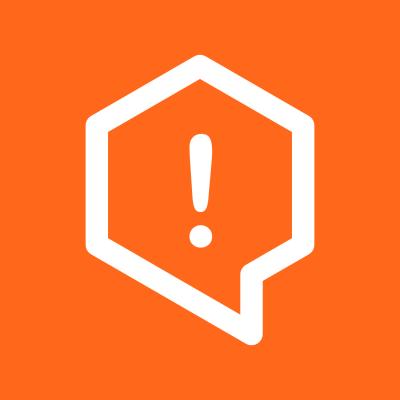
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@shopify/react-performance
Advanced tools
Primitives to measure your React application's performance using @shopify/performance
@shopify/react-performance
Primitives to measure your React application's performance using @shopify/performance
$ yarn add @shopify/react-performance
The most basic way to use the tools in this package is to record information and display it locally to the user. In practice you usually only want to do this in development so that developers can easily see performance information.
Before we can use any of the components or hooks in the package we must wrap our app tree with the PerformanceContext.Provider
component.
// App.tsx
import React from 'react';
import {Performance, PerformanceContext} from '@shopify/react-performance';
// in a Server-Side-Rendering enabled app you will likely only want to instantiate this if `document` is defined.
const performance = new Performance();
function App() {
return (
<PerformanceContext.Provider value={performance}>
{/* The rest of your app */}
</PerformanceContext.Provider>
);
}
Now that we have access to PerformanceContext
we can use the other components and hooks offered by @shopify/react-performance
anywhere in our tree. To demonstrate, we'll create a component called LastNavigationDetails
and use it to display some basic data about our app's performance.
// LastNavigationDetails.tsx
import React, {useState} from 'react';
import {useNavigationListener, Navigation} from '@shopify/react-performance';
export function LastNavigationDetails() {
const [lastNavigation, setLastNavigation] = useState<Navigation | null>(null);
useNavigationListener(navigation => {
setLastNavigation(navigation);
});
if (lastNavigation == null) {
return <p>no data</p>;
}
const {duration, isFullPageNavigation, target};
const navigationType = isFullPageNavigation
? 'full page navigation'
: 'single-page-app style navigation';
return (
<p>
The last navigation was to {target}. It was a {navigationType} navigation
which took {duration / 1000} seconds to complete.
</p>
);
}
We can render this component anywhere in our tree, but lets do so in our App component from earlier.
// App.tsx
import React from 'react';
import {Performance, PerformanceContext} from '@shopify/react-performance';
import {LastNavigationDetails} from './LastNavigationDetails';
// in a Server-Side-Rendering enabled app you will likely only want to instantiate this if `document` is defined.
const performance = new Performance();
function App() {
return (
<PerformanceContext.Provider value={performance}>
{/* The rest of your app */}
<LastNavigationDetails />
</PerformanceContext.Provider>
);
}
Now our developers will have access to up-to-date information about how long initial page-loads and incremental navigations take to complete without needing to open their devtools or dig into DOM APIs manually.
Performance metrics data is most useful when it can be aggregated and used to make smart optimization decisions or warn you when your app is beginning to become slow. To aggregate such data you'll need to be able to send it to a server. @shopify/react-performance
provides the PerformanceReport
component to facilitate this.
This section is a work in progress. It will be filled out as helper libraries for marshalling data on the server are added to Quilt.
Most APIs provided by this package are available as both hooks (ie. useNavigationListener
), as well as components (ie. <NavigationListener />
).
This package also re-exports all of the API of @shopify/performance
.
A custom hook which takes in callback to invoke whenever the Performance
context object emits a lifecycleEvent.
import {useLifecycleEventListener} from '@shopify/react-performance';
function SomeComponent() {
useLifecycleEventListener(event => {
console.log(event);
});
return <div>something</div>;
}
A custom hook which takes in a callback to invoke whenever a navigation takes place, whether it is a full-page load (such as the result of location.assign
) or an incrmental load (such as the result of router.push
in a ReactRouter app).
import {useNavigationListener} from '@shopify/react-performance';
function SomeComponent() {
useNavigationListener(navigation => {
console.log(navigation);
});
return <div>something</div>;
}
A custom hook which takes an id
and stage
and uses them to generate a tag for a call to window.performance.mark
when the component is mounted. This can be used to mark specialized moments in your applications startup, or a specific interaction.
import React from 'react';
import {usePerformanceMark} from '@shopify/react-performance';
function SomeComponent() {
usePerformanceMark('some-stage', 'some-id');
return <div>something</div>;
}
If the hook is called with a stage
of usable
or complete
, it also calls the appropriate method on the Performance
object from context, allowing you to trigger the finish
and usable
lifecycleEvents based on a particular component mounting.
import React from 'react';
import {usePerformanceMark} from '@shopify/react-performance';
function ProductPage() {
// this will call `performance.finish()` on the Performance object in context
usePerformanceMark('complete', 'products');
return <div>cool product page</div>;
}
Todo
A component which takes in an onEvent
callback as a prop. This callback is invoked whenever the Performance
context object emits a lifecycleEvent.
import React from 'react';
import {LifecycleEventListener} from '@shopify/react-performance';
function SomeComponent() {
return (
<>
<LifecycleEventListener onEvent={event => console.log(event)} />
<div>something</div>
</>
);
}
A component which takes in an onNavigation
callback as a prop. This callback is invoked whenever the Performance
context reports a navigation.
import React from 'react';
import {NavigationListener} from '@shopify/react-performance';
function SomeComponent() {
return (
<>
<NavigationListener
onNavigation={navigation => console.log(navigation)}
/>
<div>something</div>
</>
);
}
A componenr which takes both id
and stage
props and uses them to generate a tag for a call to window.performance.mark
when it is mounted. This can be used to mark specialized moments in your applications startup, or a specific interaction.
import React from 'react';
import {PerformanceMark} from '@shopify/react-performance';
function SomeComponent() {
return (
<>
<PerformanceMark stage="some-stage" id="some-id" />
<div>something</div>
</>
);
}
If the hook is called with a stage
of usable
or complete
, it also calls the appropriate method on the Performance
object from context, allowing you to trigger the finish
and usable
lifecycleEvents based on a particular component mounting.
import React from 'react';
import {PerformanceMark} from '@shopify/react-performance';
function ProductPage() {
import React from 'react';
import {PerformanceMark} from '@shopify/react-performance';
function ProductPage() {
return (
<>
<PerformanceMark stage="usable" id="ProductPage" />
<div>Some cool product page</div>
</>
);
}
}
A vanilla React.createContext
object to provide a Performance
class through your application's tree.
Todo
FAQs
Primitives to measure your React application's performance using `@shopify/performance`
The npm package @shopify/react-performance receives a total of 15,313 weekly downloads. As such, @shopify/react-performance popularity was classified as popular.
We found that @shopify/react-performance demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 24 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.