj, ...sources)
- Extends an object with properties from additional sourcespick(obj, ...keys)
- Creates an object composed of the picked object propertiesget(obj, string)
- Access a nested object field with a string, like 'a.b.c'onlyKeys(obj, keysToKeep)
- Returns an object with only specified keyshasProperty(obj, prop)
- Return true if the object has the specified propertyreverseKeys(obj)
- Reverses a lookup object's keys and valuesarrayFromObject(obj)
- Returns array with key value pairs from objectproxyObject(sourceObj, referenceObj)
- Creates a proxy combining source and reference objectsweightedObjectSearch(query, array, options)
- Performs weighted search across object arrayisObject(x)
- Checks if the value is an objectisPlainObject(x)
- Checks if the value is a plain objectisString(x)
- Checks if the value is a stringisBoolean(x)
- Checks if the value is a booleanisNumber(x)
- Checks if the value is a numberisArray(x)
- Checks if the value is an arrayisBinary(x)
- Checks if the value is binary (Uint8Array)isFunction(x)
- Checks if the value is a functionisPromise(x)
- Checks if the value is a promiseisArguments(obj)
- Checks if the value is an arguments objectisDOM(x)
- Checks if the value is a DOM elementisNode(x)
- Checks if the value is a DOM nodeisEmpty(x)
- Checks if the value is empty like {}isClassInstance(x)
- Checks if value is instance of custom classformatDate(date, format)
- Formats a date object into a string based on the provided formatnoop()
- A no-operation function for use as a default callbackwrapFunction(x)
- Wraps a value in a functionmemoize(fn, hashFunction)
- Memoizes a function with optional custom cache key generationdebounce(fn, options)
- Creates a debounced version of a functionkebabToCamel(str)
- Converts kebab-case to camelCasecamelToKebab(str)
- Converts camelCase to kebab-casecapitalize(str)
- Capitalize only the first wordcapitalizeWords(str)
- Capitalizes each wordtoTitleCase(str)
- Converts string to title casejoinWords(words, options)
- Joins words with configurable formattinggetArticle(word, settings)
- Gets appropriate indefinite article (a/an)escapeRegExp(string)
- Escapes special characters for use in a regular expressionescapeHTML(string)
- Escapes string for html '&<>"' onlyeach(iterable, func, context)
- Calls function for each element of an iterableasyncEach(iterable, func, context)
- Calls iteratee awaiting each functionasyncMap(iterable, func, context)
- Calls each func awaiting the mapped resultisEqual(a, b)
- Deep compares values for equivalenceclone(a)
- Performant clone of Array, Object, Date, RegExp, Map, Set or other datatokenize(str)
- Returns a tokenized version of a stringprettifyID(num)
- Converts numeric ID to human-readable stringhashCode(input, options)
- Creates a hash code from inputgenerateID()
- Generates a pseudo-random unique identifierfatal(message, options)
- Throws a custom error asynchronouslyisServer
- Boolean whether code is running on serverisClient
- Boolean whether code is running on clientFAQs
**Utils is a tiny 3kb library for simplifying common javascript boilerplate.**
The npm package @semantic-ui/utils receives a total of 89 weekly downloads. As such, @semantic-ui/utils popularity was classified as not popular.
We found that @semantic-ui/utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.