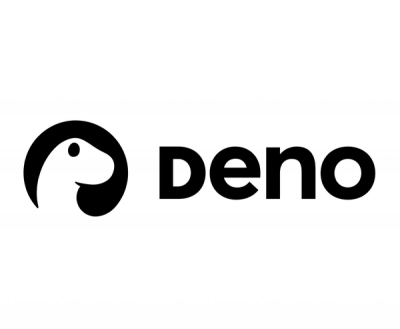
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@sentry/browser
Advanced tools
The @sentry/browser package is a JavaScript SDK for Sentry, which is an error tracking service that helps developers monitor and fix crashes in real time. The SDK hooks into the global error handling mechanisms of the browser and captures unhandled exceptions and other errors, providing rich context and insights into the underlying issues.
Error Tracking
This feature automatically captures JavaScript exceptions and sends them to Sentry for analysis and tracking. The code initializes Sentry with a DSN (Data Source Name) and sets up a global error handler to capture exceptions.
Sentry.init({ dsn: 'YOUR_DSN' });
window.onerror = function (errorMsg, url, lineNumber, column, errorObj) {
Sentry.captureException(errorObj);
return false;
}
Manual Error Reporting
This allows developers to manually report exceptions or errors to Sentry. The code block demonstrates how to catch an error in a try-catch block and manually send it to Sentry using the captureException method.
Sentry.init({ dsn: 'YOUR_DSN' });
try {
// Code that might fail
} catch (error) {
Sentry.captureException(error);
}
Performance Monitoring
This feature enables performance monitoring by capturing transactions and spans. The code initializes Sentry with a sample rate for transactions and demonstrates how to start and finish a transaction, which can be used to measure the performance of operations.
Sentry.init({ dsn: 'YOUR_DSN', tracesSampleRate: 1.0 });
const transaction = Sentry.startTransaction({ name: 'test transaction' });
// ... some code ...
transaction.finish();
Breadcrumbs
Breadcrumbs are a trail of events that happened prior to an issue. This feature allows you to record breadcrumbs manually. The code shows how to add a breadcrumb for an authentication event.
Sentry.init({ dsn: 'YOUR_DSN' });
Sentry.addBreadcrumb({
category: 'auth',
message: 'User authenticated',
level: Sentry.Severity.Info
});
User Feedback
This feature allows you to collect user feedback when an error occurs. The code initializes Sentry and then shows a dialog to the user to collect feedback for a specific event.
Sentry.init({ dsn: 'YOUR_DSN' });
Sentry.showReportDialog({ eventId: 'event_id' });
Bugsnag provides similar error tracking and monitoring capabilities as Sentry. It offers real-time error reporting and can be used to monitor application stability. Bugsnag also provides features for error diagnostics and supports multiple platforms.
Raygun is another error monitoring service that offers crash reporting with detailed diagnostics. It supports real-time error tracking and performance monitoring, and it can be used to identify and diagnose software errors and performance issues.
Rollbar offers real-time error tracking and is similar to Sentry in its capabilities. It includes features like telemetry, person tracking, and version tracking. Rollbar provides a comprehensive dashboard for monitoring and analyzing errors.
To use this SDK, call SentryClient.create(options)
as early as possible after
loading the page. This will initialize the SDK and hook into the environment.
Note that you can turn off almost all side effects using the respective options.
import { SentryClient } from '@sentry/browser';
SentryClient.create({
dsn: '__DSN__',
// ...
});
To set context information or send manual events, use the provided methods on
SentryClient
. Note that these functions will not perform any action before you
have called SentryClient.install()
:
// Set user information, as well as tags and further extras
SentryClient.setContext({
extra: { battery: 0.7 },
tags: { user_mode: 'admin' },
user: { id: '4711' },
});
// Add a breadcrumb for future events
SentryClient.addBreadcrumb({
message: 'My Breadcrumb',
// ...
});
// Capture exceptions, messages or manual events
SentryClient.captureMessage('Hello, world!');
SentryClient.captureException(new Error('Good bye'));
SentryClient.captureEvent({
message: 'Manual',
stacktrace: [
// ...
],
});
If you don't want to use a global static instance of Sentry, you can create one yourself:
import { BrowserFrontend } from '@sentry/browser';
const client = new BrowserFrontend({
dsn: '__DSN__',
// ...
});
client.install();
// ...
Note that install()
returns a Promise
that resolves when the installation
has finished. It is not necessary to wait for the installation before adding
breadcrumbs, defining context or sending events. However, the return value
indicates whether the installation was successful and the environment could be
instrumented:
import { BrowserFrontend } from '@sentry/browser';
const client = new BrowserFrontend({
dsn: '__DSN__',
// ...
});
const success = await client.install();
if (success) {
// Will catch global exceptions, record breadcrumbs for DOM events, etc...
} else {
// Limited instrumentation, but sending events will still work
}
FAQs
Official Sentry SDK for browsers
The npm package @sentry/browser receives a total of 5,887,192 weekly downloads. As such, @sentry/browser popularity was classified as popular.
We found that @sentry/browser demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.