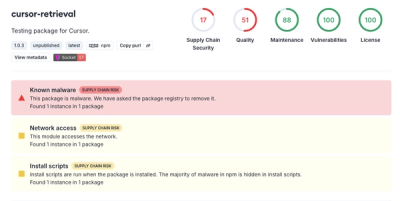
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@settlemint/sdk-utils
Advanced tools
SettleMint SDK, integrate SettleMint into your application with ease.
✨ https://settlemint.com ✨
Integrate SettleMint into your application with ease.
The SettleMint Utils SDK provides a collection of shared utilities and helper functions used across the SettleMint SDK packages. It includes common functionality for configuration management, error handling, validation, and type definitions that ensure consistency and reliability across the SDK ecosystem.
ascii():
void
Defined in: sdk/utils/src/terminal/ascii.ts:13
Prints the SettleMint ASCII art logo to the console in magenta color. Used for CLI branding and visual identification.
void
import { ascii } from "@settlemint/sdk-utils";
// Prints the SettleMint logo
ascii();
cancel(
msg
):never
Defined in: sdk/utils/src/terminal/cancel.ts:17
Displays an error message in red inverse text and exits the process. Used to terminate execution with a visible error message. Any sensitive tokens in the message are masked before display.
Parameter | Type | Description |
---|---|---|
msg | string | The error message to display |
never
never - Function does not return as it exits the process
import { cancel } from "@settlemint/sdk-utils";
// Exits process with error message
cancel("An error occurred");
capitalizeFirstLetter(
val
):string
Defined in: sdk/utils/src/string.ts:13
Capitalizes the first letter of a string.
Parameter | Type | Description |
---|---|---|
val | string | The string to capitalize |
string
The input string with its first letter capitalized
import { capitalizeFirstLetter } from "@settlemint/sdk-utils";
const capitalized = capitalizeFirstLetter("hello");
// Returns: "Hello"
emptyDir(
dir
):Promise
<void
>
Defined in: sdk/utils/src/package-manager/download-and-extract.ts:53
Removes all contents of a directory except the .git folder
Parameter | Type | Description |
---|---|---|
dir | string | The directory path to empty |
Promise
<void
>
import { emptyDir } from "@settlemint/sdk-utils";
await emptyDir("/path/to/dir"); // Removes all contents except .git
ensureBrowser():
void
Defined in: sdk/utils/src/runtime/ensure-server.ts:31
Ensures that code is running in a browser environment and not on the server.
void
If called from a server environment
import { ensureBrowser } from "@settlemint/sdk-utils";
// Will throw if running on server
ensureBrowser();
ensureServer():
void
Defined in: sdk/utils/src/runtime/ensure-server.ts:13
Ensures that code is running on the server and not in a browser environment.
void
If called from a browser environment
import { ensureServer } from "@settlemint/sdk-utils";
// Will throw if running in browser
ensureServer();
executeCommand(
command
,args
,options
?):Promise
<string
[]>
Defined in: sdk/utils/src/terminal/execute-command.ts:31
Executes a command with the given arguments in a child process. Pipes stdin to the child process and captures stdout/stderr output. Masks any sensitive tokens in the output before displaying or returning.
Parameter | Type | Description |
---|---|---|
command | string | The command to execute |
args | string [] | Array of arguments to pass to the command |
options ? | ExecuteCommandOptions | Options for customizing command execution |
Promise
<string
[]>
Array of output strings from stdout and stderr
If the process fails to start or exits with non-zero code
import { executeCommand } from "@settlemint/sdk-utils";
// Execute git clone
await executeCommand("git", ["clone", "repo-url"]);
// Execute silently
await executeCommand("npm", ["install"], { silent: true });
exists(
path
):Promise
<boolean
>
Defined in: sdk/utils/src/filesystem/exists.ts:17
Checks if a file or directory exists at the given path
Parameter | Type | Description |
---|---|---|
path | PathLike | The file system path to check for existence |
Promise
<boolean
>
Promise that resolves to true if the path exists, false otherwise
import { exists } from "@settlemint/sdk-utils";
// Check if file exists before reading
if (await exists('/path/to/file.txt')) {
// File exists, safe to read
}
formatTargetDir(
targetDir
):string
Defined in: sdk/utils/src/package-manager/download-and-extract.ts:23
Formats a directory path by removing trailing slashes and whitespace
Parameter | Type | Description |
---|---|---|
targetDir | string | The directory path to format |
string
The formatted directory path
import { formatTargetDir } from "@settlemint/sdk-utils";
const formatted = formatTargetDir("/path/to/dir/ "); // "/path/to/dir"
getPackageManager(
targetDir
?):Promise
<AgentName
>
Defined in: sdk/utils/src/package-manager/get-package-manager.ts:15
Detects the package manager used in the current project
Parameter | Type | Description |
---|---|---|
targetDir ? | string | The directory to check for package manager (optional, defaults to process.cwd()) |
