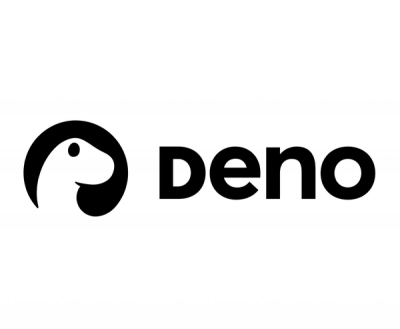
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@shibacore/apis
Advanced tools
A TypeScript library for interacting with the WhatsApp Business API. It provides a simple and flexible interface to send various types of messages (text, templates, media, interactive components, etc.) using WhatsApp's Graph API.
A TypeScript library for interacting with the WhatsApp Business API. It provides a simple and flexible interface to send various types of messages (text, templates, media, interactive components, etc.) using WhatsApp's Graph API.
npm install @shibacore/apis
Prerequisites
import { WhatsappMessages } from "@shibacore/apis";
const accessToken = "your-access-token";
const phoneNumberId = "your-phone-number-id";
const apiVersion = "v20.0";
const whatsapp =
new WhatsappMessages(accessToken, apiVersion, phoneNumberId);
// Send a text message
(async () => {
const response =
await whatsapp.text("recipient-phone-number", "Hello, World!");
console.log(response);
})();
Provides methods to send different types of WhatsApp messages. Constructor
constructor(
access_token: string,
version: Version,
phoneNumberId: PhoneNumberId,
headerOptions?: HeaderOptions,
tokenType?: TokenType
)
async template(
to: string,
templateName: string,
language_code: LanguageCode,
components?: TemplateComponents
): Promise<WhatsappMessageResponse>;
Sends a WhatsApp template message.
Example:
await whatsapp
.template("recipient-phone-number", "hello_world", "en_US");
async text(
to: string,
bodyText: string,
preview_url?: boolean
): Promise<WhatsappMessageResponse>;
Sends a plain text message.
Example:
await whatsapp
.text("recipient-phone-number", "Hello, this is a message!");
Image
async image(
to: string,
imageOption: Media
): Promise<WhatsappMessageResponse>;
Video
async video(
to: string,
videoOption: Media
): Promise<WhatsappMessageResponse>;
Example:
await whatsapp
.image("recipient-phone-number", { id: "media-id" });
Interactive Messages
async interactiveListReply(
to: string,
component: InteractionButtonComponent
): Promise<WhatsappMessageResponse>;
Example:
const response = await whatsapp.interactiveReplyButtons("recipient-phone-number", {
body: {
text: "body text",
},
buttons: [
{
type: "reply",
reply: {
id: "1",
title: "reply 1",
},
},
],
});
async interactiveListReply(
to: string,
component: InteractiveListComponent
): Promise<WhatsappMessageResponse>;
Example:
const response = await whatsapp.interactiveListReply(req.body.to, {
body: {
text: "body text",
},
button: "list",
section: [
{
title: "section 1",
rows: [
{
id: "1",
title: "Option 1",
},
],
},
],
});
Location Messages
async location(
to: string,
location: Location
): Promise<WhatsappMessageResponse>;
Example:
await whatsapp.location("recipient-phone-number", {
latitude: "12.34",
longitude: "56.78",
name: "Location Name",
address: "123 Address St."
});
This project is licensed under the MIT License
. See the LICENSE file for details.
This README.md
ensures clarity and usability for users integrating your library.
FAQs
A TypeScript library for interacting with the WhatsApp Business API. It provides a simple and flexible interface to send various types of messages (text, templates, media, interactive components, etc.) using WhatsApp's Graph API.
The npm package @shibacore/apis receives a total of 0 weekly downloads. As such, @shibacore/apis popularity was classified as not popular.
We found that @shibacore/apis demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.