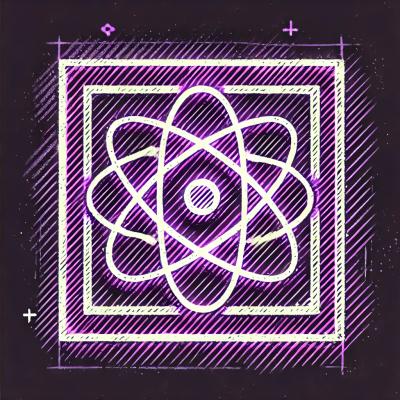
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@simbathesailor/use-what-changed
Advanced tools
A simple hook to debug various Reactjs hooks which supports dependency as the second argument.
If you use yarn. Run
yarn add @simbathesailor/use-what-changed
If you use npm. Run
npm i @simbathesailor/use-what-changed --save
I have been working on hooks for quite a long time. I use react hooks every day in my open source projects and also at work.
Now, using useEffect, useCallback, useMemo have really helped me compose the logic well together. But when the dependency list gets long. When I say long , it can be any thing greater than 3 for me and can be more or less for others.
With these large dependency array, I found it really difficult to debug and find out what is causing my useEffect to run again( same for useCallback and useMemo). I know two strategies to debug:
Break the useEffect logic into multiple useEffect. It is still fine, but expertise and time constraints will be there. People will not break the useEffect logic into smaller pieces first, they will try to spend time using logging the values and adding debugger so that not to change the production code.
Make use of usePrevious hook which can be defined something like this
import React from 'react';
function usePrevious(value) {
const ref = React.useRef(value);
React.useEffect(() => {
ref.current = value;
});
return ref.current;
}
export default usePrevious;
And can be consumed like this:
const previousA = usePrevious(a);
const previousB = usePrevious(b);
const previousC = usePrevious(c);
useEffect(() => {
if (previousA !== a) {
console.log(`a has changed from ${previousA} to ${a}`);
}
if (previousB !== b) {
console.log(`a has changed from ${previousB} to ${b}`);
}
if (previousC !== c) {
console.log(`a has changed from ${previousC} to ${c}`);
}
}, [a, b, c]);
However we can do it , it quite too much of work every time you run in the issue , where useEffect callback is running unexpectedly.
To solve the above problem, I tried to create something which can enhance developer experience in this case. Let's see my try for the above problems.
Note: This hook only logs in the development environment. It make use of standard process.env.NODE_ENV to decide. Open devtools console tab to see the logs.
import { useWhatChanged } from '@simbathesailor/use-what-changed';
function App() {
const [a, setA] = React.useState(0);
const [b, setB] = React.useState(0);
const [c, setC] = React.useState(0);
const [d, setD] = React.useState(0);
// Just place the useWhatChanged hook call with dependency before your
// useEffect, useCallback or useMemo
useWhatChanged([a, b, c, d]); // debugs the below useEffect
React.useEffect(() => {
// console.log("some thing changed , need to figure out")
}, [a, b, c, d]);
return <div className="container">Your app jsx</div>;
}
Above snapshot show the console log when b and c has changed in the above code example.
useWhatChanged([a, b, c, d], 'a, b, c, d'); // debugs the below useEffect
A unique background color will be given to each title text. It helps us in recognising the specific effect when debugging. A unique id is also given to help the debugging further.
Please read CONTRIBUTING.md for details on our code of conduct, and the process for submitting pull requests to us.
We use SemVer for versioning. For the versions available, see the tags on this repository.
See also the list of contributors who participated in this project.
This project is licensed under the MIT License - see the LICENSE.md file for details
Thanks goes to these wonderful people (emoji key):
Anil kumar Chaudhary 💻 🤔 🎨 📖 🐛 |
FAQs
A simple hook to debug various Reactjs hooks which supports dependency as the second argument.
The npm package @simbathesailor/use-what-changed receives a total of 54,882 weekly downloads. As such, @simbathesailor/use-what-changed popularity was classified as popular.
We found that @simbathesailor/use-what-changed demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.