Promise
<AgentName
>
The name of the package manager
import { getPackageManager } from "@settlemint/sdk-utils";
const packageManager = await getPackageManager();
console.log(`Using ${packageManager}`);
getPackageManagerExecutable(
targetDir
?):Promise
<{args
:string
[];command
:string
; }>
Defined in: sdk/utils/src/package-manager/get-package-manager-executable.ts:14
Retrieves the executable command and arguments for the package manager
Parameter | Type | Description |
---|---|---|
targetDir ? | string | The directory to check for package manager (optional, defaults to process.cwd()) |
Promise
<{ args
: string
[]; command
: string
; }>
An object containing the command and arguments for the package manager
import { getPackageManagerExecutable } from "@settlemint/sdk-utils";
const { command, args } = await getPackageManagerExecutable();
console.log(`Using ${command} with args: ${args.join(" ")}`);
installDependencies(
pkgs
):Promise
<void
>
Defined in: sdk/utils/src/package-manager/install-dependencies.ts:18
Installs one or more packages as dependencies using the detected package manager
Parameter | Type | Description |
---|---|---|
pkgs | string | string [] | A single package name or array of package names to install |
Promise
<void
>
A promise that resolves when installation is complete
If package installation fails
import { installDependencies } from "@settlemint/sdk-utils";
// Install a single package
await installDependencies("express");
// Install multiple packages
await installDependencies(["express", "cors"]);
intro(
msg
):void
Defined in: sdk/utils/src/terminal/intro.ts:15
Displays an introductory message in magenta text with padding. Any sensitive tokens in the message are masked before display.
Parameter | Type | Description |
---|---|---|
msg | string | The message to display as introduction |
void
import { intro } from "@settlemint/sdk-utils";
// Display intro message
intro("Starting deployment...");
isEmpty(
path
):Promise
<boolean
>
Defined in: sdk/utils/src/package-manager/download-and-extract.ts:39
Checks if a directory is empty or contains only a .git folder
Parameter | Type | Description |
---|---|---|
path | string | The directory path to check |
Promise
<boolean
>
True if directory is empty or contains only .git, false otherwise
import { isEmpty } from "@settlemint/sdk-utils";
if (await isEmpty("/path/to/dir")) {
// Directory is empty
}
isPackageInstalled(
name
,path
?):Promise
<boolean
>
Defined in: sdk/utils/src/package-manager/is-package-installed.ts:12
Checks if a package is installed in the project's dependencies, devDependencies, or peerDependencies.
Parameter | Type | Description |
---|---|---|
name | string | The name of the package to check |
path ? | string | The path to the project root directory. If not provided, will be automatically determined |
Promise
<boolean
>
Whether the package is installed
If unable to read or parse the package.json file
loadEnv<
T
>(validateEnv
,prod
,path
):Promise
<T
extendstrue
?DotEnv
:DotEnvPartial
>
Defined in: sdk/utils/src/environment/load-env.ts:25
Loads environment variables from .env files.
To enable encryption with dotenvx (https://www.dotenvx.com/docs) run bunx dotenvx encrypt
Type Parameter | Default type |
---|---|
T extends boolean | true |
Parameter | Type | Description |
---|---|---|
validateEnv | T | Whether to validate the environment variables against the schema |
prod | boolean | Whether to load production environment variables |
path | string | Optional path to the directory containing .env files. Defaults to process.cwd() |
Promise
<T
extends true
? DotEnv
: DotEnvPartial
>
A promise that resolves to the validated environment variables
Will throw an error if validation fails and validateEnv is true
import { loadEnv } from '@settlemint/sdk-utils';
// Load and validate environment variables
const env = await loadEnv(true, false);
console.log(env.SETTLEMINT_INSTANCE);
// Load without validation
const rawEnv = await loadEnv(false, false);
maskTokens(
output
):string
Defined in: sdk/utils/src/terminal/mask-tokens.ts:13
Masks sensitive SettleMint tokens in output text by replacing them with asterisks. Handles personal access tokens (PAT), application access tokens (AAT), and service account tokens (SAT).
Parameter | Type | Description |
---|---|---|
output | string | The text string that may contain sensitive tokens |
string
The text with any sensitive tokens masked with asterisks
import { maskTokens } from "@settlemint/sdk-utils";
// Masks a token in text
const masked = maskTokens("Token: sm_pat_****"); // "Token: ***"
note(
message
,level
):void
Defined in: sdk/utils/src/terminal/note.ts:20
Displays a note message with optional warning level formatting. Regular notes are displayed in normal text, while warnings are shown in yellow. Any sensitive tokens in the message are masked before display.
