Serenity/JS
Serenity/JS is a framework designed to make acceptance and regression testing
of modern full-stack applications faster, more collaborative and easier to scale.
Visit serenity-js.org for the latest tutorials
and API docs, and follow @SerenityJS and @JanMolak on Twitter for project updates.
Learning Serenity/JS
To learn more about Serenity/JS, check out the video below, read the tutorial, review the examples, and create your own test suite with Serenity/JS template projects.
If you have any questions, join us on Serenity/JS Community Chat.
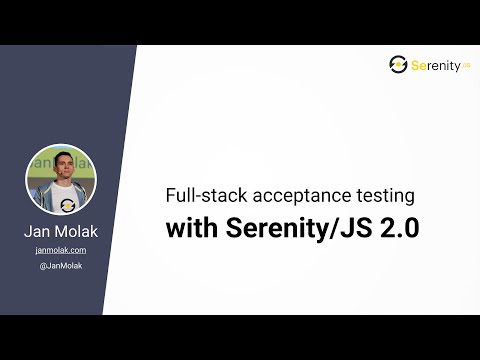
Serenity/JS Assertions
@serenity-js/assertions
is an assertions library implementing the Screenplay Pattern.
Installation
To install this module, run the following command in your computer terminal:
npm install --save-dev @serenity-js/{core,assertions}
Performing verifications using Ensure
import { Ensure, endsWith } from '@serenity-js/assertions';
import { actorCalled } from '@serenity-js/core';
import { Navigate, Page } from '@serenity-js/web';
await actorCalled('Erica').attemptsTo(
Navigate.to('https://serenity-js.org'),
Ensure.that(
Page.current().title(),
endsWith('Serenity/JS')
),
);
Controlling execution flow using Check
import { actorCalled } from '@serenity-js/core';
import { Check } from '@serenity-js/assertions';
import { Click, isVisible } from '@serenity-js/protractor';
await actorCalled('Erica').attemptsTo(
Check.whether(NewsletterModal, isVisible())
.andIfSo(Click.on(CloseModalButton)),
);
Synchronising the test with the System Under Test using Wait
import { actorCalled } from '@serenity-js/core';
import { Click, isVisible, Wait } from '@serenity-js/protractor';
await actorCalled('Erica').attemptsTo(
Wait.until(CloseModalButton, isVisible()),
Click.on(CloseModalButton)
);
Defining custom expectations using Expectation.thatActualShould
import { actorCalled } from '@serenity-js/core';
import { Expectation, Ensure } from '@serenity-js/assertions';
function isDivisibleBy(expected: Answerable<number>): Expectation<number> {
return Expectation.thatActualShould<number, number>('have value divisible by', expected)
.soThat((actualValue, expectedValue) => actualValue % expectedValue === 0);
}
await actorCalled('Erica').attemptsTo(
Ensure.that(4, isDivisibleBy(2)),
);
Composing expectations using Expectation.to
import { actorCalled } from '@serenity-js/core';
import { Expectation, Ensure, and, or, isGreaterThan, isLessThan, equals } from '@serenity-js/assertions';
function isWithin(lowerBound: number, upperBound: number) {
return Expectation
.to(`have value within ${ lowerBound } and ${ upperBound }`)
.soThatActual(and(
or(isGreaterThan(lowerBound), equals(lowerBound)),
or(isLessThan(upperBound), equals(upperBound)),
));
}
await actorCalled('Erica').attemptsTo(
Ensure.that(5, isWithin(3, 6)),
);