Parameter | Type | Default value | Description |
---|---|---|---|
message | string | undefined | The message to display as a note |
level | "info" | "warn" | "info" | The note level: "info" (default) or "warn" for warning styling |
void
import { note } from "@settlemint/sdk-utils";
// Display info note
note("Operation completed successfully");
// Display warning note
note("Low disk space remaining", "warn");
outro(
msg
):void
Defined in: sdk/utils/src/terminal/outro.ts:15
Displays a closing message in green inverted text with padding. Any sensitive tokens in the message are masked before display.
Parameter | Type | Description |
---|---|---|
msg | string | The message to display as conclusion |
void
import { outro } from "@settlemint/sdk-utils";
// Display outro message
outro("Deployment completed successfully!");
projectRoot():
Promise
<string
>
Defined in: sdk/utils/src/filesystem/project-root.ts:16
Finds the root directory of the current project by locating the nearest package.json file
Promise
<string
>
Promise that resolves to the absolute path of the project root directory
Will throw an error if no package.json is found in the directory tree
import { projectRoot } from "@settlemint/sdk-utils";
// Get project root path
const rootDir = await projectRoot();
console.log(`Project root is at: ${rootDir}`);
setName(
name
,path
?):Promise
<void
>
Defined in: sdk/utils/src/package-manager/set-name.ts:16
Sets the name field in the package.json file
Parameter | Type | Description |
---|---|---|
name | string | The new name to set in the package.json file |
path ? | string | The path to the project root directory. If not provided, will be automatically determined |
Promise
<void
>
A promise that resolves when the package.json has been updated
If unable to read, update or save the package.json file
import { setName } from "@settlemint/sdk-utils";
await setName("my-new-project-name");
spinner<
R
>(options
):Promise
<R
>
Defined in: sdk/utils/src/terminal/spinner.ts:39
Displays a loading spinner while executing an async task. Shows progress with start/stop messages and handles errors. Spinner is disabled in CI environments.
Type Parameter |
---|
R |
Parameter | Type | Description |
---|---|---|
options | SpinnerOptions <R > | Configuration options for the spinner |
Promise
<R
>
The result from the executed task
Will exit process with code 1 if task fails
import { spinner } from "@settlemint/sdk-utils";
// Show spinner during async task
const result = await spinner({
startMessage: "Deploying...",
task: async () => {
// Async work here
return "success";
},
stopMessage: "Deployed successfully!"
});
tryParseJson<
T
>(value
,defaultValue
):T
|null
Defined in: sdk/utils/src/json.ts:23
Attempts to parse a JSON string into a typed value, returning a default value if parsing fails.
Type Parameter |
---|
T |
Parameter | Type | Default value | Description |
---|---|---|---|
value | string | undefined | The JSON string to parse |
defaultValue | null | T | null | The value to return if parsing fails or results in null/undefined |
T
| null
The parsed JSON value as type T, or the default value if parsing fails
import { tryParseJson } from "@settlemint/sdk-utils";
const config = tryParseJson<{ port: number }>(
'{"port": 3000}',
{ port: 8080 }
);
// Returns: { port: 3000 }
const invalid = tryParseJson<string[]>(
'invalid json',
[]
);
// Returns: []
validate<
T
>(schema
,value
):T
["_output"
]
Defined in: sdk/utils/src/validation.ts:14
Validates a value against a given Zod schema.
Type Parameter |
---|
T extends ZodType |
Parameter | Type | Description |
---|---|---|
schema | T | The Zod schema to validate against. |
value | unknown | The value to validate. |
T
["_output"
]
The validated and parsed value.
Will throw an error if validation fails, with formatted error messages.
const validatedId = validate(IdSchema, "550e8400-e29b-41d4-a716-446655440000");
writeEnv(
prod
,env
,secrets
):Promise
<void
>
Defined in: sdk/utils/src/environment/write-env.ts:81
Writes environment variables to .env files across a project or monorepo
Parameter | Type | Description |
---|---|---|
prod | boolean | Whether to write production environment variables |
env | Partial <{ SETTLEMINT_ACCESS_TOKEN : string ; SETTLEMINT_APPLICATION : string ; SETTLEMINT_BLOCKCHAIN_NETWORK : string ; SETTLEMINT_BLOCKCHAIN_NODE : string ; SETTLEMINT_BLOCKSCOUT : string ; SETTLEMINT_BLOCKSCOUT_GRAPHQL_ENDPOINT : string ; SETTLEMINT_BLOCKSCOUT_UI_ENDPOINT : string ; SETTLEMINT_CUSTOM_DEPLOYMENT : string ; SETTLEMINT_CUSTOM_DEPLOYMENT_ENDPOINT : string ; SETTLEMINT_HASURA : string ; SETTLEMINT_HASURA_ADMIN_SECRET : string ; SETTLEMINT_HASURA_DATABASE_URL : string ; SETTLEMINT_HASURA_ENDPOINT : string ; SETTLEMINT_HD_PRIVATE_KEY : string ; SETTLEMINT_INSTANCE : string ; SETTLEMINT_IPFS : string ; SETTLEMINT_IPFS_API_ENDPOINT : string ; SETTLEMINT_IPFS_GATEWAY_ENDPOINT : string ; SETTLEMINT_IPFS_PINNING_ENDPOINT : string ; SETTLEMINT_LOAD_BALANCER : string ; SETTLEMINT_MINIO : string ; SETTLEMINT_MINIO_ACCESS_KEY : string ; SETTLEMINT_MINIO_ENDPOINT : string ; SETTLEMINT_MINIO_SECRET_KEY : string ; SETTLEMINT_NEW_PROJECT_NAME : string ; SETTLEMINT_PERSONAL_ACCESS_TOKEN : string ; SETTLEMINT_PORTAL : string ; SETTLEMINT_PORTAL_GRAPHQL_ENDPOINT : string ; SETTLEMINT_PORTAL_REST_ENDPOINT : string ; SETTLEMINT_SMART_CONTRACT_ADDRESS : string ; SETTLEMINT_SMART_CONTRACT_DEPLOYMENT_ID : string ; SETTLEMINT_THEGRAPH : string ; SETTLEMINT_THEGRAPH_SUBGRAPH_NAME : string ; SETTLEMINT_THEGRAPH_SUBGRAPHS_ENDPOINTS : string []; SETTLEMINT_WORKSPACE : string ; }> | The environment variables to write |
secrets | boolean | Whether to write to .env.local files for secrets |
Promise
<void
>
Promise that resolves when writing is complete
Will throw an error if writing fails
import { writeEnv } from '@settlemint/sdk-utils';
// Write development environment variables
await writeEnv(false, {
SETTLEMINT_INSTANCE: 'https://dev.example.com'
}, false);
// Write production secrets
await writeEnv(true, {
SETTLEMINT_ACCESS_TOKEN: 'secret-token'
}, true);
Defined in: sdk/utils/src/terminal/execute-command.ts:7
Options for executing a command, extending SpawnOptionsWithoutStdio
SpawnOptionsWithoutStdio
Property | Type | Description | Defined in |
---|---|---|---|
silent? | boolean | Whether to suppress output to stdout/stderr | sdk/utils/src/terminal/execute-command.ts:9 |
Defined in: sdk/utils/src/terminal/spinner.ts:9
Options for configuring the spinner behavior
Type Parameter |
---|
R |
Property | Type | Description | Defined in |
---|---|---|---|
startMessage | string | Message to display when spinner starts | sdk/utils/src/terminal/spinner.ts:11 |
stopMessage | string | Message to display when spinner completes successfully | sdk/utils/src/terminal/spinner.ts:15 |
task | () => Promise <R > | Async task to execute while spinner is active | sdk/utils/src/terminal/spinner.ts:13 |
AccessToken:
string
Defined in: sdk/utils/src/validation/access-token.schema.ts:22
Schema for validating both application and personal access tokens. Accepts tokens starting with either 'sm_pat_' or 'sm_aat_' prefix.
ApplicationAccessToken:
string
Defined in: sdk/utils/src/validation/access-token.schema.ts:8
Schema for validating application access tokens. Application access tokens start with 'sm_aat_' prefix.
DotEnv:
object
Defined in: sdk/utils/src/validation/dot-env.schema.ts:91
Type definition for the environment variables schema.
Name | Type | Description | Defined in |
---|---|---|---|
SETTLEMINT_ACCESS_TOKEN ? | string | Application access token for authenticating with SettleMint services | sdk/utils/src/validation/dot-env.schema.ts:16 |
SETTLEMINT_APPLICATION ? | string | Unique name of the application | sdk/utils/src/validation/dot-env.schema.ts:22 |
SETTLEMINT_BLOCKCHAIN_NETWORK ? | string | Unique name of the blockchain network | sdk/utils/src/validation/dot-env.schema.ts:24 |
SETTLEMINT_BLOCKCHAIN_NODE ? | string | Unique name of the blockchain node | sdk/utils/src/validation/dot-env.schema.ts:26 |
SETTLEMINT_BLOCKSCOUT ? | string | Unique name of the Blockscout instance | sdk/utils/src/validation/dot-env.schema.ts:75 |
SETTLEMINT_BLOCKSCOUT_GRAPHQL_ENDPOINT ? | string | GraphQL endpoint URL for Blockscout | sdk/utils/src/validation/dot-env.schema.ts:77 |
SETTLEMINT_BLOCKSCOUT_UI_ENDPOINT ? | string | UI endpoint URL for Blockscout | sdk/utils/src/validation/dot-env.schema.ts:79 |
SETTLEMINT_CUSTOM_DEPLOYMENT ? | string | Unique name of the custom deployment | sdk/utils/src/validation/dot-env.schema.ts:71 |
SETTLEMINT_CUSTOM_DEPLOYMENT_ENDPOINT ? | string | Endpoint URL for the custom deployment | sdk/utils/src/validation/dot-env.schema.ts:73 |
SETTLEMINT_HASURA ? | string | Unique name of the Hasura instance | sdk/utils/src/validation/dot-env.schema.ts:30 |
SETTLEMINT_HASURA_ADMIN_SECRET ? | string | Admin secret for authenticating with Hasura | sdk/utils/src/validation/dot-env.schema.ts:34 |
SETTLEMINT_HASURA_DATABASE_URL ? | string | Database connection URL for Hasura | sdk/utils/src/validation/dot-env.schema.ts:36 |
SETTLEMINT_HASURA_ENDPOINT ? | string | Endpoint URL for the Hasura GraphQL API | sdk/utils/src/validation/dot-env.schema.ts:32 |
SETTLEMINT_HD_PRIVATE_KEY ? | string | Unique name of the HD private key | sdk/utils/src/validation/dot-env.schema.ts:53 |
SETTLEMINT_INSTANCE | string | Base URL of the SettleMint platform instance | sdk/utils/src/validation/dot-env.schema.ts:14 |
SETTLEMINT_IPFS ? | string | Unique name of the IPFS instance | sdk/utils/src/validation/dot-env.schema.ts:63 |
SETTLEMINT_IPFS_API_ENDPOINT ? | string | API endpoint URL for IPFS | sdk/utils/src/validation/dot-env.schema.ts:65 |
SETTLEMINT_IPFS_GATEWAY_ENDPOINT ? | string | Gateway endpoint URL for IPFS | sdk/utils/src/validation/dot-env.schema.ts:69 |
SETTLEMINT_IPFS_PINNING_ENDPOINT ? | string | Pinning service endpoint URL for IPFS | sdk/utils/src/validation/dot-env.schema.ts:67 |
SETTLEMINT_LOAD_BALANCER ? | string | Unique name of the load balancer | sdk/utils/src/validation/dot-env.schema.ts:28 |
SETTLEMINT_MINIO ? | string | Unique name of the MinIO instance | sdk/utils/src/validation/dot-env.schema.ts:55 |
SETTLEMINT_MINIO_ACCESS_KEY ? | string | Access key for MinIO authentication | sdk/utils/src/validation/dot-env.schema.ts:59 |
SETTLEMINT_MINIO_ENDPOINT ? | string | Endpoint URL for MinIO | sdk/utils/src/validation/dot-env.schema.ts:57 |
SETTLEMINT_MINIO_SECRET_KEY ? | string | Secret key for MinIO authentication | sdk/utils/src/validation/dot-env.schema.ts:61 |
SETTLEMINT_NEW_PROJECT_NAME ? | string | Name of the new project being created | sdk/utils/src/validation/dot-env.schema.ts:81 |
SETTLEMINT_PERSONAL_ACCESS_TOKEN ? | string | Personal access token for authenticating with SettleMint services | sdk/utils/src/validation/dot-env.schema.ts:18 |
SETTLEMINT_PORTAL ? | string | Unique name of the Smart Contract Portal instance | sdk/utils/src/validation/dot-env.schema.ts:47 |
SETTLEMINT_PORTAL_GRAPHQL_ENDPOINT ? | string | GraphQL endpoint URL for the Portal | sdk/utils/src/validation/dot-env.schema.ts:49 |
SETTLEMINT_PORTAL_REST_ENDPOINT ? | string | REST endpoint URL for the Portal | sdk/utils/src/validation/dot-env.schema.ts:51 |
SETTLEMINT_SMART_CONTRACT_ADDRESS ? | string | Address of the deployed smart contract | sdk/utils/src/validation/dot-env.schema.ts:83 |
SETTLEMINT_SMART_CONTRACT_DEPLOYMENT_ID ? | string | Deployment ID of the smart contract | sdk/utils/src/validation/dot-env.schema.ts:85 |
SETTLEMINT_THEGRAPH ? | string | Unique name of The Graph instance | sdk/utils/src/validation/dot-env.schema.ts:38 |
SETTLEMINT_THEGRAPH_SUBGRAPH_NAME ? | string | Name of The Graph subgraph | sdk/utils/src/validation/dot-env.schema.ts:45 |
SETTLEMINT_THEGRAPH_SUBGRAPHS_ENDPOINTS ? | string [] | Array of endpoint URLs for The Graph subgraphs | sdk/utils/src/validation/dot-env.schema.ts:40 |
SETTLEMINT_WORKSPACE ? | string | Unique name of the workspace | sdk/utils/src/validation/dot-env.schema.ts:20 |
DotEnvPartial:
object
Defined in: sdk/utils/src/validation/dot-env.schema.ts:102
Type definition for the partial environment variables schema.
Name | Type | Description | Defined in |
---|---|---|---|
SETTLEMINT_ACCESS_TOKEN ? | string | Application access token for authenticating with SettleMint services | sdk/utils/src/validation/dot-env.schema.ts:16 |
SETTLEMINT_APPLICATION ? | string | Unique name of the application | sdk/utils/src/validation/dot-env.schema.ts:22 |
SETTLEMINT_BLOCKCHAIN_NETWORK ? | string | Unique name of the blockchain network | sdk/utils/src/validation/dot-env.schema.ts:24 |
SETTLEMINT_BLOCKCHAIN_NODE ? | string | Unique name of the blockchain node | sdk/utils/src/validation/dot-env.schema.ts:26 |
SETTLEMINT_BLOCKSCOUT ? | string | Unique name of the Blockscout instance | sdk/utils/src/validation/dot-env.schema.ts:75 |
SETTLEMINT_BLOCKSCOUT_GRAPHQL_ENDPOINT ? | string | GraphQL endpoint URL for Blockscout | sdk/utils/src/validation/dot-env.schema.ts:77 |
SETTLEMINT_BLOCKSCOUT_UI_ENDPOINT ? | string | UI endpoint URL for Blockscout | sdk/utils/src/validation/dot-env.schema.ts:79 |
SETTLEMINT_CUSTOM_DEPLOYMENT ? | string | Unique name of the custom deployment | sdk/utils/src/validation/dot-env.schema.ts:71 |
SETTLEMINT_CUSTOM_DEPLOYMENT_ENDPOINT ? | string | Endpoint URL for the custom deployment | sdk/utils/src/validation/dot-env.schema.ts:73 |
SETTLEMINT_HASURA ? | string | Unique name of the Hasura instance | sdk/utils/src/validation/dot-env.schema.ts:30 |
SETTLEMINT_HASURA_ADMIN_SECRET ? | string | Admin secret for authenticating with Hasura | sdk/utils/src/validation/dot-env.schema.ts:34 |
SETTLEMINT_HASURA_DATABASE_URL ? | string | Database connection URL for Hasura | sdk/utils/src/validation/dot-env.schema.ts:36 |
SETTLEMINT_HASURA_ENDPOINT ? | string | Endpoint URL for the Hasura GraphQL API | sdk/utils/src/validation/dot-env.schema.ts:32 |
SETTLEMINT_HD_PRIVATE_KEY ? | string | Unique name of the HD private key | sdk/utils/src/validation/dot-env.schema.ts:53 |
SETTLEMINT_INSTANCE ? | string | Base URL of the SettleMint platform instance | sdk/utils/src/validation/dot-env.schema.ts:14 |
SETTLEMINT_IPFS ? | string | Unique name of the IPFS instance | sdk/utils/src/validation/dot-env.schema.ts:63 |
SETTLEMINT_IPFS_API_ENDPOINT ? | string | API endpoint URL for IPFS | sdk/utils/src/validation/dot-env.schema.ts:65 |
SETTLEMINT_IPFS_GATEWAY_ENDPOINT ? | string | Gateway endpoint URL for IPFS | sdk/utils/src/validation/dot-env.schema.ts:69 |
SETTLEMINT_IPFS_PINNING_ENDPOINT ? | string | Pinning service endpoint URL for IPFS | sdk/utils/src/validation/dot-env.schema.ts:67 |
SETTLEMINT_LOAD_BALANCER ? | string | Unique name of the load balancer | sdk/utils/src/validation/dot-env.schema.ts:28 |
SETTLEMINT_MINIO ? | string | Unique name of the MinIO instance | sdk/utils/src/validation/dot-env.schema.ts:55 |
SETTLEMINT_MINIO_ACCESS_KEY ? | string | Access key for MinIO authentication | sdk/utils/src/validation/dot-env.schema.ts:59 |
SETTLEMINT_MINIO_ENDPOINT ? | string | Endpoint URL for MinIO | sdk/utils/src/validation/dot-env.schema.ts:57 |
SETTLEMINT_MINIO_SECRET_KEY ? | string | Secret key for MinIO authentication | sdk/utils/src/validation/dot-env.schema.ts:61 |
SETTLEMINT_NEW_PROJECT_NAME ? | string | Name of the new project being created | sdk/utils/src/validation/dot-env.schema.ts:81 |
SETTLEMINT_PERSONAL_ACCESS_TOKEN ? | string | Personal access token for authenticating with SettleMint services | sdk/utils/src/validation/dot-env.schema.ts:18 |
SETTLEMINT_PORTAL ? | string | Unique name of the Smart Contract Portal instance | sdk/utils/src/validation/dot-env.schema.ts:47 |
SETTLEMINT_PORTAL_GRAPHQL_ENDPOINT ? | string | GraphQL endpoint URL for the Portal | sdk/utils/src/validation/dot-env.schema.ts:49 |
SETTLEMINT_PORTAL_REST_ENDPOINT ? | string | REST endpoint URL for the Portal | sdk/utils/src/validation/dot-env.schema.ts:51 |
SETTLEMINT_SMART_CONTRACT_ADDRESS ? | string | Address of the deployed smart contract | sdk/utils/src/validation/dot-env.schema.ts:83 |
SETTLEMINT_SMART_CONTRACT_DEPLOYMENT_ID ? | string | Deployment ID of the smart contract | sdk/utils/src/validation/dot-env.schema.ts:85 |
SETTLEMINT_THEGRAPH ? | string | Unique name of The Graph instance | sdk/utils/src/validation/dot-env.schema.ts:38 |
SETTLEMINT_THEGRAPH_SUBGRAPH_NAME ? | string | Name of The Graph subgraph | sdk/utils/src/validation/dot-env.schema.ts:45 |
SETTLEMINT_THEGRAPH_SUBGRAPHS_ENDPOINTS ? | string [] | Array of endpoint URLs for The Graph subgraphs | sdk/utils/src/validation/dot-env.schema.ts:40 |
SETTLEMINT_WORKSPACE ? | string | Unique name of the workspace | sdk/utils/src/validation/dot-env.schema.ts:20 |
Id:
string
Defined in: sdk/utils/src/validation/id.schema.ts:30
Type definition for database IDs, inferred from IdSchema. Can be either a PostgreSQL UUID string or MongoDB ObjectID string.
PersonalAccessToken:
string
Defined in: sdk/utils/src/validation/access-token.schema.ts:15
Schema for validating personal access tokens. Personal access tokens start with 'sm_pat_' prefix.
Template:
object
Defined in: sdk/utils/src/package-manager/download-and-extract.ts:8
Available templates for project creation
Name | Type | Defined in |
---|---|---|
label | string | sdk/utils/src/package-manager/download-and-extract.ts:8 |
value | string | sdk/utils/src/package-manager/download-and-extract.ts:8 |
Url:
string
Defined in: sdk/utils/src/validation/url.schema.ts:18
Schema for validating URLs.
import { UrlSchema } from "@settlemint/sdk-utils";
// Validate a URL
const isValidUrl = UrlSchema.safeParse("https://console.settlemint.com").success;
// true
// Invalid URLs will fail validation
const isInvalidUrl = UrlSchema.safeParse("not-a-url").success;
// false
UrlOrPath:
string
Defined in: sdk/utils/src/validation/url.schema.ts:55
Schema that accepts either a full URL or a URL path.
import { UrlOrPathSchema } from "@settlemint/sdk-utils";
// Validate a URL
const isValidUrl = UrlOrPathSchema.safeParse("https://console.settlemint.com").success;
// true
// Validate a path
const isValidPath = UrlOrPathSchema.safeParse("/api/v1/users").success;
// true
UrlPath:
string
Defined in: sdk/utils/src/validation/url.schema.ts:38
Schema for validating URL paths.
import { UrlPathSchema } from "@settlemint/sdk-utils";
// Validate a URL path
const isValidPath = UrlPathSchema.safeParse("/api/v1/users").success;
// true
// Invalid paths will fail validation
const isInvalidPath = UrlPathSchema.safeParse("not-a-path").success;
// false
const
AccessTokenSchema:ZodString
<AccessToken
>
Defined in: sdk/utils/src/validation/access-token.schema.ts:21
Schema for validating both application and personal access tokens. Accepts tokens starting with either 'sm_pat_' or 'sm_aat_' prefix.
const
ApplicationAccessTokenSchema:ZodString
<ApplicationAccessToken
>
Defined in: sdk/utils/src/validation/access-token.schema.ts:7
Schema for validating application access tokens. Application access tokens start with 'sm_aat_' prefix.
const
DotEnvSchema:ZodObject
<DotEnv
>
Defined in: sdk/utils/src/validation/dot-env.schema.ts:12
Schema for validating environment variables used by the SettleMint SDK. Defines validation rules and types for configuration values like URLs, access tokens, workspace names, and service endpoints.
const
DotEnvSchemaPartial:ZodObject
<DotEnvPartial
>
Defined in: sdk/utils/src/validation/dot-env.schema.ts:97
Partial version of the environment variables schema where all fields are optional. Useful for validating incomplete configurations during development or build time.
const
IdSchema:ZodUnion
<Id
>
Defined in: sdk/utils/src/validation/id.schema.ts:17
Schema for validating database IDs. Accepts both PostgreSQL UUIDs and MongoDB ObjectIDs. PostgreSQL UUIDs are 32 hexadecimal characters with hyphens (e.g. 123e4567-e89b-12d3-a456-426614174000). MongoDB ObjectIDs are 24 hexadecimal characters (e.g. 507f1f77bcf86cd799439011).
import { IdSchema } from "@settlemint/sdk-utils";
// Validate PostgreSQL UUID
const isValidUuid = IdSchema.safeParse("123e4567-e89b-12d3-a456-426614174000").success;
// Validate MongoDB ObjectID
const isValidObjectId = IdSchema.safeParse("507f1f77bcf86cd799439011").success;
const
PersonalAccessTokenSchema:ZodString
<PersonalAccessToken
>
Defined in: sdk/utils/src/validation/access-token.schema.ts:14
Schema for validating personal access tokens. Personal access tokens start with 'sm_pat_' prefix.
const
runsInBrowser:boolean
=isBrowser
Defined in: sdk/utils/src/runtime/ensure-server.ts:40
Boolean indicating if code is currently running in a browser environment
const
runsOnServer:boolean
=!isBrowser
Defined in: sdk/utils/src/runtime/ensure-server.ts:45
Boolean indicating if code is currently running in a server environment
const
UniqueNameSchema:ZodString
Defined in: sdk/utils/src/validation/unique-name.schema.ts:19
Schema for validating unique names used across the SettleMint platform. Only accepts lowercase alphanumeric characters and hyphens. Used for workspace names, application names, service names etc.
import { UniqueNameSchema } from "@settlemint/sdk-utils";
// Validate a workspace name
const isValidName = UniqueNameSchema.safeParse("my-workspace-123").success;
// true
// Invalid names will fail validation
const isInvalidName = UniqueNameSchema.safeParse("My Workspace!").success;
// false
const
UrlOrPathSchema:ZodUnion
<UrlOrPath
>
Defined in: sdk/utils/src/validation/url.schema.ts:54
Schema that accepts either a full URL or a URL path.
import { UrlOrPathSchema } from "@settlemint/sdk-utils";
// Validate a URL
const isValidUrl = UrlOrPathSchema.safeParse("https://console.settlemint.com").success;
// true
// Validate a path
const isValidPath = UrlOrPathSchema.safeParse("/api/v1/users").success;
// true
const
UrlPathSchema:ZodString
<UrlPath
>
Defined in: sdk/utils/src/validation/url.schema.ts:34
Schema for validating URL paths.
import { UrlPathSchema } from "@settlemint/sdk-utils";
// Validate a URL path
const isValidPath = UrlPathSchema.safeParse("/api/v1/users").success;
// true
// Invalid paths will fail validation
const isInvalidPath = UrlPathSchema.safeParse("not-a-path").success;
// false
const
UrlSchema:ZodString
<Url
>
Defined in: sdk/utils/src/validation/url.schema.ts:17
Schema for validating URLs.
import { UrlSchema } from "@settlemint/sdk-utils";
// Validate a URL
const isValidUrl = UrlSchema.safeParse("https://console.settlemint.com").success;
// true
// Invalid URLs will fail validation
const isInvalidUrl = UrlSchema.safeParse("not-a-url").success;
// false
We welcome contributions from the community! Please check out our Contributing guide to learn how you can help improve the SettleMint SDK through bug reports, feature requests, documentation updates, or code contributions.
The SettleMint SDK is released under the FSL Software License. See the LICENSE file for more details.
FAQs
Shared utilities and helper functions for SettleMint SDK modules
The npm package @settlemint/sdk-utils receives a total of 16,698 weekly downloads. As such, @settlemint/sdk-utils popularity was classified as popular.
We found that @settlemint/sdk-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.